Closed
Description
I have a schema that works under normal mongoose CRUD. This schema and session_friend.js work with Fawn when I use it under a simple Express project setup. But when I am moving this code to a larger API it seems to be falling down. I assume it is related to where the require happens but here is a simple version of what I am doing.
Structure Snippet:
server.js - Main node js file.
'------- /config/config.js
'------- /custom_modules/util.js - Main anchor for include objects (mongoose, fawn, twilio, etc...)
'------- /api/** - Custom api routes:
'------- /api/session/model/Session.js - Session Schema - Contains fawn tasks
'------- /api/session/session.js - Route code.
util.js
NOTE: Promise is a global for BlueBird promises.
...
var mongoose = require('mongoose');
var uri = Config.get("SESSION.mongodburi");
mongoose.Promise = Promise;
mongoose.connect(uri);
var Fawn = require('fawn');
Fawn.init(mongoose, "fawn_task_cache", {promiseLibrary: Promise});
...
module.exports = {
...
mongoose: mongoose,
fawn: Fawn
}
/api/session/session_friend.js
var build = require('../presence/build_functions');
util.mongoose.Promise = Promise;
// Model Objects
var UserPresence = require('../presence/model/UserPresence');
var GroupSession = require('./model/GroupSession');
// ROUTE '/session/friend/:friendId'
module.exports = {
post: function (req, res) {
var userPrincipal = build.getUserPrincipal(req.headers['x-access-token']);
console.log("*** UserPrincipal " + userPrincipal.userId);
console.log("*** req.params.friendId : " + req.params.friendId);
if (userPrincipal.userId && req.params.friendId) {
UserPresence.findById(req.params.friendId).then(function (friendPresence) {
if (friendPresence && friendPresence.sessionId) {
console.log("**** We Got Presence! ****");
var task = util.fawn.Task();
console.log("*** Lets use some tasks time...");
// Add the session to the user and add the user to the session. Do it in a "transaction".
task.update("UserPresence", {_id: userPrincipal.userId}, {sessionId: friendPresence.sessionId})
.update("GroupSession", {_id: friendPresence.sessionId}, {$push: {userList: userPrincipal.userId}})
.run({useMongoose: true})
.then(function () {
GroupSession.findById(friendPresence.sessionId)
.then(function (session) {
session.userList = [];
res.send(session);
});
}).catch(function (err) {
res.send(err);
});
} else if (!friendPresence.sessionId) {
console.log("**** We Not Gottem! ****");
var groupSession = new GroupSession();
groupSession.userList = [req.params.friendId, userPrincipal.userId];
groupSession.hosted = false;
console.log("**** Group Session: " + JSON.stringify(groupSession));
var task = util.fawn.Task();
// Create the session, add both users get updated.
task.save(GroupSession, groupSession)
.update("UserPresence", {_id: userPrincipal.userId}, {sessionId: {$ojFuture: "0._id"}})
.update("UserPresence", {_id: req.params.friendId}, {sessionId: {$ojFuture: "0._id"}})
.run({useMongoose: true})
.then(function (results) {
console.log("**** After Save Group Session: " + JSON.stringify(results[0]));
// Update of users sessions complete.
var gsess = results[0];
console.log("Saved Group Session " + gsess.userList);
gsess.userlist = [];
res.send(gsess);
})
.catch(function (err) {
console.log("****** " + err);
res.send(err);
});
}
});
}
}
}
When I get to this code all three steps are created in the fawn cache table but then the api hangs because nothing is returned. On the console I get the following warning.
(node:2996) DeprecationWarning: Mongoose: mpromise (mongoose's default promise library) is deprecated, plug in your own promise library instead: http://mongoosejs.com/docs/promises.html```
and the state of step 1 is set to "1", the others are still "0". I do not get any error. It just appears to exit the process abruptly.```
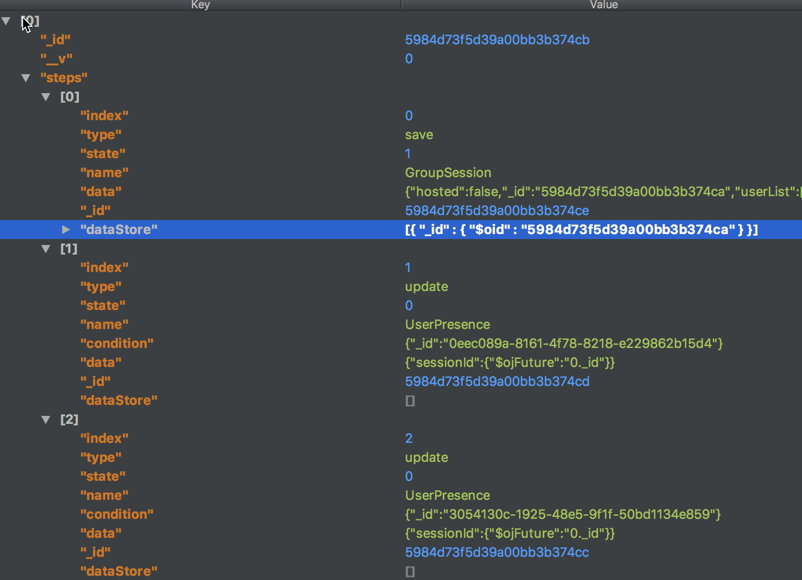