diff --git "a/problems/0020.\346\234\211\346\225\210\347\232\204\346\213\254\345\217\267.md" "b/problems/0020.\346\234\211\346\225\210\347\232\204\346\213\254\345\217\267.md"
index 9f6c8487bb..77c6e10a45 100644
--- "a/problems/0020.\346\234\211\346\225\210\347\232\204\346\213\254\345\217\267.md"
+++ "b/problems/0020.\346\234\211\346\225\210\347\232\204\346\213\254\345\217\267.md"
@@ -202,6 +202,23 @@ func isValid(s string) bool {
}
```
+Ruby:
+```ruby
+def is_valid(strs)
+ symbol_map = {')' => '(', '}' => '{', ']' => '['}
+ stack = []
+ strs.size.times {|i|
+ c = strs[i]
+ if symbol_map.has_key?(c)
+ top_e = stack.shift
+ return false if symbol_map[c] != top_e
+ else
+ stack.unshift(c)
+ end
+ }
+ stack.empty?
+end
+```
-----------------------
diff --git "a/problems/0027.\347\247\273\351\231\244\345\205\203\347\264\240.md" "b/problems/0027.\347\247\273\351\231\244\345\205\203\347\264\240.md"
index 959474fc36..9481af1f47 100644
--- "a/problems/0027.\347\247\273\351\231\244\345\205\203\347\264\240.md"
+++ "b/problems/0027.\347\247\273\351\231\244\345\205\203\347\264\240.md"
@@ -123,6 +123,24 @@ public:
Java:
+```java
+class Solution {
+ public int removeElement(int[] nums, int val) {
+
+ // 快慢指针
+ int fastIndex = 0;
+ int slowIndex;
+ for (slowIndex = 0; fastIndex < nums.length; fastIndex++) {
+ if (nums[fastIndex] != val) {
+ nums[slowIndex] = nums[fastIndex];
+ slowIndex++;
+ }
+ }
+ return slowIndex;
+
+ }
+}
+```
Python:
@@ -172,4 +190,4 @@ var removeElement = (nums, val) => {
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
\ No newline at end of file
+
diff --git "a/problems/0028.\345\256\236\347\216\260strStr.md" "b/problems/0028.\345\256\236\347\216\260strStr.md"
index f1de00f876..c6463e8bf8 100644
--- "a/problems/0028.\345\256\236\347\216\260strStr.md"
+++ "b/problems/0028.\345\256\236\347\216\260strStr.md"
@@ -565,6 +565,54 @@ public:
Java:
+```Java
+class Solution {
+ /**
+ * 基于窗口滑动的算法
+ *
+ * 时间复杂度:O(m*n)
+ * 空间复杂度:O(1)
+ * 注:n为haystack的长度,m为needle的长度
+ */
+ public int strStr(String haystack, String needle) {
+ int m = needle.length();
+ // 当 needle 是空字符串时我们应当返回 0
+ if (m == 0) {
+ return 0;
+ }
+ int n = haystack.length();
+ if (n < m) {
+ return -1;
+ }
+ int i = 0;
+ int j = 0;
+ while (i < n - m + 1) {
+ // 找到首字母相等
+ while (i < n && haystack.charAt(i) != needle.charAt(j)) {
+ i++;
+ }
+ if (i == n) {// 没有首字母相等的
+ return -1;
+ }
+ // 遍历后续字符,判断是否相等
+ i++;
+ j++;
+ while (i < n && j < m && haystack.charAt(i) == needle.charAt(j)) {
+ i++;
+ j++;
+ }
+ if (j == m) {// 找到
+ return i - j;
+ } else {// 未找到
+ i -= j - 1;
+ j = 0;
+ }
+ }
+ return -1;
+ }
+}
+```
+
```java
// 方法一
class Solution {
diff --git "a/problems/0055.\350\267\263\350\267\203\346\270\270\346\210\217.md" "b/problems/0055.\350\267\263\350\267\203\346\270\270\346\210\217.md"
index 179ac24630..a25c831a7b 100644
--- "a/problems/0055.\350\267\263\350\267\203\346\270\270\346\210\217.md"
+++ "b/problems/0055.\350\267\263\350\267\203\346\270\270\346\210\217.md"
@@ -107,7 +107,19 @@ class Solution {
```
Python:
-
+```python
+class Solution:
+ def canJump(self, nums: List[int]) -> bool:
+ cover = 0
+ if len(nums) == 1: return True
+ i = 0
+ # python不支持动态修改for循环中变量,使用while循环代替
+ while i <= cover:
+ cover = max(i + nums[i], cover)
+ if cover >= len(nums) - 1: return True
+ i += 1
+ return False
+```
Go:
diff --git "a/problems/0056.\345\220\210\345\271\266\345\214\272\351\227\264.md" "b/problems/0056.\345\220\210\345\271\266\345\214\272\351\227\264.md"
index f939325f9e..e84a1634a3 100644
--- "a/problems/0056.\345\220\210\345\271\266\345\214\272\351\227\264.md"
+++ "b/problems/0056.\345\220\210\345\271\266\345\214\272\351\227\264.md"
@@ -137,7 +137,35 @@ public:
Java:
+```java
+class Solution {
+ public int[][] merge(int[][] intervals) {
+ List res = new LinkedList<>();
+ Arrays.sort(intervals, new Comparator() {
+ @Override
+ public int compare(int[] o1, int[] o2) {
+ if (o1[0] != o2[0]) {
+ return Integer.compare(o1[0],o2[0]);
+ } else {
+ return Integer.compare(o1[1],o2[1]);
+ }
+ }
+ });
+ int start = intervals[0][0];
+ for (int i = 1; i < intervals.length; i++) {
+ if (intervals[i][0] > intervals[i - 1][1]) {
+ res.add(new int[]{start, intervals[i - 1][1]});
+ start = intervals[i][0];
+ } else {
+ intervals[i][1] = Math.max(intervals[i][1], intervals[i - 1][1]);
+ }
+ }
+ res.add(new int[]{start, intervals[intervals.length - 1][1]});
+ return res.toArray(new int[res.size()][]);
+ }
+}
+```
Python:
@@ -151,4 +179,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0070.\347\210\254\346\245\274\346\242\257\345\256\214\345\205\250\350\203\214\345\214\205\347\211\210\346\234\254.md" "b/problems/0070.\347\210\254\346\245\274\346\242\257\345\256\214\345\205\250\350\203\214\345\214\205\347\211\210\346\234\254.md"
index beda45d529..d6b1245083 100644
--- "a/problems/0070.\347\210\254\346\245\274\346\242\257\345\256\214\345\205\250\350\203\214\345\214\205\347\211\210\346\234\254.md"
+++ "b/problems/0070.\347\210\254\346\245\274\346\242\257\345\256\214\345\205\250\350\203\214\345\214\205\347\211\210\346\234\254.md"
@@ -127,7 +127,23 @@ public:
Java:
+```java
+class Solution {
+ public int climbStairs(int n) {
+ int[] dp = new int[n + 1];
+ int[] weight = {1,2};
+ dp[0] = 1;
+
+ for (int i = 0; i <= n; i++) {
+ for (int j = 0; j < weight.length; j++) {
+ if (i >= weight[j]) dp[i] += dp[i - weight[j]];
+ }
+ }
+ return dp[n];
+ }
+}
+```
Python:
@@ -141,4 +157,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0098.\351\252\214\350\257\201\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md" "b/problems/0098.\351\252\214\350\257\201\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
index ddd634b490..45f2308b12 100644
--- "a/problems/0098.\351\252\214\350\257\201\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
+++ "b/problems/0098.\351\252\214\350\257\201\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
@@ -254,6 +254,19 @@ public:
Java:
+```java
+class Solution {
+ public boolean isValidBST(TreeNode root) {
+ return isValidBST_2(root, Long.MIN_VALUE, Long.MAX_VALUE);
+ }
+
+ public boolean isValidBST_2 (TreeNode root, long lo, long hi) {
+ if (root == null) return true;
+ if (root.val >= hi || root.val <= lo) return false;
+ return isValidBST_2(root.left,lo,root.val) && isValidBST_2(root.right,root.val,hi);
+ }
+}
+```
Python:
@@ -286,4 +299,4 @@ func isBST(root *TreeNode, min, max int) bool {
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0101.\345\257\271\347\247\260\344\272\214\345\217\211\346\240\221.md" "b/problems/0101.\345\257\271\347\247\260\344\272\214\345\217\211\346\240\221.md"
index fdb0a929e2..b7d4155746 100644
--- "a/problems/0101.\345\257\271\347\247\260\344\272\214\345\217\211\346\240\221.md"
+++ "b/problems/0101.\345\257\271\347\247\260\344\272\214\345\217\211\346\240\221.md"
@@ -254,6 +254,7 @@ public:
## 其他语言版本
Java:
+
```Java
/**
* 递归法
@@ -263,12 +264,14 @@ Java:
}
private boolean compare(TreeNode left, TreeNode right) {
+
if (left == null && right != null) {
return false;
}
if (left != null && right == null) {
return false;
}
+
if (left == null && right == null) {
return true;
}
@@ -352,6 +355,7 @@ Java:
}
return true;
}
+
```
Python:
diff --git "a/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md" "b/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md"
index 0781e01d09..9b5f9ed5d8 100644
--- "a/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md"
+++ "b/problems/0102.\344\272\214\345\217\211\346\240\221\347\232\204\345\261\202\345\272\217\351\201\215\345\216\206.md"
@@ -420,54 +420,236 @@ public:
Java:
```Java
+// 102.二叉树的层序遍历
class Solution {
- public List> resList=new ArrayList>();
+ public List> resList = new ArrayList>();
+
public List> levelOrder(TreeNode root) {
//checkFun01(root,0);
checkFun02(root);
-
+
return resList;
}
- //DFS--递归方式
- public void checkFun01(TreeNode node,Integer deep){
- if(node==null) return;
+ //DFS--递归方式
+ public void checkFun01(TreeNode node, Integer deep) {
+ if (node == null) return;
deep++;
- if(resList.size() item=new ArrayList();
+ List item = new ArrayList();
resList.add(item);
}
- resList.get(deep-1).add(node.val);
-
- checkFun01(node.left,deep);
- checkFun01(node.right,deep);
+ resList.get(deep - 1).add(node.val);
+
+ checkFun01(node.left, deep);
+ checkFun01(node.right, deep);
}
-
+
//BFS--迭代方式--借助队列
- public void checkFun02(TreeNode node){
- if(node==null) return;
- Queue que=new LinkedList();
+ public void checkFun02(TreeNode node) {
+ if (node == null) return;
+ Queue que = new LinkedList();
que.offer(node);
- while(!que.isEmpty()){
- List itemList=new ArrayList();
- int len=que.size();
+ while (!que.isEmpty()) {
+ List itemList = new ArrayList();
+ int len = que.size();
- while(len>0){
- TreeNode tmpNode=que.poll();
+ while (len > 0) {
+ TreeNode tmpNode = que.poll();
itemList.add(tmpNode.val);
- if(tmpNode.left!=null) que.offer(tmpNode.left);
- if(tmpNode.right!=null) que.offer(tmpNode.right);
+ if (tmpNode.left != null) que.offer(tmpNode.left);
+ if (tmpNode.right != null) que.offer(tmpNode.right);
len--;
}
-
+
resList.add(itemList);
}
}
+}
+
+
+// 107. 二叉树的层序遍历 II
+public class N0107 {
+
+ /**
+ * 解法:队列,迭代。
+ * 层序遍历,再翻转数组即可。
+ */
+ public List> solution1(TreeNode root) {
+ List> list = new ArrayList<>();
+ Deque que = new LinkedList<>();
+
+ if (root == null) {
+ return list;
+ }
+
+ que.offerLast(root);
+ while (!que.isEmpty()) {
+ List levelList = new ArrayList<>();
+
+ int levelSize = que.size();
+ for (int i = 0; i < levelSize; i++) {
+ TreeNode peek = que.peekFirst();
+ levelList.add(que.pollFirst().val);
+
+ if (peek.left != null) {
+ que.offerLast(peek.left);
+ }
+ if (peek.right != null) {
+ que.offerLast(peek.right);
+ }
+ }
+ list.add(levelList);
+ }
+
+ List> result = new ArrayList<>();
+ for (int i = list.size() - 1; i >= 0; i-- ) {
+ result.add(list.get(i));
+ }
+
+ return result;
+ }
+}
+
+// 199.二叉树的右视图
+public class N0199 {
+ /**
+ * 解法:队列,迭代。
+ * 每次返回每层的最后一个字段即可。
+ *
+ * 小优化:每层右孩子先入队。代码略。
+ */
+ public List rightSideView(TreeNode root) {
+ List list = new ArrayList<>();
+ Deque que = new LinkedList<>();
+
+ if (root == null) {
+ return list;
+ }
+
+ que.offerLast(root);
+ while (!que.isEmpty()) {
+ int levelSize = que.size();
+
+ for (int i = 0; i < levelSize; i++) {
+ TreeNode poll = que.pollFirst();
+
+ if (poll.left != null) {
+ que.addLast(poll.left);
+ }
+ if (poll.right != null) {
+ que.addLast(poll.right);
+ }
+
+ if (i == levelSize - 1) {
+ list.add(poll.val);
+ }
+ }
+ }
+
+ return list;
+ }
+}
+
+// 637. 二叉树的层平均值
+public class N0637 {
+
+ /**
+ * 解法:队列,迭代。
+ * 每次返回每层的最后一个字段即可。
+ */
+ public List averageOfLevels(TreeNode root) {
+ List list = new ArrayList<>();
+ Deque que = new LinkedList<>();
+
+ if (root == null) {
+ return list;
+ }
+
+ que.offerLast(root);
+ while (!que.isEmpty()) {
+ TreeNode peek = que.peekFirst();
+
+ int levelSize = que.size();
+ double levelSum = 0.0;
+ for (int i = 0; i < levelSize; i++) {
+ TreeNode poll = que.pollFirst();
+
+ levelSum += poll.val;
+
+ if (poll.left != null) {
+ que.addLast(poll.left);
+ }
+ if (poll.right != null) {
+ que.addLast(poll.right);
+ }
+ }
+ list.add(levelSum / levelSize);
+ }
+ return list;
+ }
+}
+
+// 429. N 叉树的层序遍历
+public class N0429 {
+ /**
+ * 解法1:队列,迭代。
+ */
+ public List> levelOrder(Node root) {
+ List> list = new ArrayList<>();
+ Deque que = new LinkedList<>();
+
+ if (root == null) {
+ return list;
+ }
+
+ que.offerLast(root);
+ while (!que.isEmpty()) {
+ int levelSize = que.size();
+ List levelList = new ArrayList<>();
+
+ for (int i = 0; i < levelSize; i++) {
+ Node poll = que.pollFirst();
+
+ levelList.add(poll.val);
+
+ List children = poll.children;
+ if (children == null || children.size() == 0) {
+ continue;
+ }
+ for (Node child : children) {
+ if (child != null) {
+ que.offerLast(child);
+ }
+ }
+ }
+ list.add(levelList);
+ }
+
+ return list;
+ }
+
+ class Node {
+ public int val;
+ public List children;
+
+ public Node() {}
+
+ public Node(int _val) {
+ val = _val;
+ }
+
+ public Node(int _val, List _children) {
+ val = _val;
+ children = _children;
+ }
+ }
+}
```
diff --git "a/problems/0104.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\244\247\346\267\261\345\272\246.md" "b/problems/0104.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\244\247\346\267\261\345\272\246.md"
index 814beb55bc..ccecea43e7 100644
--- "a/problems/0104.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\244\247\346\267\261\345\272\246.md"
+++ "b/problems/0104.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\244\247\346\267\261\345\272\246.md"
@@ -232,6 +232,52 @@ public:
Java:
+```Java
+class Solution {
+ /**
+ * 递归法
+ */
+ public int maxDepth(TreeNode root) {
+ if (root == null) {
+ return 0;
+ }
+ int leftDepth = maxDepth(root.left);
+ int rightDepth = maxDepth(root.right);
+ return Math.max(leftDepth, rightDepth) + 1;
+
+ }
+}
+```
+
+```Java
+class Solution {
+ /**
+ * 迭代法,使用层序遍历
+ */
+ public int maxDepth(TreeNode root) {
+ if(root == null) {
+ return 0;
+ }
+ Deque deque = new LinkedList<>();
+ deque.offer(root);
+ int depth = 0;
+ while (!deque.isEmpty()) {
+ int size = deque.size();
+ depth++;
+ for (int i = 0; i < size; i++) {
+ TreeNode poll = deque.poll();
+ if (poll.left != null) {
+ deque.offer(poll.left);
+ }
+ if (poll.right != null) {
+ deque.offer(poll.right);
+ }
+ }
+ }
+ return depth;
+ }
+}
+```
Python:
@@ -239,10 +285,16 @@ Python:
Go:
-
+JavaScript
+```javascript
+var maxDepth = function(root) {
+ if (!root) return root
+ return 1 + Math.max(maxDepth(root.left), maxDepth(root.right))
+};
+```
-----------------------
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0106.\344\273\216\344\270\255\345\272\217\344\270\216\345\220\216\345\272\217\351\201\215\345\216\206\345\272\217\345\210\227\346\236\204\351\200\240\344\272\214\345\217\211\346\240\221.md" "b/problems/0106.\344\273\216\344\270\255\345\272\217\344\270\216\345\220\216\345\272\217\351\201\215\345\216\206\345\272\217\345\210\227\346\236\204\351\200\240\344\272\214\345\217\211\346\240\221.md"
index f1f30b7174..e51cf08bf3 100644
--- "a/problems/0106.\344\273\216\344\270\255\345\272\217\344\270\216\345\220\216\345\272\217\351\201\215\345\216\206\345\272\217\345\210\227\346\236\204\351\200\240\344\272\214\345\217\211\346\240\221.md"
+++ "b/problems/0106.\344\273\216\344\270\255\345\272\217\344\270\216\345\220\216\345\272\217\351\201\215\345\216\206\345\272\217\345\210\227\346\236\204\351\200\240\344\272\214\345\217\211\346\240\221.md"
@@ -582,7 +582,40 @@ tree2 的前序遍历是[1 2 3], 后序遍历是[3 2 1]。
Java:
-
+```java
+class Solution {
+ public TreeNode buildTree(int[] inorder, int[] postorder) {
+ return buildTree1(inorder, 0, inorder.length, postorder, 0, postorder.length);
+ }
+ public TreeNode buildTree1(int[] inorder, int inLeft, int inRight,
+ int[] postorder, int postLeft, int postRight) {
+ // 没有元素了
+ if (inRight - inLeft < 1) {
+ return null;
+ }
+ // 只有一个元素了
+ if (inRight - inLeft == 1) {
+ return new TreeNode(inorder[inLeft]);
+ }
+ // 后序数组postorder里最后一个即为根结点
+ int rootVal = postorder[postRight - 1];
+ TreeNode root = new TreeNode(rootVal);
+ int rootIndex = 0;
+ // 根据根结点的值找到该值在中序数组inorder里的位置
+ for (int i = inLeft; i < inRight; i++) {
+ if (inorder[i] == rootVal) {
+ rootIndex = i;
+ }
+ }
+ // 根据rootIndex划分左右子树
+ root.left = buildTree1(inorder, inLeft, rootIndex,
+ postorder, postLeft, postLeft + (rootIndex - inLeft));
+ root.right = buildTree1(inorder, rootIndex + 1, inRight,
+ postorder, postLeft + (rootIndex - inLeft), postRight - 1);
+ return root;
+ }
+}
+```
Python:
@@ -596,4 +629,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0108.\345\260\206\346\234\211\345\272\217\346\225\260\347\273\204\350\275\254\346\215\242\344\270\272\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md" "b/problems/0108.\345\260\206\346\234\211\345\272\217\346\225\260\347\273\204\350\275\254\346\215\242\344\270\272\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
index 12d47e6a01..93dc5fd683 100644
--- "a/problems/0108.\345\260\206\346\234\211\345\272\217\346\225\260\347\273\204\350\275\254\346\215\242\344\270\272\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
+++ "b/problems/0108.\345\260\206\346\234\211\345\272\217\346\225\260\347\273\204\350\275\254\346\215\242\344\270\272\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
@@ -209,7 +209,28 @@ public:
Java:
+```Java
+class Solution {
+ public TreeNode sortedArrayToBST(int[] nums) {
+ return sortedArrayToBST(nums, 0, nums.length);
+ }
+
+ public TreeNode sortedArrayToBST(int[] nums, int left, int right) {
+ if (left >= right) {
+ return null;
+ }
+ if (right - left == 1) {
+ return new TreeNode(nums[left]);
+ }
+ int mid = left + (right - left) / 2;
+ TreeNode root = new TreeNode(nums[mid]);
+ root.left = sortedArrayToBST(nums, left, mid);
+ root.right = sortedArrayToBST(nums, mid + 1, right);
+ return root;
+ }
+}
+```
Python:
@@ -223,4 +244,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0110.\345\271\263\350\241\241\344\272\214\345\217\211\346\240\221.md" "b/problems/0110.\345\271\263\350\241\241\344\272\214\345\217\211\346\240\221.md"
index ac66cc40f9..da71ccb269 100644
--- "a/problems/0110.\345\271\263\350\241\241\344\272\214\345\217\211\346\240\221.md"
+++ "b/problems/0110.\345\271\263\350\241\241\344\272\214\345\217\211\346\240\221.md"
@@ -355,6 +355,146 @@ public:
Java:
+```Java
+class Solution {
+ /**
+ * 递归法
+ */
+ public boolean isBalanced(TreeNode root) {
+ return getHeight(root) != -1;
+ }
+
+ private int getHeight(TreeNode root) {
+ if (root == null) {
+ return 0;
+ }
+ int leftHeight = getHeight(root.left);
+ if (leftHeight == -1) {
+ return -1;
+ }
+ int rightHeight = getHeight(root.right);
+ if (rightHeight == -1) {
+ return -1;
+ }
+ // 左右子树高度差大于1,return -1表示已经不是平衡树了
+ if (Math.abs(leftHeight - rightHeight) > 1) {
+ return -1;
+ }
+ return Math.max(leftHeight, rightHeight) + 1;
+ }
+}
+
+class Solution {
+ /**
+ * 迭代法,效率较低,计算高度时会重复遍历
+ * 时间复杂度:O(n^2)
+ */
+ public boolean isBalanced(TreeNode root) {
+ if (root == null) {
+ return true;
+ }
+ Stack stack = new Stack<>();
+ TreeNode pre = null;
+ while (root!= null || !stack.isEmpty()) {
+ while (root != null) {
+ stack.push(root);
+ root = root.left;
+ }
+ TreeNode inNode = stack.peek();
+ // 右结点为null或已经遍历过
+ if (inNode.right == null || inNode.right == pre) {
+ // 比较左右子树的高度差,输出
+ if (Math.abs(getHeight(inNode.left) - getHeight(inNode.right)) > 1) {
+ return false;
+ }
+ stack.pop();
+ pre = inNode;
+ root = null;// 当前结点下,没有要遍历的结点了
+ } else {
+ root = inNode.right;// 右结点还没遍历,遍历右结点
+ }
+ }
+ return true;
+ }
+
+ /**
+ * 层序遍历,求结点的高度
+ */
+ public int getHeight(TreeNode root) {
+ if (root == null) {
+ return 0;
+ }
+ Deque deque = new LinkedList<>();
+ deque.offer(root);
+ int depth = 0;
+ while (!deque.isEmpty()) {
+ int size = deque.size();
+ depth++;
+ for (int i = 0; i < size; i++) {
+ TreeNode poll = deque.poll();
+ if (poll.left != null) {
+ deque.offer(poll.left);
+ }
+ if (poll.right != null) {
+ deque.offer(poll.right);
+ }
+ }
+ }
+ return depth;
+ }
+}
+
+class Solution {
+ /**
+ * 优化迭代法,针对暴力迭代法的getHeight方法做优化,利用TreeNode.val来保存当前结点的高度,这样就不会有重复遍历
+ * 获取高度算法时间复杂度可以降到O(1),总的时间复杂度降为O(n)。
+ *
+ * 时间复杂度:O(n)
+ */
+ public boolean isBalanced(TreeNode root) {
+ if (root == null) {
+ return true;
+ }
+ Stack stack = new Stack<>();
+ TreeNode pre = null;
+ while (root != null || !stack.isEmpty()) {
+ while (root != null) {
+ stack.push(root);
+ root = root.left;
+ }
+ TreeNode inNode = stack.peek();
+ // 右结点为null或已经遍历过
+ if (inNode.right == null || inNode.right == pre) {
+ // 输出
+ if (Math.abs(getHeight(inNode.left) - getHeight(inNode.right)) > 1) {
+ return false;
+ }
+ stack.pop();
+ pre = inNode;
+ root = null;// 当前结点下,没有要遍历的结点了
+ } else {
+ root = inNode.right;// 右结点还没遍历,遍历右结点
+ }
+ }
+ return true;
+ }
+
+ /**
+ * 求结点的高度
+ */
+ public int getHeight(TreeNode root) {
+ if (root == null) {
+ return 0;
+ }
+ int leftHeight = root.left != null ? root.left.val : 0;
+ int rightHeight = root.right != null ? root.right.val : 0;
+ int height = Math.max(leftHeight, rightHeight) + 1;
+ root.val = height;// 用TreeNode.val来保存当前结点的高度
+ return height;
+ }
+}
+// LeetCode题解链接:https://leetcode-cn.com/problems/balanced-binary-tree/solution/110-ping-heng-er-cha-shu-di-gui-fa-bao-l-yqr3/
+```
Python:
@@ -401,4 +541,4 @@ func abs(a int)int{
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
\ No newline at end of file
+
diff --git "a/problems/0111.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\260\217\346\267\261\345\272\246.md" "b/problems/0111.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\260\217\346\267\261\345\272\246.md"
index 6c6b4632d4..01b6c89c6f 100644
--- "a/problems/0111.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\260\217\346\267\261\345\272\246.md"
+++ "b/problems/0111.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\345\260\217\346\267\261\345\272\246.md"
@@ -195,6 +195,64 @@ public:
Java:
+```Java
+class Solution {
+ /**
+ * 递归法,相比求MaxDepth要复杂点
+ * 因为最小深度是从根节点到最近**叶子节点**的最短路径上的节点数量
+ */
+ public int minDepth(TreeNode root) {
+ if (root == null) {
+ return 0;
+ }
+ int leftDepth = minDepth(root.left);
+ int rightDepth = minDepth(root.right);
+ if (root.left == null) {
+ return rightDepth + 1;
+ }
+ if (root.right == null) {
+ return leftDepth + 1;
+ }
+ // 左右结点都不为null
+ return Math.min(leftDepth, rightDepth) + 1;
+ }
+}
+```
+
+```Java
+class Solution {
+ /**
+ * 迭代法,层序遍历
+ */
+ public int minDepth(TreeNode root) {
+ if (root == null) {
+ return 0;
+ }
+ Deque deque = new LinkedList<>();
+ deque.offer(root);
+ int depth = 0;
+ while (!deque.isEmpty()) {
+ int size = deque.size();
+ depth++;
+ for (int i = 0; i < size; i++) {
+ TreeNode poll = deque.poll();
+ if (poll.left == null && poll.right == null) {
+ // 是叶子结点,直接返回depth,因为从上往下遍历,所以该值就是最小值
+ return depth;
+ }
+ if (poll.left != null) {
+ deque.offer(poll.left);
+ }
+ if (poll.right != null) {
+ deque.offer(poll.right);
+ }
+ }
+ }
+ return depth;
+ }
+}
+```
+
Python:
递归法:
@@ -250,4 +308,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
\ No newline at end of file
+
diff --git "a/problems/0112.\350\267\257\345\276\204\346\200\273\345\222\214.md" "b/problems/0112.\350\267\257\345\276\204\346\200\273\345\222\214.md"
index 718a2f5bd3..1b75113e4d 100644
--- "a/problems/0112.\350\267\257\345\276\204\346\200\273\345\222\214.md"
+++ "b/problems/0112.\350\267\257\345\276\204\346\200\273\345\222\214.md"
@@ -305,7 +305,34 @@ public:
Java:
+```Java
+class Solution {
+ public boolean hasPathSum(TreeNode root, int targetSum) {
+ if (root == null) {
+ return false;
+ }
+ targetSum -= root.val;
+ // 叶子结点
+ if (root.left == null && root.right == null) {
+ return targetSum == 0;
+ }
+ if (root.left != null) {
+ boolean left = hasPathSum(root.left, targetSum);
+ if (left) {// 已经找到
+ return true;
+ }
+ }
+ if (root.right != null) {
+ boolean right = hasPathSum(root.right, targetSum);
+ if (right) {// 已经找到
+ return true;
+ }
+ }
+ return false;
+ }
+}
+```
Python:
@@ -387,4 +414,4 @@ let pathSum = function (root, targetSum) {
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0122.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272II.md" "b/problems/0122.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272II.md"
index 1a9b4f7f6c..249d44a778 100644
--- "a/problems/0122.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272II.md"
+++ "b/problems/0122.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272II.md"
@@ -135,10 +135,33 @@ public:
Java:
-
+```java
+class Solution {
+ public int maxProfit(int[] prices) {
+ int sum = 0;
+ int profit = 0;
+ int buy = prices[0];
+ for (int i = 1; i < prices.length; i++) {
+ profit = prices[i] - buy;
+ if (profit > 0) {
+ sum += profit;
+ }
+ buy = prices[i];
+ }
+ return sum;
+ }
+}
+```
Python:
-
+```python
+class Solution:
+ def maxProfit(self, prices: List[int]) -> int:
+ result = 0
+ for i in range(1, len(prices)):
+ result += max(prices[i] - prices[i - 1], 0)
+ return result
+```
Go:
@@ -149,4 +172,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0134.\345\212\240\346\262\271\347\253\231.md" "b/problems/0134.\345\212\240\346\262\271\347\253\231.md"
index 9c72e84d9c..393e46279f 100644
--- "a/problems/0134.\345\212\240\346\262\271\347\253\231.md"
+++ "b/problems/0134.\345\212\240\346\262\271\347\253\231.md"
@@ -199,7 +199,28 @@ public:
Java:
+```java
+class Solution {
+ public int canCompleteCircuit(int[] gas, int[] cost) {
+ int sum = 0;
+ int min = 0;
+ for (int i = 0; i < gas.length; i++) {
+ sum += (gas[i] - cost[i]);
+ min = Math.min(sum, min);
+ }
+
+ if (sum < 0) return -1;
+ if (min >= 0) return 0;
+
+ for (int i = gas.length - 1; i > 0; i--) {
+ min += (gas[i] - cost[i]);
+ if (min >= 0) return i;
+ }
+ return -1;
+ }
+}
+```
Python:
@@ -213,4 +234,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0135.\345\210\206\345\217\221\347\263\226\346\236\234.md" "b/problems/0135.\345\210\206\345\217\221\347\263\226\346\236\234.md"
index 0595cff663..fedf876534 100644
--- "a/problems/0135.\345\210\206\345\217\221\347\263\226\346\236\234.md"
+++ "b/problems/0135.\345\210\206\345\217\221\347\263\226\346\236\234.md"
@@ -130,7 +130,35 @@ public:
Java:
+```java
+class Solution {
+ public int candy(int[] ratings) {
+ int[] candy = new int[ratings.length];
+ for (int i = 0; i < candy.length; i++) {
+ candy[i] = 1;
+ }
+ for (int i = 1; i < ratings.length; i++) {
+ if (ratings[i] > ratings[i - 1]) {
+ candy[i] = candy[i - 1] + 1;
+ }
+ }
+
+ for (int i = ratings.length - 2; i >= 0; i--) {
+ if (ratings[i] > ratings[i + 1]) {
+ candy[i] = Math.max(candy[i],candy[i + 1] + 1);
+ }
+ }
+
+ int count = 0;
+ for (int i = 0; i < candy.length; i++) {
+ count += candy[i];
+ }
+
+ return count;
+ }
+}
+```
Python:
@@ -144,4 +172,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0139.\345\215\225\350\257\215\346\213\206\345\210\206.md" "b/problems/0139.\345\215\225\350\257\215\346\213\206\345\210\206.md"
index ec9965651e..c6d8e43b35 100644
--- "a/problems/0139.\345\215\225\350\257\215\346\213\206\345\210\206.md"
+++ "b/problems/0139.\345\215\225\350\257\215\346\213\206\345\210\206.md"
@@ -232,7 +232,23 @@ public:
Java:
+```java
+class Solution {
+ public boolean wordBreak(String s, List wordDict) {
+ boolean[] valid = new boolean[s.length() + 1];
+ valid[0] = true;
+ for (int i = 1; i <= s.length(); i++) {
+ for (int j = 0; j < i; j++) {
+ if (wordDict.contains(s.substring(j,i)) && valid[j]) {
+ valid[i] = true;
+ }
+ }
+ }
+ return valid[s.length()];
+ }
+}
+```
Python:
@@ -246,4 +262,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0142.\347\216\257\345\275\242\351\223\276\350\241\250II.md" "b/problems/0142.\347\216\257\345\275\242\351\223\276\350\241\250II.md"
index 9622affc86..7556b854b6 100644
--- "a/problems/0142.\347\216\257\345\275\242\351\223\276\350\241\250II.md"
+++ "b/problems/0142.\347\216\257\345\275\242\351\223\276\350\241\250II.md"
@@ -186,6 +186,29 @@ public:
Java:
+```java
+public class Solution {
+ public ListNode detectCycle(ListNode head) {
+ ListNode slow = head;
+ ListNode fast = head;
+ while (fast != null && fast.next != null) {
+ slow = slow.next;
+ fast = fast.next.next;
+ if (slow == fast) {// 有环
+ ListNode index1 = fast;
+ ListNode index2 = head;
+ // 两个指针,从头结点和相遇结点,各走一步,直到相遇,相遇点即为环入口
+ while (index1 != index2) {
+ index1 = index1.next;
+ index2 = index2.next;
+ }
+ return index1;
+ }
+ }
+ return null;
+ }
+}
+```
Python:
diff --git "a/problems/0151.\347\277\273\350\275\254\345\255\227\347\254\246\344\270\262\351\207\214\347\232\204\345\215\225\350\257\215.md" "b/problems/0151.\347\277\273\350\275\254\345\255\227\347\254\246\344\270\262\351\207\214\347\232\204\345\215\225\350\257\215.md"
index d81c139dfa..d9ecfc1252 100644
--- "a/problems/0151.\347\277\273\350\275\254\345\255\227\347\254\246\344\270\262\351\207\214\347\232\204\345\215\225\350\257\215.md"
+++ "b/problems/0151.\347\277\273\350\275\254\345\255\227\347\254\246\344\270\262\351\207\214\347\232\204\345\215\225\350\257\215.md"
@@ -213,6 +213,74 @@ public:
Java:
+```Java
+class Solution {
+ /**
+ * 不使用Java内置方法实现
+ *
+ * 1.去除首尾以及中间多余空格
+ * 2.反转整个字符串
+ * 3.反转各个单词
+ */
+ public String reverseWords(String s) {
+ // System.out.println("ReverseWords.reverseWords2() called with: s = [" + s + "]");
+ // 1.去除首尾以及中间多余空格
+ StringBuilder sb = removeSpace(s);
+ // 2.反转整个字符串
+ reverseString(sb, 0, sb.length() - 1);
+ // 3.反转各个单词
+ reverseEachWord(sb);
+ return sb.toString();
+ }
+
+ private StringBuilder removeSpace(String s) {
+ // System.out.println("ReverseWords.removeSpace() called with: s = [" + s + "]");
+ int start = 0;
+ int end = s.length() - 1;
+ while (s.charAt(start) == ' ') start++;
+ while (s.charAt(end) == ' ') end--;
+ StringBuilder sb = new StringBuilder();
+ while (start <= end) {
+ char c = s.charAt(start);
+ if (c != ' ' || sb.charAt(sb.length() - 1) != ' ') {
+ sb.append(c);
+ }
+ start++;
+ }
+ // System.out.println("ReverseWords.removeSpace returned: sb = [" + sb + "]");
+ return sb;
+ }
+
+ /**
+ * 反转字符串指定区间[start, end]的字符
+ */
+ public void reverseString(StringBuilder sb, int start, int end) {
+ // System.out.println("ReverseWords.reverseString() called with: sb = [" + sb + "], start = [" + start + "], end = [" + end + "]");
+ while (start < end) {
+ char temp = sb.charAt(start);
+ sb.setCharAt(start, sb.charAt(end));
+ sb.setCharAt(end, temp);
+ start++;
+ end--;
+ }
+ // System.out.println("ReverseWords.reverseString returned: sb = [" + sb + "]");
+ }
+
+ private void reverseEachWord(StringBuilder sb) {
+ int start = 0;
+ int end = 1;
+ int n = sb.length();
+ while (start < n) {
+ while (end < n && sb.charAt(end) != ' ') {
+ end++;
+ }
+ reverseString(sb, start, end - 1);
+ start = end + 1;
+ end = start + 1;
+ }
+ }
+}
+```
Python:
diff --git "a/problems/0206.\347\277\273\350\275\254\351\223\276\350\241\250.md" "b/problems/0206.\347\277\273\350\275\254\351\223\276\350\241\250.md"
index b465cdf9d9..886bbfcdb6 100644
--- "a/problems/0206.\347\277\273\350\275\254\351\223\276\350\241\250.md"
+++ "b/problems/0206.\347\277\273\350\275\254\351\223\276\350\241\250.md"
@@ -102,7 +102,45 @@ public:
Java:
+```java
+// 双指针
+class Solution {
+ public ListNode reverseList(ListNode head) {
+ ListNode prev = null;
+ ListNode cur = head;
+ ListNode temp = null;
+ while (cur != null) {
+ temp = cur.next;// 保存下一个节点
+ cur.next = prev;
+ prev = cur;
+ cur = temp;
+ }
+ return prev;
+ }
+}
+```
+```java
+// 递归
+class Solution {
+ public ListNode reverseList(ListNode head) {
+ return reverse(null, head);
+ }
+
+ private ListNode reverse(ListNode prev, ListNode cur) {
+ if (cur == null) {
+ return prev;
+ }
+ ListNode temp = null;
+ temp = cur.next;// 先保存下一个节点
+ cur.next = prev;// 反转
+ // 更新prev、cur位置
+ prev = cur;
+ cur = temp;
+ return reverse(prev, cur);
+ }
+}
+```
Python:
@@ -116,4 +154,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0209.\351\225\277\345\272\246\346\234\200\345\260\217\347\232\204\345\255\220\346\225\260\347\273\204.md" "b/problems/0209.\351\225\277\345\272\246\346\234\200\345\260\217\347\232\204\345\255\220\346\225\260\347\273\204.md"
index 1b00c4e360..0aaa466ea5 100644
--- "a/problems/0209.\351\225\277\345\272\246\346\234\200\345\260\217\347\232\204\345\255\220\346\225\260\347\273\204.md"
+++ "b/problems/0209.\351\225\277\345\272\246\346\234\200\345\260\217\347\232\204\345\255\220\346\225\260\347\273\204.md"
@@ -148,7 +148,25 @@ class Solution:
Java:
+```java
+class Solution {
+ // 滑动窗口
+ public int minSubArrayLen(int s, int[] nums) {
+ int left = 0;
+ int sum = 0;
+ int result = Integer.MAX_VALUE;
+ for (int right = 0; right < nums.length; right++) {
+ sum += nums[right];
+ while (sum >= s) {
+ result = Math.min(result, right - left + 1);
+ sum -= nums[left++];
+ }
+ }
+ return result == Integer.MAX_VALUE ? 0 : result;
+ }
+}
+```
Python:
@@ -177,4 +195,4 @@ var minSubArrayLen = (target, nums) => {
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0216.\347\273\204\345\220\210\346\200\273\345\222\214III.md" "b/problems/0216.\347\273\204\345\220\210\346\200\273\345\222\214III.md"
index 11a8eb8f1e..21230e0f9f 100644
--- "a/problems/0216.\347\273\204\345\220\210\346\200\273\345\222\214III.md"
+++ "b/problems/0216.\347\273\204\345\220\210\346\200\273\345\222\214III.md"
@@ -227,7 +227,39 @@ public:
Java:
+```java
+class Solution {
+ List> res = new ArrayList<>();
+ List list = new ArrayList<>();
+
+ public List> combinationSum3(int k, int n) {
+ res.clear();
+ list.clear();
+ backtracking(k, n, 9);
+ return res;
+ }
+
+ private void backtracking(int k, int n, int maxNum) {
+ if (k == 0 && n == 0) {
+ res.add(new ArrayList<>(list));
+ return;
+ }
+ // 因为不能重复,并且单个数字最大值是maxNum,所以sum最大值为
+ // (maxNum + (maxNum - 1) + ... + (maxNum - k + 1)) == k * maxNum - k*(k - 1) / 2
+ if (maxNum == 0
+ || n > k * maxNum - k * (k - 1) / 2
+ || n < (1 + k) * k / 2) {
+ return;
+ }
+ list.add(maxNum);
+ backtracking(k - 1, n - maxNum, maxNum - 1);
+ list.remove(list.size() - 1);
+ backtracking(k, n, maxNum - 1);
+ }
+
+}
+```
Python:
@@ -241,4 +273,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0222.\345\256\214\345\205\250\344\272\214\345\217\211\346\240\221\347\232\204\350\212\202\347\202\271\344\270\252\346\225\260.md" "b/problems/0222.\345\256\214\345\205\250\344\272\214\345\217\211\346\240\221\347\232\204\350\212\202\347\202\271\344\270\252\346\225\260.md"
index 367fa717b9..b28b8dfb0b 100644
--- "a/problems/0222.\345\256\214\345\205\250\344\272\214\345\217\211\346\240\221\347\232\204\350\212\202\347\202\271\344\270\252\346\225\260.md"
+++ "b/problems/0222.\345\256\214\345\205\250\344\272\214\345\217\211\346\240\221\347\232\204\350\212\202\347\202\271\344\270\252\346\225\260.md"
@@ -194,7 +194,49 @@ public:
Java:
+```java
+class Solution {
+ // 通用递归解法
+ public int countNodes(TreeNode root) {
+ if(root == null) {
+ return 0;
+ }
+ return countNodes(root.left) + countNodes(root.right) + 1;
+ }
+}
+```
+
+```java
+class Solution {
+ /**
+ * 针对完全二叉树的解法
+ *
+ * 满二叉树的结点数为:2^depth - 1
+ */
+ public int countNodes(TreeNode root) {
+ if(root == null) {
+ return 0;
+ }
+ int leftDepth = getDepth(root.left);
+ int rightDepth = getDepth(root.right);
+ if (leftDepth == rightDepth) {// 左子树是满二叉树
+ // 2^leftDepth其实是 (2^leftDepth - 1) + 1 ,左子树 + 根结点
+ return (1 << leftDepth) + countNodes(root.right);
+ } else {// 右子树是满二叉树
+ return (1 << rightDepth) + countNodes(root.left);
+ }
+ }
+ private int getDepth(TreeNode root) {
+ int depth = 0;
+ while (root != null) {
+ root = root.left;
+ depth++;
+ }
+ return depth;
+ }
+}
+```
Python:
@@ -208,4 +250,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0226.\347\277\273\350\275\254\344\272\214\345\217\211\346\240\221.md" "b/problems/0226.\347\277\273\350\275\254\344\272\214\345\217\211\346\240\221.md"
index f5626ea0f2..3b96b4f6dc 100644
--- "a/problems/0226.\347\277\273\350\275\254\344\272\214\345\217\211\346\240\221.md"
+++ "b/problems/0226.\347\277\273\350\275\254\344\272\214\345\217\211\346\240\221.md"
@@ -204,6 +204,29 @@ public:
Java:
+```Java
+class Solution {
+ /**
+ * 前后序遍历都可以
+ * 中序不行,因为先左孩子交换孩子,再根交换孩子(做完后,右孩子已经变成了原来的左孩子),再右孩子交换孩子(此时其实是对原来的左孩子做交换)
+ */
+ public TreeNode invertTree(TreeNode root) {
+ if (root == null) {
+ return null;
+ }
+ invertTree(root.left);
+ invertTree(root.right);
+ swapChildren(root);
+ return root;
+ }
+
+ private void swapChildren(TreeNode root) {
+ TreeNode tmp = root.left;
+ root.left = root.right;
+ root.right = tmp;
+ }
+}
+```
Python:
@@ -230,4 +253,4 @@ func invertTree(root *TreeNode) *TreeNode {
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0235.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md" "b/problems/0235.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
index cb9de8b0ed..93642de5d5 100644
--- "a/problems/0235.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
+++ "b/problems/0235.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
@@ -29,7 +29,7 @@
输入: root = [6,2,8,0,4,7,9,null,null,3,5], p = 2, q = 4
输出: 2
