-
Notifications
You must be signed in to change notification settings - Fork 494
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
Merge branch 'Lakhankumawat:main' into dequeue
- Loading branch information
Showing
13 changed files
with
820 additions
and
2 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,73 @@ | ||
//-------To find Connencted component in an undirected graph-------// | ||
|
||
#include<iostream> | ||
#include<list> | ||
|
||
using namespace std ; | ||
|
||
class Graph{//Graph class to create Undirected graph | ||
int n; //number of vertex in the graph | ||
list<int>* adj; //array pointer of adjacency list | ||
|
||
void dfs(int n,bool checked[]); //applying dfs | ||
|
||
public : | ||
Graph(int n); | ||
~Graph(); | ||
void addEdge(int v, int w); // to add an edge in graph | ||
void conenctedComponents(); // print connected components of graph | ||
}; | ||
|
||
void Graph::conenctedComponents(){ // print connected components of graph | ||
bool* checked = new bool[n]; // initialized all vertices as unchecked | ||
for (int v = 0; v < n; v++){ | ||
checked[v] =false; | ||
} | ||
for (int v = 0; v < n; v++){ | ||
if(checked[v]==false) { //if not checked than apply dfs | ||
dfs(v,checked); // calling dfs function | ||
cout<<endl; | ||
} | ||
} | ||
delete[] checked; | ||
} | ||
|
||
void Graph::dfs(int v,bool checked[]){ | ||
checked[v] = true; | ||
cout<<v<<"\t"; | ||
list<int>::iterator i; //for neighbouring vertex | ||
for ( i = adj[v].begin() ; i !=adj[v].end() ; ++i){ | ||
if (!checked[*i]) // if not checked | ||
dfs(*i,checked); // applying dfs to unchecked neighbouring vertex | ||
} | ||
} | ||
|
||
Graph::Graph(int n){ | ||
this->n=n; | ||
adj = new list<int>[n]; | ||
} | ||
|
||
Graph::~Graph(){ | ||
delete[] adj; | ||
} | ||
|
||
void Graph::addEdge(int v,int w){ | ||
adj[v].push_back(w); | ||
adj[w].push_back(v); | ||
} | ||
|
||
int main(){ | ||
int n,v,w; | ||
cout<<"enter the number of vertex"<<endl; | ||
cin>>n; | ||
Graph g(n); | ||
for (int i = 0; i < (n*(n-1)/2); i++) | ||
{ cout<<"enter edge or -1 -1 to exit : "; | ||
cin>>v>>w; | ||
if(v==-1&&w==-1){break;} | ||
g.addEdge(v,w); | ||
} | ||
cout<<"Connected Componentes are : "<<endl; | ||
g.conenctedComponents(); | ||
return 0; | ||
} |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -1 +1,40 @@ | ||
# Table of content | ||
- [Connected components in undirected graph](#connected-components-in-undirected-graph) | ||
- [Code](ConnectedComponentUndirectedGraph.cpp) | ||
- [Algorithm](#algorithm) | ||
- [Properties](#properties) | ||
- [Advantages](#advantages) | ||
|
||
|
||
## Connected components in undirected graph | ||
- connected components in a undirected graph ia a subgraph in which each pair of node in the subgraph have path between them . | ||
|
||
<!-- image to help better explain the concept --> | ||
|
||
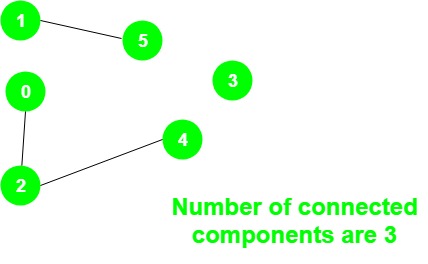 | ||
|
||
### Algorithm | ||
``` | ||
ConnectedComponent(){ | ||
1. initialized all vertices as unchecked | ||
2. for all vertices | ||
- if not checked than apply dfs by calling dfs(v,checked[]) function . | ||
} | ||
dfs(v,checked[]){ | ||
1. mark v as checked and diaplay it | ||
2. for neighbouring vertex | ||
- if not checked apply dfs to unchecked neighbouring vertex | ||
} | ||
``` | ||
|
||
### Properties | ||
|
||
- Time Complexity: O(V+E), where V is the number of vertices and E is total number edges in a graph. | ||
|
||
- Space Complexity: O(n) , where n is total number of vertices in graph . | ||
|
||
### Advantages | ||
- use in the social networking sites, connected components are used to depict the group of people who are friends of each other or who have any common interest | ||
- It can also be used to convert a graph into a Direct Acyclic graph of strongly connected components . |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,64 @@ | ||
|
||
|
||
//------------------------------------PROBLEM : Subsequence with K sum ---------------------------------- | ||
|
||
#include<iostream> | ||
#include<vector> | ||
using namespace std; | ||
|
||
void generate(vector<int> arr, vector<int> output, int index, int sum, int tempSum) | ||
{ | ||
if (index >= arr.size()) | ||
{ | ||
if (tempSum == sum) | ||
{ | ||
//printing all subsequences | ||
for (int i = 0; i < output.size(); i++) | ||
{ | ||
cout << output[i] << " "; | ||
} | ||
cout << endl; | ||
} | ||
return; | ||
} | ||
|
||
output.push_back(arr[index]); | ||
tempSum += arr[index]; | ||
generate(arr, output, index + 1, sum, tempSum); | ||
output.pop_back(); | ||
tempSum -= arr[index]; | ||
generate(arr, output, index + 1, sum, tempSum); | ||
} | ||
|
||
int main() | ||
{ | ||
|
||
vector<int> arr; | ||
int n, sum; | ||
|
||
cout << "Enter the total number of elements in the array/vector : " << endl; | ||
cin >> n; | ||
|
||
cout << "Enter the elements : " << endl; | ||
for (int i = 0; i < n; i++) | ||
{ | ||
int temp; | ||
cin >> temp; | ||
arr.push_back(temp); | ||
} | ||
|
||
cout << "Enter the subsequence sum : " << endl; | ||
cin >> sum; | ||
cout << "The subsequences are as follows : " << endl; | ||
|
||
int tempSum = 0; | ||
vector<int> output; | ||
int index = 0; | ||
|
||
//this prints all the subsequences | ||
generate(arr, output, index, sum, tempSum); | ||
//It is also possible to store all the subsequences in a 2D vector by passing it as a reference in the 'generate' function | ||
return 0; | ||
|
||
} | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,68 @@ | ||
#include <iostream> | ||
using namespace std; | ||
|
||
void cycleSort(int arr[], int n) | ||
{ | ||
//All required variables initilized | ||
int counter = 0,start,elem,pos,temp,i; | ||
|
||
//Array elems are traversed to ensure that they are in correct positions | ||
//elem represents the initial point | ||
for (start = 0; start <= n - 2; start++) { | ||
elem = arr[start]; | ||
|
||
//Smaller array elements to be shifted to the elem varaible's right | ||
//to maintain the sorted order of the final array | ||
pos = start; | ||
for (i = start + 1; i < n; i++) | ||
if (arr[i] < elem) | ||
pos++; | ||
if (pos == start) | ||
continue; | ||
|
||
//Ignore duplicate elements | ||
while (elem == arr[pos]) | ||
pos += 1; | ||
//Element swapped to keep it at right position | ||
if (pos != start) { | ||
temp = elem; | ||
elem = arr[pos]; | ||
arr[pos] = temp; | ||
counter++; | ||
} | ||
while (pos != start) { | ||
pos = start; | ||
for (i = start + 1; i < n; i++) | ||
if (arr[i] < elem) | ||
pos += 1; | ||
while (elem == arr[pos]) | ||
pos += 1; | ||
if (elem != arr[pos]) { | ||
temp = elem; | ||
elem = arr[pos]; | ||
arr[pos] = temp; | ||
counter++; | ||
} | ||
} | ||
} | ||
} | ||
|
||
//Driver method | ||
int main() | ||
{ | ||
//Taking input from the user | ||
cout<<"Enter the no. of elements in array:"<<endl; | ||
int n; | ||
cin>>n; | ||
int arr[n]; | ||
cout<<"Enter the elements in array:"<<endl; | ||
for(int i=0;i<n;i++){ | ||
cin>>arr[i]; | ||
} | ||
cycleSort(arr,n); | ||
cout<<"Sorted array:"<<endl; | ||
for(int i=0;i<n;i++){ | ||
cout << arr[i] << " "; | ||
} | ||
return 0; | ||
} |
Oops, something went wrong.