forked from react-boilerplate/react-boilerplate
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
[RFC] Hooks-based saga and reducer injectors (react-boilerplate#2583)
## React Boilerplate The saga and reducer injectors are normally used via HOCs. The HOCs will still work with Hooks-based components but IMO, we can offer a nice alternative which doesn't pollute the render tree: `useInjectSaga` and `useInjectReducer` hooks! You can see this alternative in this PR's diff [here](https://github.com/react-boilerplate/react-boilerplate/pull/2583/files?w=1) (with the no whitespace option enabled). We probably shouldn't remove the HOCs as the Hooks-based injectors won't work with Class components. I do have one question about the InjectSaga HOC: It passes props to `injectSaga`. What's the purpose of this and is there any way around it? This is more of an RFC than anything else. Looking for thoughts and feedback before completing the branch. Cheers! PS: `react-helmet@5` + `useEffect` in the same component = 🧨💥🔥 so I upgraded us to the 6.0.0 beta. 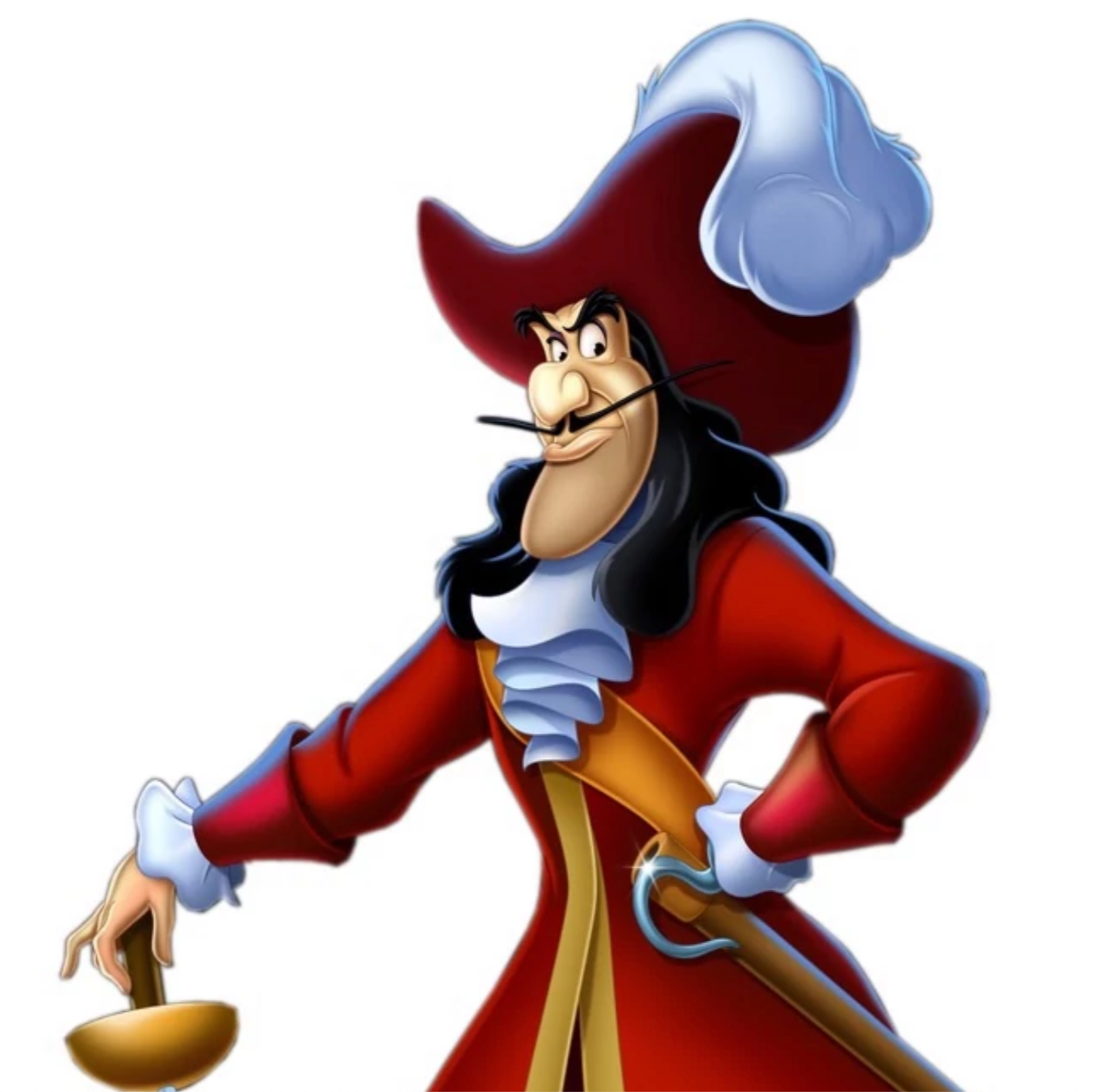
- Loading branch information
Showing
16 changed files
with
280 additions
and
291 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.