-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
0 parents
commit 309799b
Showing
96 changed files
with
8,943 additions
and
0 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,8 @@ | ||
node_modules/ | ||
dist/ | ||
.idea/workspace.xml | ||
license.json | ||
yfiles-license.json | ||
*.tsbuildinfo | ||
.tsup/ | ||
package-lock.json |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,24 @@ | ||
This source code can be used as part of a yFiles for HTML application. | ||
https://www.yworks.com/yfileshtml | ||
Copyright (c) 2000-2024 by yWorks GmbH, Vor dem Kreuzberg 28, | ||
72070 Tübingen, Germany. All rights reserved. | ||
|
||
Any redistribution of the files in this repository in source code or other form, | ||
with or without modification, is not permitted. | ||
The creation of derivative works is prohibited, unless otherwise explicitly permitted. | ||
|
||
Owners of a valid software license for a yFiles for HTML version | ||
are allowed to use these sources as basis for their own yFiles for HTML powered applications. | ||
Use of such programs is governed by the rights and conditions as set out in the | ||
yFiles for HTML license agreement. | ||
|
||
THIS SOFTWARE IS PROVIDED ''AS IS'' AND ANY EXPRESS OR IMPLIED | ||
WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED WARRANTIES OF | ||
MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. IN | ||
NO EVENT SHALL yWorks BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, | ||
SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT LIMITED | ||
TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR | ||
PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF | ||
LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING | ||
NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS | ||
SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,169 @@ | ||
# yFiles React Supply Chain Component | ||
|
||
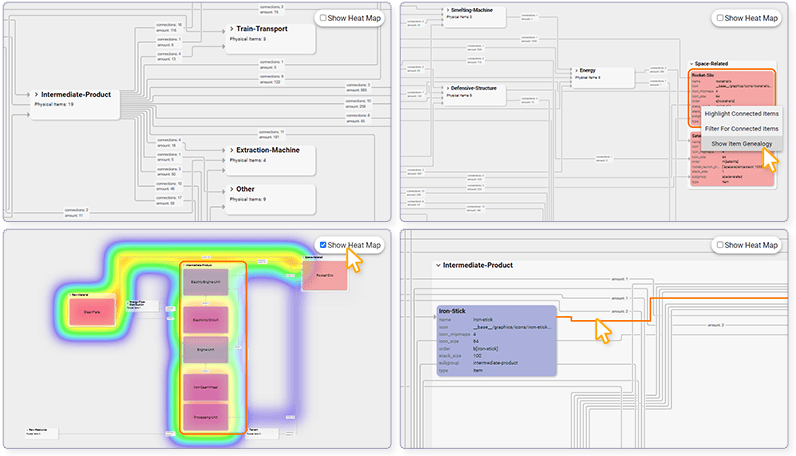 | ||
|
||
Traditionally associated with manufacturing processes, supply chain management is equally important for the provision | ||
of any good or service, whether physical, face-to-face, or purely digital. Managing a supply chain requires visualizing | ||
and analyzing all of the components, resources and process in a network. Comprehensively diagramming a supply network | ||
enables teams to identify inefficiencies and optimize the overall supply chain. | ||
|
||
Our powerful and versatile React component based on the [yFiles](https://www.yworks.com/yfiles-overview) library, allows | ||
you to seamlessly incorporate dynamic and interactive supply chain diagrams into your applications. This enhances the | ||
user experience and facilitates an intuitive exploration of complex manufacturing processes. | ||
|
||
## Getting Started | ||
|
||
1. **Installation:** | ||
Install the component via npm by running the following command in your project directory: | ||
```bash | ||
npm install @yworks/react-yfiles-supply-chain | ||
``` | ||
|
||
The supply chain module has certain peer dependencies that must be installed within your project. Since it is a React | ||
module, `react` and `react-dom` dependencies are needed. | ||
|
||
Additionally, the component relies on the [yFiles](https://www.yworks.com/yfiles-overview) library which is not | ||
available on the public npm registry. Instructions on how to work with the yFiles npm module in | ||
our [Developer's Guide](https://docs.yworks.com/yfileshtml/#/dguide/yfiles_npm_module). | ||
|
||
Ensure that the dependencies in the `package.json` file resemble the following: | ||
```json | ||
{ | ||
... | ||
"dependencies": { | ||
"@yworks/react-yfiles-supply-chain": "^1.0.0", | ||
"react": "^18.2.0", | ||
"react-dom": "^18.2.0", | ||
"yfiles": "<yFiles package path>/lib/yfiles-26.0.0.tgz", | ||
... | ||
} | ||
} | ||
``` | ||
|
||
2. **License:** | ||
Before using the component, a valid [yFiles for HTML](https://www.yworks.com/products/yfiles-for-html) version is | ||
required. You can evaluate yFiles for 60 days free of charge | ||
on [my.yworks.com](https://my.yworks.com/signup?product=YFILES_HTML_EVAL). | ||
Be sure to invoke the `registerLicense` function to furnish the license file before utilizing the supply chain | ||
component. | ||
|
||
3. **Usage:** | ||
Utilize the component in your application. | ||
Make sure to import the CSS stylesheet 'index.css' as the component requires it for correct functionality. | ||
|
||
```tsx | ||
import { | ||
registerLicense, | ||
SupplyChain, | ||
SupplyChainData, | ||
UserSupplyChainItem, | ||
UserSupplyChainConnection | ||
} from '@yworks/react-yfiles-supply-chain' | ||
import '@yworks/react-yfiles-supply-chain/dist/index.css' | ||
import yFilesLicense from './license.json' | ||
|
||
function App() { | ||
registerLicense(yFilesLicense) | ||
|
||
const data = { | ||
items: [ | ||
{ name: 'Copper-Ore', id: 1, parentId: 3 }, | ||
{ name: 'Copper-Plate', id: 2, parentId: 4 }, | ||
{ name: 'Resource', id: 3 }, | ||
{ name: 'Material', id: 4 } | ||
], | ||
connections: [{ sourceId: 1, targetId: 2 }] | ||
} satisfies SupplyChainData<UserSupplyChainItem, UserSupplyChainConnection> | ||
|
||
