-
Notifications
You must be signed in to change notification settings - Fork 336
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
Showing
7 changed files
with
118 additions
and
41 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,41 @@ | ||
# Debounce Operator | ||
|
||
## Overview | ||
|
||
Only emit an item from an Observable if a particular timespan has passed without it emitting another item. | ||
|
||
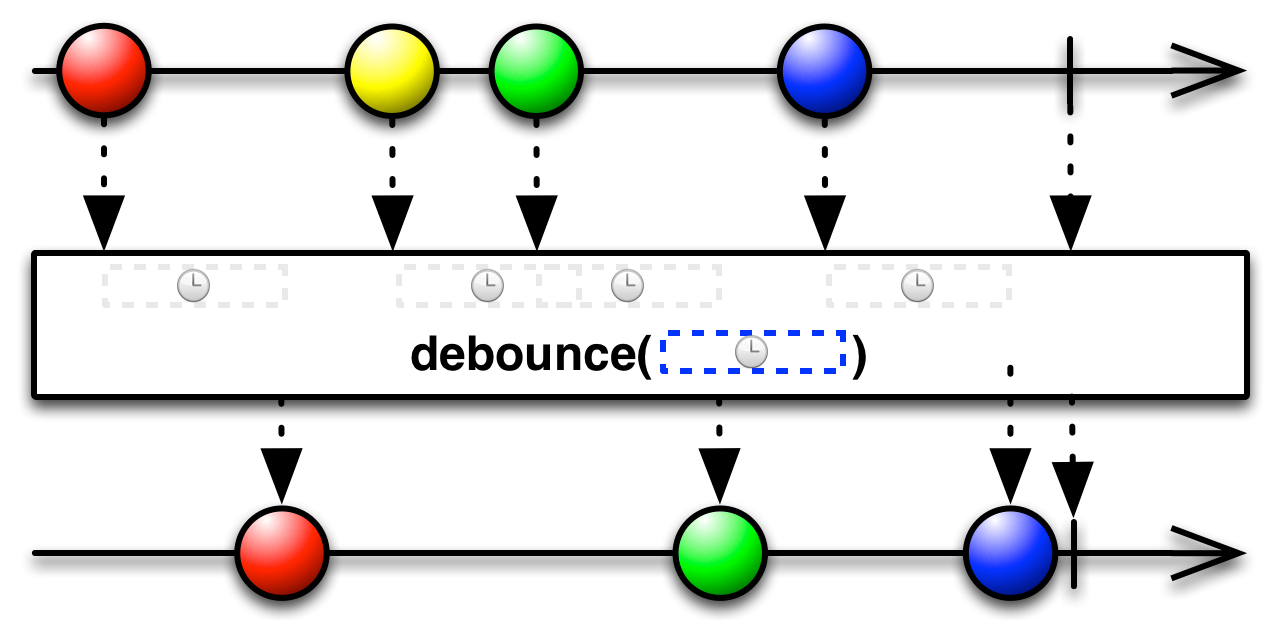 | ||
|
||
## Example | ||
|
||
```go | ||
observable.Debounce(rxgo.WithDuration(250 * time.Millisecond)) | ||
``` | ||
|
||
Output: each item emitted by the Observable if not item has been emitted after 250 milliseconds. | ||
|
||
## Options | ||
|
||
### WithBufferedChannel | ||
|
||
[Detail](options.md#withbufferedchannel) | ||
|
||
### WithContext | ||
|
||
[Detail](options.md#withcontext) | ||
|
||
### WithObservationStrategy | ||
|
||
[Detail](options.md#withobservationstrategy) | ||
|
||
### WithErrorStrategy | ||
|
||
[Detail](options.md#witherrorstrategy) | ||
|
||
### WithPool | ||
|
||
https://github.com/ReactiveX/RxGo/wiki/Options#withpool | ||
|
||
### WithCPUPool | ||
|
||
https://github.com/ReactiveX/RxGo/wiki/Options#withcpupool |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters