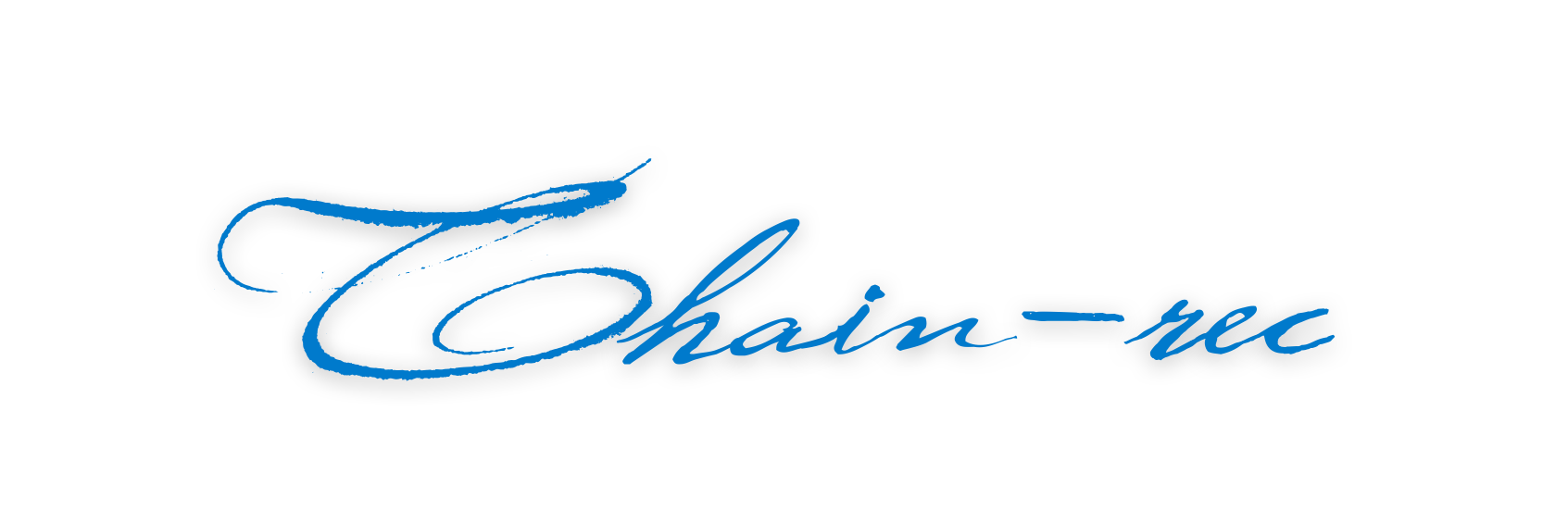
Chain-rec instances and utilities for fp-ts
ChainRec
is a typeclass that models tail-recursion in higher kinded types. However, fp-ts
only exports instances for Either
, Array
, ReadonlyArray
, IO
, Tuple
, and ReadonlyTuple
. ChainRec can be particularly useful for effectful types such as Task, Reader, and State which is the purpose of this library, and to export various utilities from purescript-tailrec.
This example constructs a program in the StateIO
monad and will print the numbers 1 through 10 to the console.
This example uses the following imports:
import * as ChnRec from '@jacob-alford/chain-rec/ChainRec'
import { ChainRec } from '@jacob-alford/chain-rec/StateIO'
import * as Cons from 'fp-ts/Console'
import { pipe } from 'fp-ts/function'
import * as O from 'fp-ts/Option'
import * as SIO from 'fp-ts-contrib/StateIO'
SIO.get<number>()
- The program takesnumber
as inputSIO.chainFirst(n => SIO.fromIO(Cons.log(n)))
- Prints the current number to the consoleSIO.map(O.fromPredicate(n => n >= 10))
- Returnsnone
if the number is less than 10SIO.chainFirst(() => SIO.modify(n => n + 1))
- Increments the number by 1
const program = pipe(
SIO.get<number>(),
SIO.chainFirst(n => SIO.fromIO(Cons.log(n))),
SIO.map(O.fromPredicate(n => n >= 10)),
SIO.chainFirst(() => SIO.modify(n => n + 1)),
)
const runProgram = ChnRec.untilSome(ChainRec)(program)
runProgram(0)()
// prints: 0, 1, 2, 3, 4, 5, 6, 7, 8, 9, 10
yarn add @jacob-alford/chain-rec
npm install @jacob-alford/chain-rec
pnpm add @jacob-alford/chain-rec