return <SupplyChain data={data}></SupplyChain> | ||
} | ||
|
||
export default App | ||
``` | ||
|
||
> **Note:** By default, the `SupplyChain` component adjusts its size to match the size of its parent element. | ||
Therefore, it is necessary to set the dimensions of the containing element or apply styling directly to | ||
the `SupplyChain` component. This can be achieved by defining a CSS class or applying inline styles. | ||
|
||
## Documentation | ||
|
||
Find the full documentation, API and many code examples in our [documentation](https://docs.yworks.com/react-yfiles-supply-chain). | ||
|
||
## Live Playground | ||
|
||
[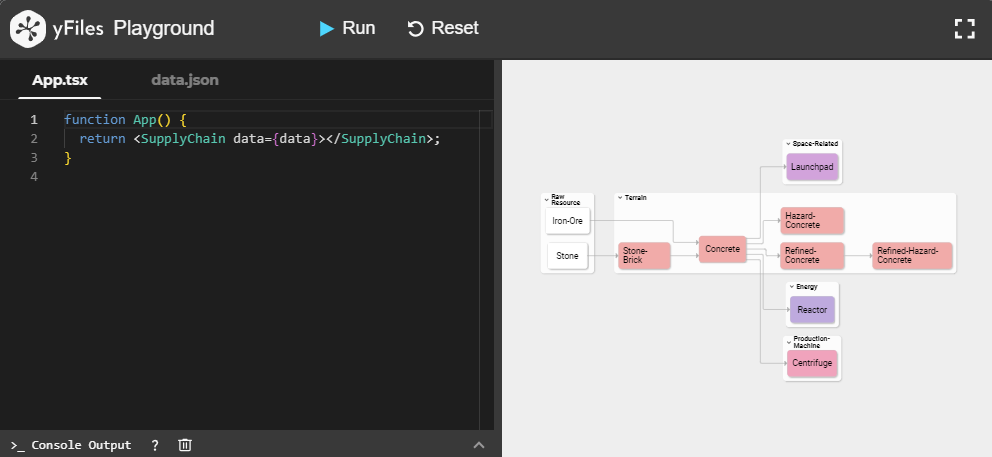](https://docs.yworks.com/react-yfiles-supply-chain/introduction/welcome) | ||
|
||
Try the yFiles React Supply Chain component directly in your browser with our [playground](https://docs.yworks.com/react-yfiles-supply-chain/introduction/welcome) | ||
|
||
## Features | ||
|
||
The supply chain component provides versatile features that can be adjusted to specific use cases or used with the | ||
provided default features. | ||
|
||
### Custom node visualization | ||
|
||
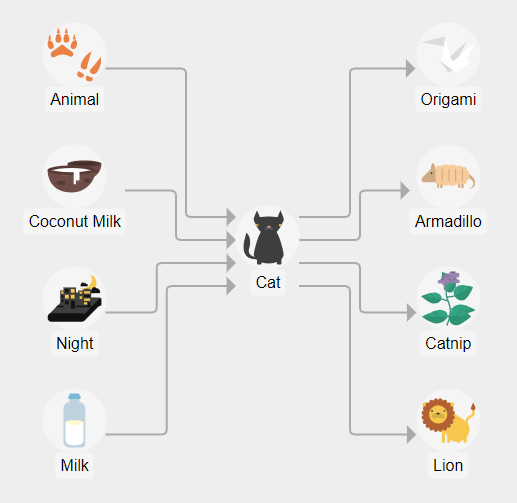 | ||
|
||
The `renderItem` and `renderGroup` property allow providing custom React components for the node visualization. Try the | ||
API in the [playground](https://docs.yworks.com/react-yfiles-supply-chain/features/custom-items). | ||
|
||
### Custom connection visualization | ||
|
||
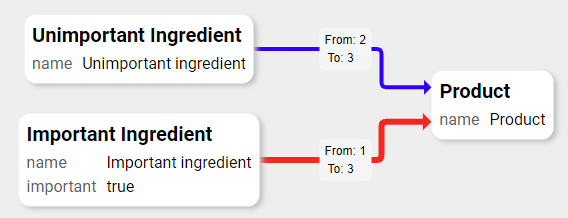 | ||
|
||
With the `connectionStyleProvider`, the connection visualization may be adjusted to the use-case. Additionally, the | ||
`connectionLabelProvider` may be used to display related information directly on the connection. Try the API in | ||
