-
-
Notifications
You must be signed in to change notification settings - Fork 1.3k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
fix: Cache
StoreUser
(JSIStoreValueUser
) on a per-`RuntimeManager…
…`-basis (#2219) ## Description <!-- Description and motivation for this PR. Inlude Fixes #<number> if this is fixing some issue. Fixes # . --> Until now, the `JSIStoreValueUser` class was used to cache `jsi::Value`s. This worked fine for Reanimated since there was only one separate Runtime apart from the React-JS runtime, but once another library tries to use the workletization code, this will break since `JSIStoreValueUser` is accessed from multiple runtimes. Instead, it should only be used on a per-runtime basis, so I moved the static code to instance code, and added a Shared Pointer to `RuntimeManager`. This fixes SIGABRT crashes for when trying to use a captured variable (such as `console`) in multiple runtimes (REA, VisionCamera, react-native-multithreading, ...): 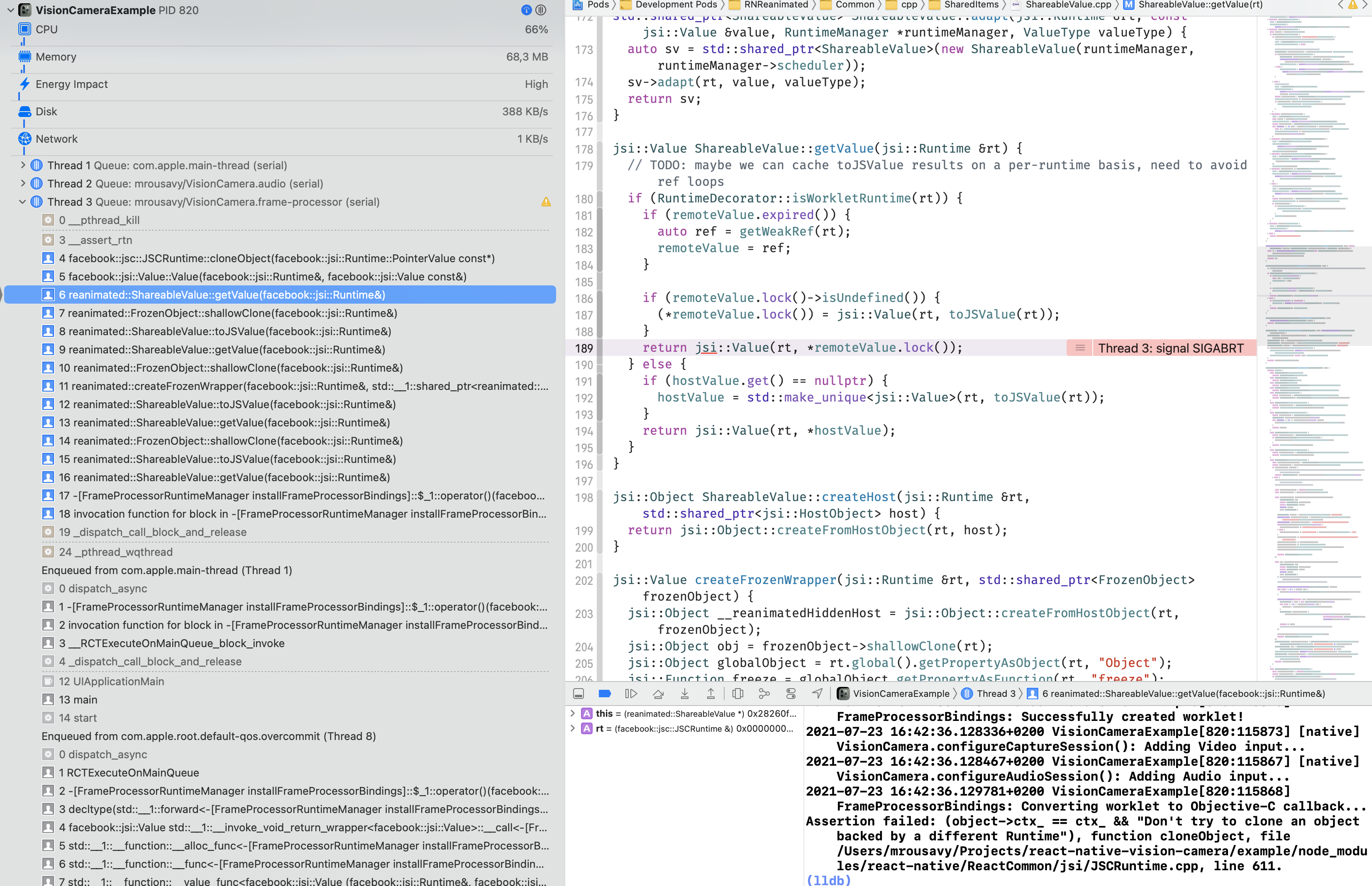 The above crash happened because a `jsi::Value` (`console`) cached inside `StoreUser` was accessed from the VisionCamera runtime, but the value was initially created on the REA runtime. ## Changes <!-- Please describe things you've changed here, make a **high level** overview, if change is simple you can omit this section. For example: - Added `foo` method which add bouncing animation - Updated `about.md` docs - Added caching in CI builds --> * Refactored `JSIStoreValueUser` to be an instance variable of `RuntimeManager` instead of a static one. * Clear store on `RuntimeManager` dealloc (dtor) * Fix a `isWorkletRuntime` check which should instead strictly compare if the runtimes are exactly same <!-- ## Screenshots / GIFs Here you can add screenshots / GIFs documenting your change. You can add before / after section if you're changing some behavior. ### Before ### After --> ## Test code and steps to reproduce 1. Create a normal function on the React-JS Thread 2. Verify that you can call that function inside of a REA UI Worklet (`useAnimatedStyle`, or `runOnUI`) using `runOnJS` 3. Verify that you can call that same function inside of a VisionCamera FP Worklet (`useFrameProcessor`) using `runOnJS` <!-- Please include code that can be used to test this change and short description how this example should work. This snippet should be as minimal as possible and ready to be pasted into editor (don't exclude exports or remove "not important" parts of reproduction example) --> ## Checklist - [ ] Included code example that can be used to test this change - [ ] Updated TS types - [ ] Added TS types tests - [ ] Added unit / integration tests - [ ] Updated documentation - [ ] Ensured that CI passes
- Loading branch information
Showing
10 changed files
with
51 additions
and
40 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters