## Description
- Update the schema of `SuiTransactionResponse` to make all fields
optional and add a new `digest` field
- Add `SuiTransactionResponseOptions`
- Update the `sui_getTransaction` and `sui_multiGetTransaction` to take
in `SuiTransactionResponseOptions`
- rewrite the `sui_multiGetTransaction` logic
Here's the new interface
```
pub struct SuiTransactionResponseOptions {
/// Whether to show transaction input data. Default to be False
pub show_input: bool,
/// Whether to show transaction effects. Default to be False
pub show_effects: bool,
/// Whether to show transaction events. Default to be False
pub show_events: bool,
/// Whether to show checkpoint sequence number. Default to be False
pub show_checkpoint: bool,
/// Whether to show timestamp. Default to be False
pub show_timestamp: bool,
}
#[derive(Serialize, Deserialize, Debug, JsonSchema, Clone, Default)]
#[serde(rename_all = "camelCase", rename = "TransactionResponse")]
pub struct SuiTransactionResponse {
pub digest: TransactionDigest,
/// Transaction input data
#[serde(skip_serializing_if = "Option::is_none")]
pub transaction: Option<SuiTransaction>,
#[serde(skip_serializing_if = "Option::is_none")]
pub effects: Option<SuiTransactionEffects>,
#[serde(skip_serializing_if = "Option::is_none")]
pub events: Option<SuiTransactionEvents>,
#[serde(default, skip_serializing_if = "Option::is_none")]
pub timestamp_ms: Option<u64>,
#[serde(default, skip_serializing_if = "Option::is_none")]
@@ -49,6 +114,17 @@ pub struct SuiTransactionResponse {
/// This is only returned in the read api, not in the transaction execution api.
#[serde(skip_serializing_if = "Option::is_none")]
pub checkpoint: Option<CheckpointSequenceNumber>,
#[serde(skip_serializing_if = "Vec::is_empty", default)]
pub errors: Vec<String>,
}
#[method(name = "getTransaction")]
async fn get_transaction_with_options(
&self,
/// the digest of the queried transaction
digest: TransactionDigest,
/// options for specifying the content to be returned
options: Option<SuiTransactionResponseOptions>,
) -> RpcResult<SuiTransactionResponse>;
/// Return the object information for a specified object
@@ -139,10 +142,16 @@ pub trait ReadApi {
descending_order: Option<bool>,
) -> RpcResult<TransactionsPage>;
/// Returns an ordered list of transaction responses
/// The method will throw an error if the input contains any duplicate or
/// the input size exceeds [QUERY_MAX_RESULT_LIMIT]
#[method(name = "multiGetTransactions")]
async fn multi_get_transactions_with_options(
&self,
/// A list of transaction digests.
digests: Vec<TransactionDigest>,
/// config options to control which fields to fetch
options: Option<SuiTransactionResponseOptions>,
) -> RpcResult<Vec<SuiTransactionResponse>>;
```
# Follow up items ( in separate PRs)
- [ ] rename `sui_getTransactions` to `sui_queryTransactions(query:
TransactionQuery, options: Option<SuiTransactionResponseOptions>) ->
Page<SuiTransactionResponse>`
- [ ] Add `SuiTransactionResponseOptions` as a parameter for
`sui_executeTransaction`
- [ ] remove existing ``sui_submitTransaction` and
`sui_executeTransactionSig`
- [ ] support options in the Indexer implementation
- [ ] Support more option variants such as `showRawBcs` and
`showLatestObjectData`
## Test Plan
1. TS-SDK e2e tests `pnpm sdk prepare:e2e` `pnpm sdk test:e2e`
2. Test Explorer with the following steps
a. In root sui directory, run `pnpm sdk prepare:e2e`
b. In root sui directory, run `pnpm explorer dev`
c. in root sui directory, run `pnpm sdk test:e2e` to generate some data
d. open localhost:3000 to check on a few transactions with different
kinds
Address page
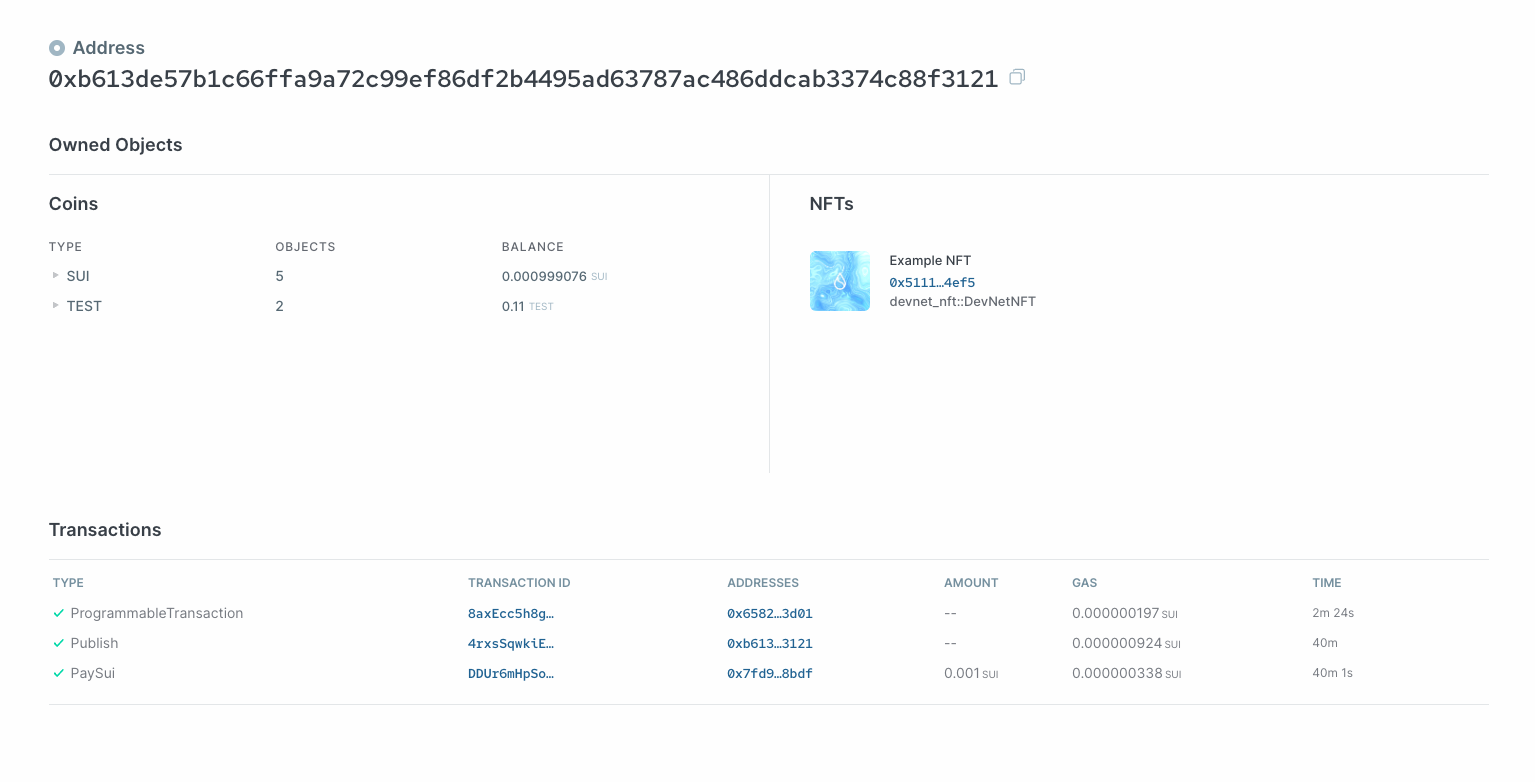
Transaction Details page(Events tab)
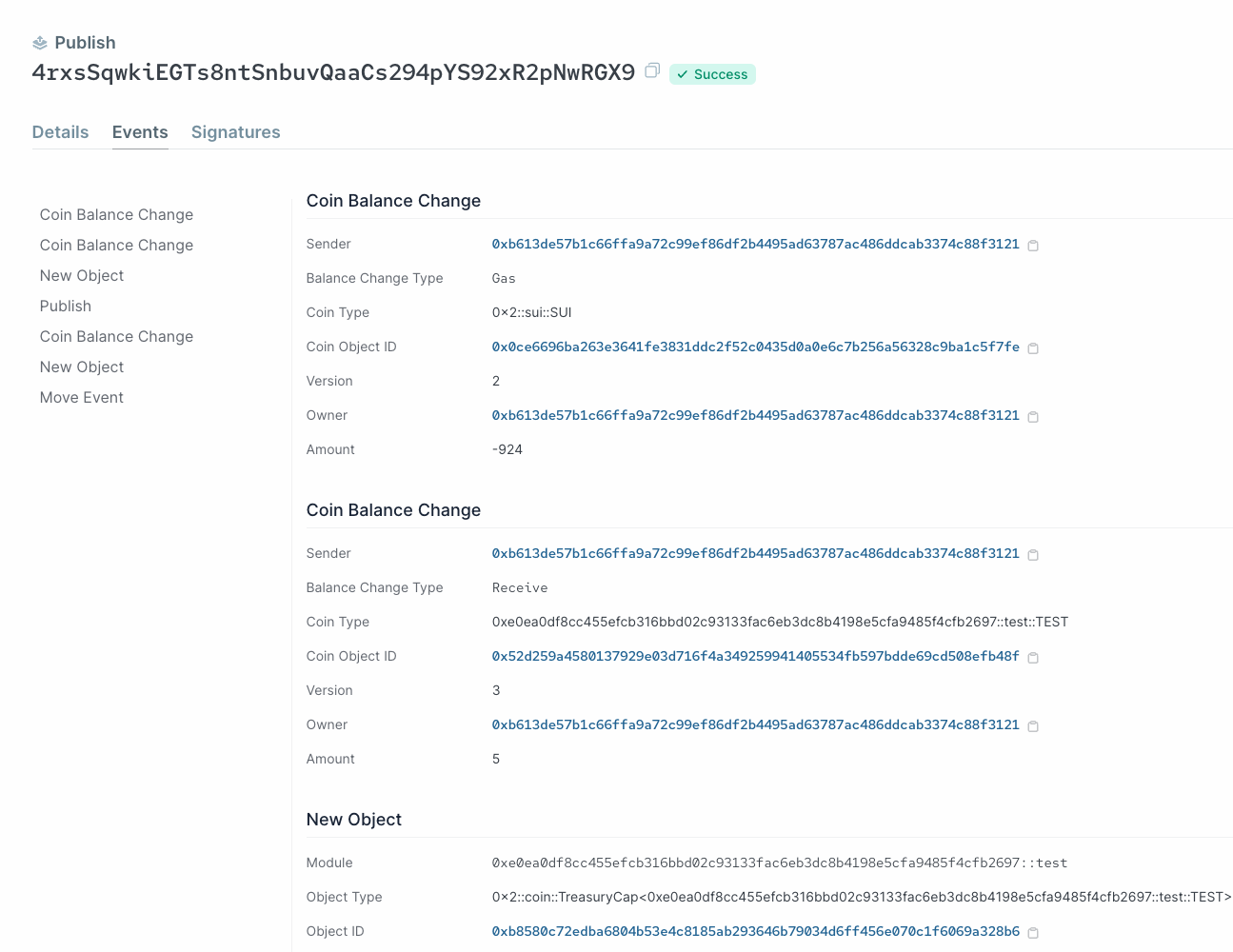
Transaction Details page (main)
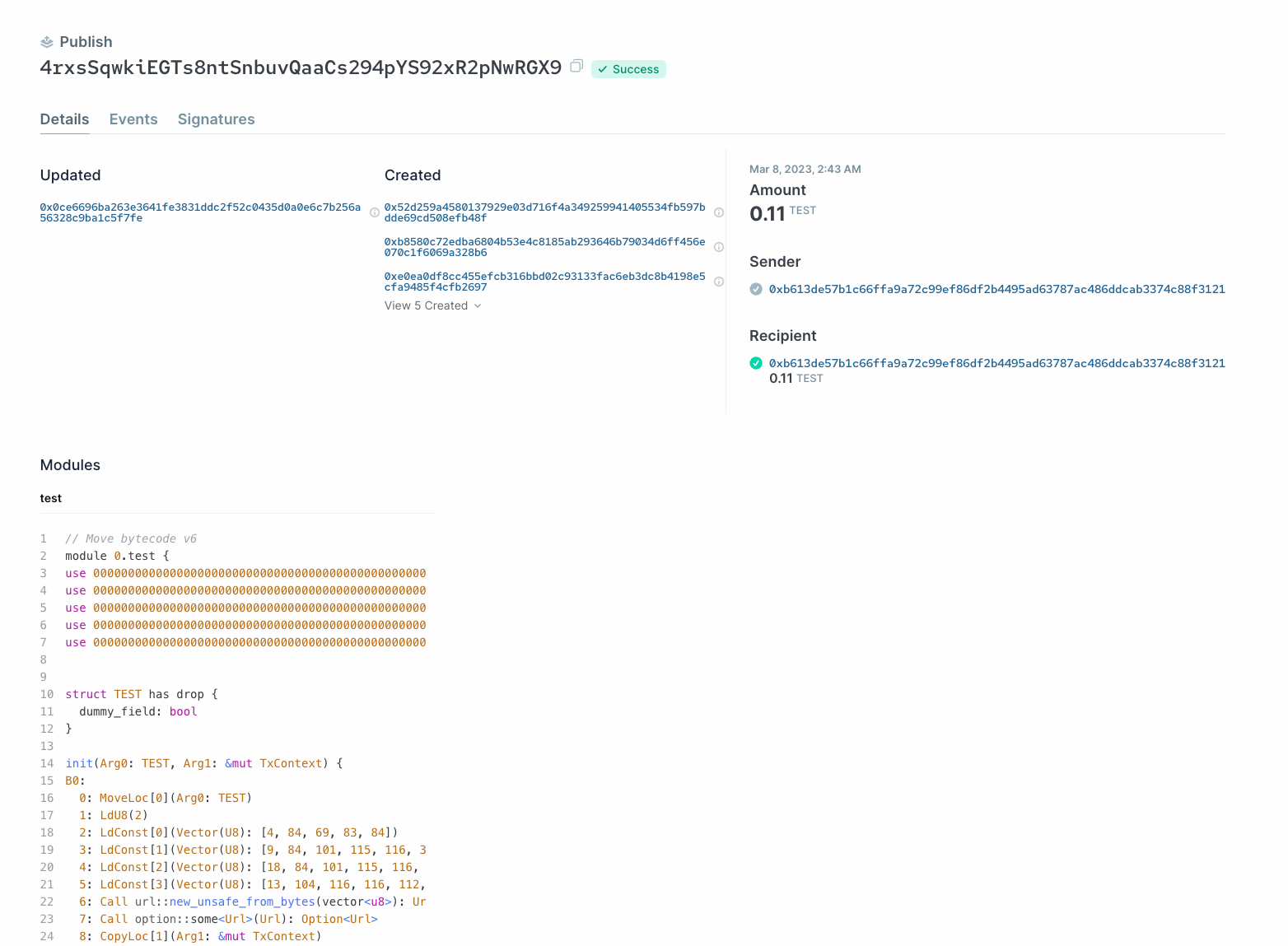
Home page
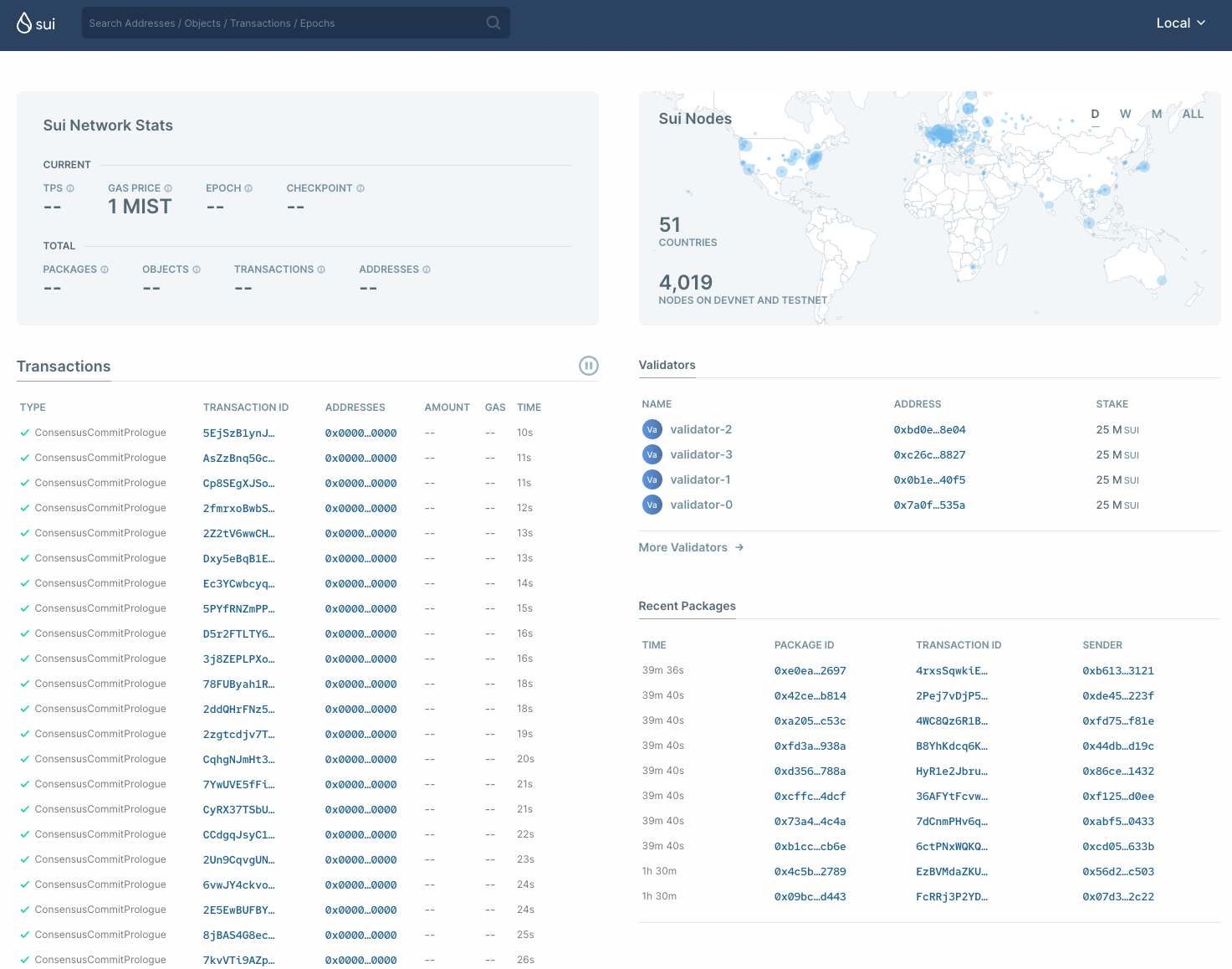
3. Test wallet with the following steps
a. In root sui directory, run `pnpm sdk prepare:e2e`
b. Duplicate this
[file](https://github.com/MystenLabs/sui/blob/main/apps/wallet/configs/environment/.env.defaults#L4)
and rename it to .env,
c. In root sui directory, run `pnpm wallet start`
d. follow
https://github.com/MystenLabs/sui/tree/main/apps/wallet#install-the-extension-to-chrome
to install the wallet
e. make some transactions(staking, transfer, nft mint)
f. verify that the activity tab looks good
Send NFT
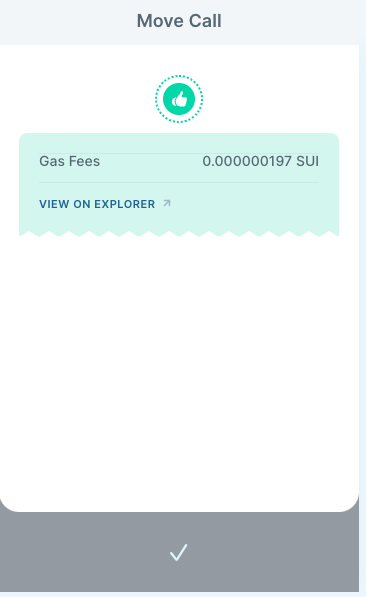
Activity Tab
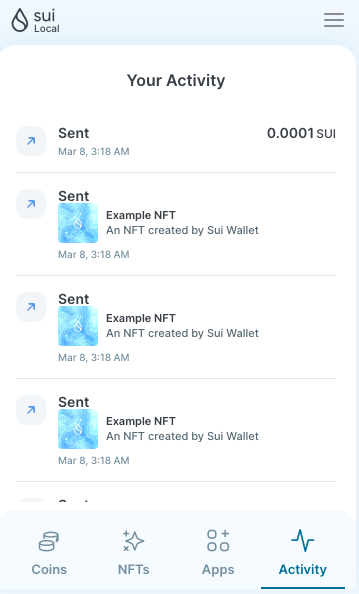
Transfer Coin
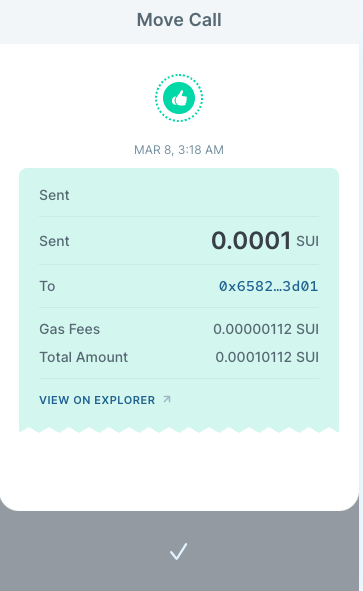
---
If your changes are not user-facing and not a breaking change, you can
skip the following section. Otherwise, please indicate what changed, and
then add to the Release Notes section as highlighted during the release
process.
### Type of Change (Check all that apply)
- [x] user-visible impact
- [x] breaking change for a client SDKs
- [x] breaking change for FNs (FN binary must upgrade)
- [ ] breaking change for validators or node operators (must upgrade
binaries)
- [ ] breaking change for on-chain data layout
- [ ] necessitate either a data wipe or data migration
### Release notes
- [RPC] `sui_getTransaction` and `sui_multiGetTransaction` now take in
an additional optional parameter called `options` which is used to
specify which fields to fetch (e.g., transaction, effects, events, etc).
By default, only the transaction digest will be returned.
- [TS SDK] Rename `provider.getTransactionWithEffects` to
`provider.getTransactionResponse`. The new method takes in an additional
parameter `SuiTransactionResponseOptions` to configure which fields to
fetch (e.g., transaction, effects, events, etc). By default, only the
transaction digest will be returned.