the [playground](https://docs.yworks.com/react-yfiles-supply-chain/features/custom-connections). | ||
|
||
### Grouping and folding | ||
|
||
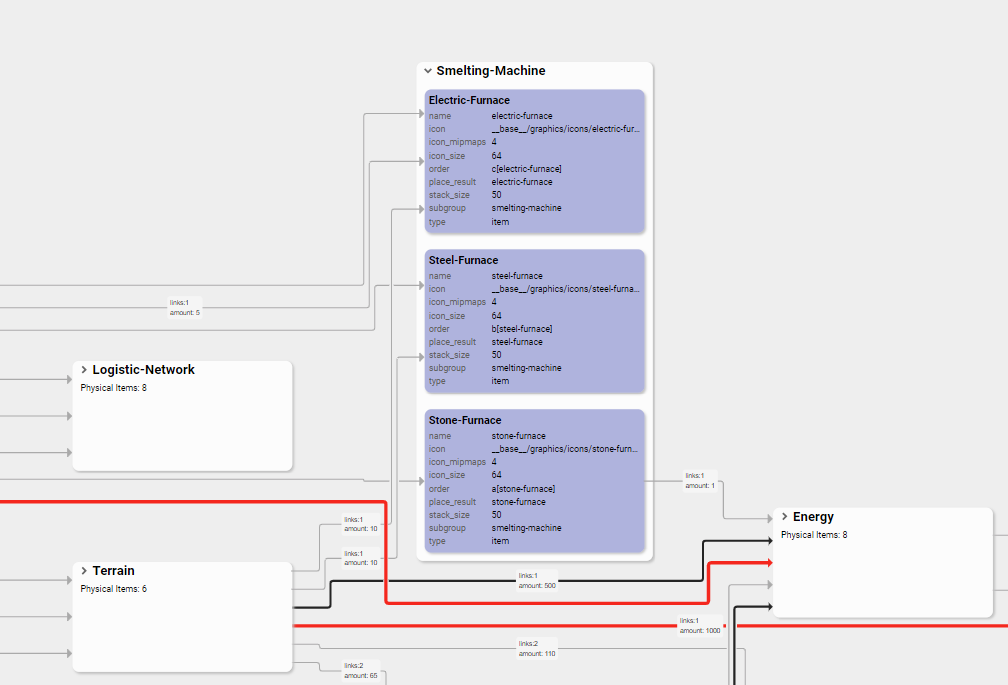 | ||
|
||
The component automatically creates nested nodes if the item contains a `parentId` property. The nested nodes can be | ||
collapsed/expanded interactively. Try the API in | ||
the [playground](https://docs.yworks.com/react-yfiles-supply-chain/features/hook-supplychainprovider). | ||
|
||
### Heat mapping | ||
|
||
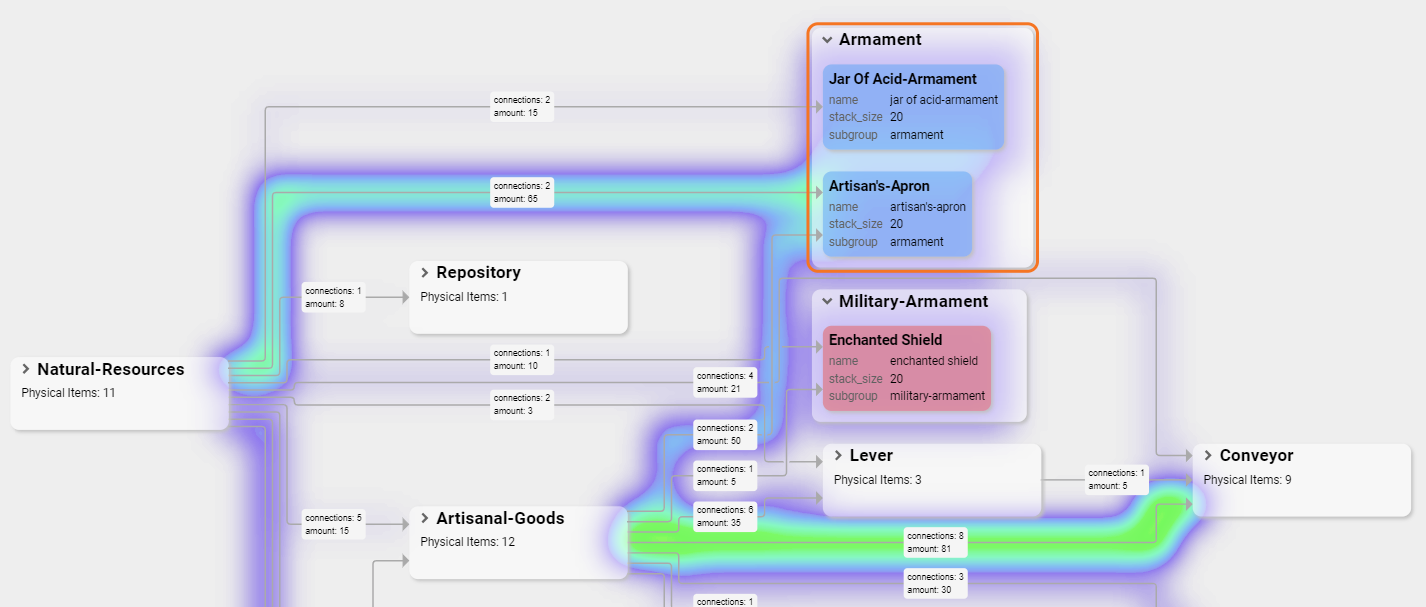 | ||
|
||
The `heatMapping` function allows to provide a "heat" value for items and connections that visualizes an additional | ||
information layer in the component. | ||
|
||
### Graph search | ||
|
||
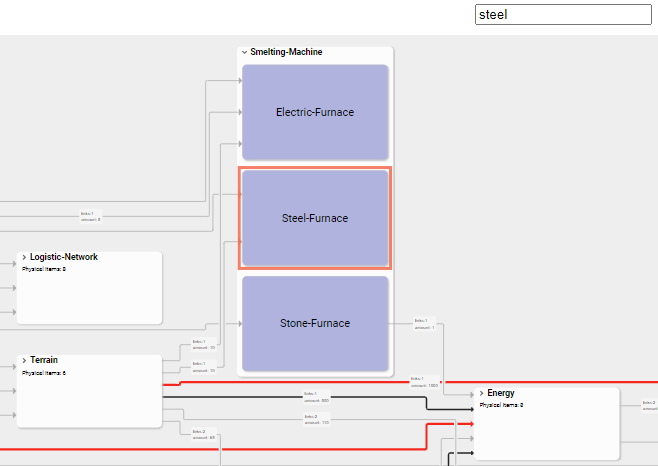 | ||
|
||
The versatile graph search helps to find items in larger supply chains. Try it in | ||
the [playground](https://docs.yworks.com/react-yfiles-supply-chain/features/search). | ||
|
||
### Custom tooltips | ||
|
||
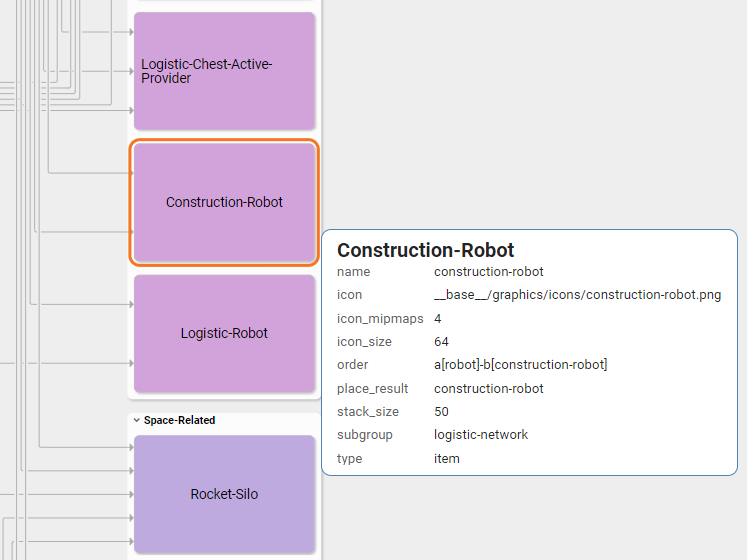 | ||
|
||
The `renderTooltip` property can be used to provide use-case specific React components as tooltips. Try the API in | ||
the [playground](https://docs.yworks.com/react-yfiles-supply-chain/features/tooltips). | ||
|
||
### Graph overview | ||
|
||
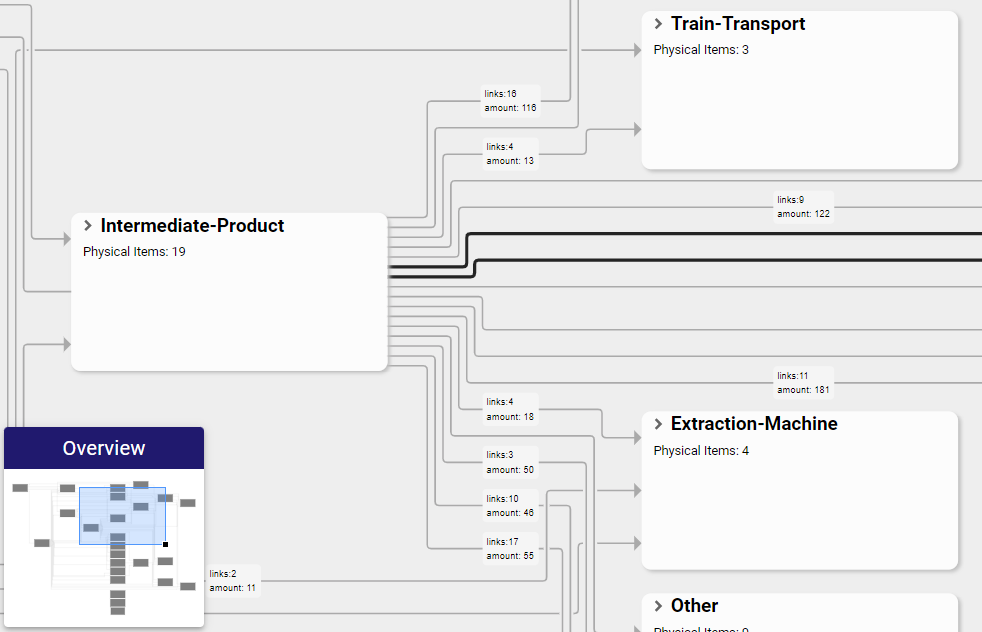 | ||
|
||
The graph overview provides a quick and easy way to navigate larger graph structures. Try it in | ||
the [playground](https://docs.yworks.com/react-yfiles-supply-chain/features/built-in-components). | ||
|
||
### Context menu | ||
|
||
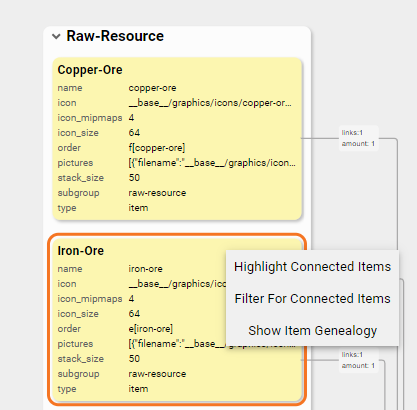 | ||
|
||
The context menu can be populated with custom components and actions. Try it in | ||
the [playground](https://docs.yworks.com/react-yfiles-supply-chain/features/context-menu). | ||
|
||
## Learn More | ||
|
||
Explore the possibilities of visualizing supply chains and other diagrams with yFiles - the powerful and versatile | ||
diagramming SDK. For | ||
further information about [yFiles for HTML](https://www.yworks.com/yfiles-overview) and our company, please | ||
visit [yWorks.com](https://www.yworks.com). | ||
|
||
For support or feedback, please reach out to [our support team](https://www.yworks.com/contact) or open | ||
an [issue on GitHub](https://github.com/yWorks/react-yfiles-supply-chain/issues). Happy diagramming! |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,36 @@ | ||
{ | ||
"$schema": "https://developer.microsoft.com/json-schemas/api-extractor/v7/api-extractor.schema.json", | ||
"mainEntryPointFilePath": "./dist/index.d.ts", | ||
"bundledPackages": [ | ||
"@yworks/react-yfiles-core" | ||
], | ||
"apiReport": { | ||
"enabled": false | ||
}, | ||
"docModel": { | ||
"enabled": false | ||
}, | ||
"dtsRollup": { | ||
"enabled": true, | ||
"untrimmedFilePath": "./dist/index.d.ts" | ||
}, | ||
"tsdocMetadata": { | ||
"enabled": false | ||
}, | ||
"messages": { | ||
"compilerMessageReporting": { | ||
"default": { | ||
"logLevel": "warning" | ||
} | ||
}, | ||
"extractorMessageReporting": { | ||
"default": { | ||
"logLevel": "warning" | ||
}, | ||
"ae-missing-release-tag": { | ||
"logLevel": "none", | ||
"addToApiReportFile": false | ||
} | ||
} | ||
} | ||
} |
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
Loading
Sorry, something went wrong. Reload?
Sorry, we cannot display this file.
Sorry, this file is invalid so it cannot be displayed.
