forked from youngyangyang04/leetcode-master
-
Notifications
You must be signed in to change notification settings - Fork 0
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
- Loading branch information
1 parent
cd4f67d
commit 80dc277
Showing
15 changed files
with
331 additions
and
267 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,97 @@ | ||
|
||
<p align="center"> | ||
<a href="https://mp.weixin.qq.com/s/RsdcQ9umo09R6cfnwXZlrQ"><img src="https://img.shields.io/badge/PDF下载-代码随想录-blueviolet" alt=""></a> | ||
<a href="https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw"><img src="https://img.shields.io/badge/刷题-微信群-green" alt=""></a> | ||
<a href="https://space.bilibili.com/525438321"><img src="https://img.shields.io/badge/B站-代码随想录-orange" alt=""></a> | ||
<a href="https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ"><img src="https://img.shields.io/badge/知识星球-代码随想录-blue" alt=""></a> | ||
</p> | ||
<p align="center"><strong>欢迎大家<a href="https://mp.weixin.qq.com/s/tqCxrMEU-ajQumL1i8im9A">参与本项目</a>,贡献其他语言版本的代码,拥抱开源,让更多学习算法的小伙伴们收益!</strong></p> | ||
|
||
|
||
# 52. N皇后II | ||
|
||
题目链接:https://leetcode-cn.com/problems/n-queens-ii/ | ||
|
||
n 皇后问题研究的是如何将 n 个皇后放置在 n×n 的棋盘上,并且使皇后彼此之间不能相互攻击。 | ||
|
||
上图为 8 皇后问题的一种解法。 | ||
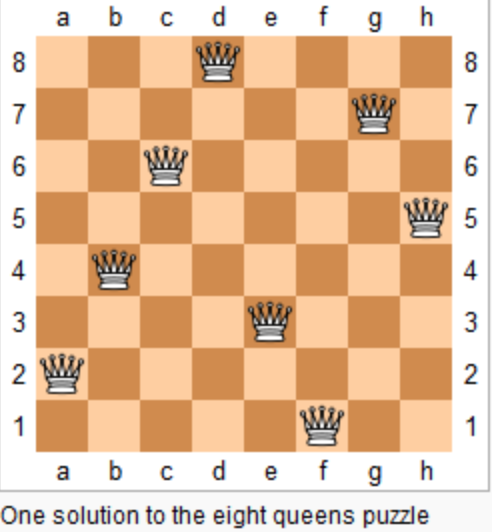 | ||
|
||
给定一个整数 n,返回 n 皇后不同的解决方案的数量。 | ||
|
||
示例: | ||
|
||
输入: 4 | ||
输出: 2 | ||
解释: 4 皇后问题存在如下两个不同的解法。 | ||
[ | ||
[".Q..", // 解法 1 | ||
"...Q", | ||
"Q...", | ||
"..Q."], | ||
|
||
["..Q.", // 解法 2 | ||
"Q...", | ||
"...Q", | ||
".Q.."] | ||
] | ||
|
||
# 思路 | ||
|
||
|
||
想看:[51.N皇后](https://mp.weixin.qq.com/s/lU_QwCMj6g60nh8m98GAWg) ,基本没有区别 | ||
|
||
# C++代码 | ||
|
||
```CPP | ||
class Solution { | ||
private: | ||
int count = 0; | ||
void backtracking(int n, int row, vector<string>& chessboard) { | ||
if (row == n) { | ||
count++; | ||
return; | ||
} | ||
for (int col = 0; col < n; col++) { | ||
if (isValid(row, col, chessboard, n)) { | ||
chessboard[row][col] = 'Q'; // 放置皇后 | ||
backtracking(n, row + 1, chessboard); | ||
chessboard[row][col] = '.'; // 回溯 | ||
} | ||
} | ||
} | ||
bool isValid(int row, int col, vector<string>& chessboard, int n) { | ||
int count = 0; | ||
// 检查列 | ||
for (int i = 0; i < row; i++) { // 这是一个剪枝 | ||
if (chessboard[i][col] == 'Q') { | ||
return false; | ||
} | ||
} | ||
// 检查 45度角是否有皇后 | ||
for (int i = row - 1, j = col - 1; i >=0 && j >= 0; i--, j--) { | ||
if (chessboard[i][j] == 'Q') { | ||
return false; | ||
} | ||
} | ||
// 检查 135度角是否有皇后 | ||
for(int i = row - 1, j = col + 1; i >= 0 && j < n; i--, j++) { | ||
if (chessboard[i][j] == 'Q') { | ||
return false; | ||
} | ||
} | ||
return true; | ||
} | ||
|
||
public: | ||
int totalNQueens(int n) { | ||
std::vector<std::string> chessboard(n, std::string(n, '.')); | ||
backtracking(n, 0, chessboard); | ||
return count; | ||
|
||
} | ||
}; | ||
``` | ||
|
||
# 其他语言补充 | ||
|
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Oops, something went wrong.