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,3 @@ | ||
{ | ||
"mainComponents": ["SupplyChain", "SupplyChainProvider"] | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,19 @@ | ||
export const SITE = { | ||
title: 'yFiles React Supply Chain Component', | ||
description: '', | ||
defaultLanguage: 'en_US', | ||
twitter: '@yworks', | ||
github: 'yWorks/react-yfiles-supply-chain', | ||
linkedin: 'yworks-gmbh', | ||
name: 'react-yfiles-supply-chain' | ||
} | ||
|
||
export const OPEN_GRAPH = { | ||
image: { | ||
src: '', | ||
alt: '' | ||
}, | ||
twitter: '' | ||
} | ||
|
||
// TODO twitter card |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
--- | ||
title: Built-In Components | ||
section: 9 | ||
tags: [] | ||
showRightSideBar: false | ||
--- | ||
import Playground from '../../components/Playground-Supply-Chain.astro' | ||
import TypeLink from '../../components/TypeLink.astro' | ||
|
||
# Built-In Components | ||
|
||
There are optional components available to enhance the interaction with the supply chain, which can be added as children of the <TypeLink type="SupplyChain" /> component: | ||
<ul className="list-disc ml-4"> | ||
<li>**<TypeLink type="Overview" />**: Presents a simplified view of the entire graph along with the current viewport.</li> | ||
<li>**<TypeLink type="Controls" />**: Features a toolbar with buttons to adjust the viewport.</li> | ||
</ul> | ||
|
||
The controls component comes with a default implementation for supply chains. | ||
|
||
|
||
<Playground | ||
code={` | ||
function App () { | ||
return ( | ||
<SupplyChain data={data}> | ||
<Overview></Overview> | ||
<Controls buttons={SupplyChainControlButtons}></Controls> | ||
</SupplyChain> | ||
) | ||
} | ||
`} | ||
data={`{ | ||
"items": [ | ||
{ "name": "Copper-Ore", "id": 1, "parentId": 3 }, | ||
{ "name": "Copper-Plate", "id": 2, "parentId": 4 }, | ||
{ "name": "Resource", "id": 3 }, | ||
{ "name": "Material", "id": 4 } | ||
], | ||
"connections": [{ "sourceId": 1, "targetId": 2 }] | ||
}`} | ||
/> |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,48 @@ | ||
--- | ||
title: Connection Labels | ||
section: 3 | ||
tags: [] | ||
showRightSideBar: false | ||
--- | ||
import Playground from '../../components/Playground-Supply-Chain.astro' | ||
import TypeLink from '../../components/TypeLink.astro' | ||
|
||
# Connection Labels | ||
With the <TypeLink type="SupplyChain" member="connectionLabelProvider"/> specific data can be visualized for each | ||
connection. | ||
|
||
The provider is called for each <TypeLink type="SupplyChainConnection"/> (or <TypeLink type="FoldingConnection" /> if | ||
either source or target is collapsed) and should return a <TypeLink type="SimpleConnectionLabel" /> if a label should | ||
be shown on the connection. If the connection should not show a label, `undefined` may be returned. | ||
|
||
<Playground | ||
code={` | ||
function connectionLabelProvider( | ||
item: SupplyChainConnection | FoldingConnection, | ||
{ isFoldingConnection }: SupplyChainModel | ||
): SimpleConnectionLabel | undefined { | ||
if (!isFoldingConnection(item)) { | ||
return { | ||
text: \`From: \${item.sourceId}\\n To: \${item.targetId}\`, | ||
labelShape: 'round-rectangle', | ||
className: 'default-connection-label' | ||
} satisfies SimpleConnectionLabel | ||
} | ||
} | ||
function App() { | ||
return <SupplyChain data={data} connectionLabelProvider={connectionLabelProvider}></SupplyChain> | ||
} | ||
`} | ||
data={`{ | ||
"items": [ | ||
{ "name": "Important ingredient", "id": 1 }, | ||
{ "name": "Unimportant ingredient", "id": 2 }, | ||
{ "name": "Product", "id": 3 } | ||
], | ||
"connections": [ | ||
{ "sourceId": 1, "targetId": 3 }, | ||
{ "sourceId": 2, "targetId": 3 } | ||
] | ||
}`} | ||
/> |
Oops, something went wrong.