解释: 节点 2 和节点 4 的最近公共祖先是 2, 因为根据定义最近公共祖先节点可以为节点本身。
-
+
说明:
@@ -229,7 +229,22 @@ public:
Java:
-
+```java
+class Solution {
+ public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
+ while (true) {
+ if (root.val > p.val && root.val > q.val) {
+ root = root.left;
+ } else if (root.val < p.val && root.val < q.val) {
+ root = root.right;
+ } else {
+ break;
+ }
+ }
+ return root;
+ }
+}
+```
Python:
@@ -243,4 +258,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md" "b/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
index 17096d489b..bd31209693 100644
--- "a/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
+++ "b/problems/0236.\344\272\214\345\217\211\346\240\221\347\232\204\346\234\200\350\277\221\345\205\254\345\205\261\347\245\226\345\205\210.md"
@@ -224,6 +224,43 @@ public:
Java:
+```Java
+class Solution {
+ public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
+ return lowestCommonAncestor1(root, p, q);
+
+ }
+ public TreeNode lowestCommonAncestor1(TreeNode root, TreeNode p, TreeNode q) {
+ if (root == null || root == p || root == q) {
+ return root;
+ }
+ TreeNode left = lowestCommonAncestor1(root.left, p, q);
+ TreeNode right = lowestCommonAncestor1(root.right, p, q);
+ if (left != null && right != null) {// 左右子树分别找到了,说明此时的root就是要求的结果
+ return root;
+ }
+ if (left == null) {
+ return right;
+ }
+ return left;
+ }
+}
+```
+
+```java
+// 代码精简版
+class Solution {
+ TreeNode pre;
+ public TreeNode lowestCommonAncestor(TreeNode root, TreeNode p, TreeNode q) {
+ if (root == null || root.val == p.val ||root.val == q.val) return root;
+ TreeNode left = lowestCommonAncestor(root.left,p,q);
+ TreeNode right = lowestCommonAncestor(root.right,p,q);
+ if (left != null && right != null) return root;
+ else if (left == null && right != null) return right;
+ else if (left != null && right == null) return left;
+ else return null;
+ }
+}
Python:
@@ -237,4 +274,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0242.\346\234\211\346\225\210\347\232\204\345\255\227\346\257\215\345\274\202\344\275\215\350\257\215.md" "b/problems/0242.\346\234\211\346\225\210\347\232\204\345\255\227\346\257\215\345\274\202\344\275\215\350\257\215.md"
index 8918662047..1927f47627 100644
--- "a/problems/0242.\346\234\211\346\225\210\347\232\204\345\255\227\346\257\215\345\274\202\344\275\215\350\257\215.md"
+++ "b/problems/0242.\346\234\211\346\225\210\347\232\204\345\255\227\346\257\215\345\274\202\344\275\215\350\257\215.md"
@@ -88,6 +88,7 @@ Java:
```java
class Solution {
public boolean isAnagram(String s, String t) {
+
int[] record = new int[26];
for (char c : s.toCharArray()) {
record[c - 'a'] += 1;
@@ -138,4 +139,4 @@ func isAnagram(s string, t string) bool {
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0257.\344\272\214\345\217\211\346\240\221\347\232\204\346\211\200\346\234\211\350\267\257\345\276\204.md" "b/problems/0257.\344\272\214\345\217\211\346\240\221\347\232\204\346\211\200\346\234\211\350\267\257\345\276\204.md"
index a104b27c08..73387257b8 100644
--- "a/problems/0257.\344\272\214\345\217\211\346\240\221\347\232\204\346\211\200\346\234\211\350\267\257\345\276\204.md"
+++ "b/problems/0257.\344\272\214\345\217\211\346\240\221\347\232\204\346\211\200\346\234\211\350\267\257\345\276\204.md"
@@ -280,9 +280,48 @@ public:
## 其他语言版本
-
Java:
+```Java
+class Solution {
+ /**
+ * 递归法
+ */
+ public List binaryTreePaths(TreeNode root) {
+ List res = new ArrayList<>();
+ if (root == null) {
+ return res;
+ }
+ List paths = new ArrayList<>();
+ traversal(root, paths, res);
+ return res;
+ }
+
+ private void traversal(TreeNode root, List paths, List res) {
+ paths.add(root.val);
+ // 叶子结点
+ if (root.left == null && root.right == null) {
+ // 输出
+ StringBuilder sb = new StringBuilder();
+ for (int i = 0; i < paths.size() - 1; i++) {
+ sb.append(paths.get(i)).append("->");
+ }
+ sb.append(paths.get(paths.size() - 1));
+ res.add(sb.toString());
+ return;
+ }
+ if (root.left != null) {
+ traversal(root.left, paths, res);
+ paths.remove(paths.size() - 1);// 回溯
+ }
+ if (root.right != null) {
+ traversal(root.right, paths, res);
+ paths.remove(paths.size() - 1);// 回溯
+ }
+ }
+}
+
+```
Python:
@@ -296,4 +335,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0347.\345\211\215K\344\270\252\351\253\230\351\242\221\345\205\203\347\264\240.md" "b/problems/0347.\345\211\215K\344\270\252\351\253\230\351\242\221\345\205\203\347\264\240.md"
index 1902bd6888..0e70f5530c 100644
--- "a/problems/0347.\345\211\215K\344\270\252\351\253\230\351\242\221\345\205\203\347\264\240.md"
+++ "b/problems/0347.\345\211\215K\344\270\252\351\253\230\351\242\221\345\205\203\347\264\240.md"
@@ -133,6 +133,32 @@ public:
Java:
+```java
+
+class Solution {
+ public int[] topKFrequent(int[] nums, int k) {
+ int[] result = new int[k];
+ HashMap map = new HashMap<>();
+ for (int num : nums) {
+ map.put(num, map.getOrDefault(num, 0) + 1);
+ }
+
+ Set> entries = map.entrySet();
+ // 根据map的value值正序排,相当于一个小顶堆
+ PriorityQueue> queue = new PriorityQueue<>((o1, o2) -> o1.getValue() - o2.getValue());
+ for (Map.Entry entry : entries) {
+ queue.offer(entry);
+ if (queue.size() > k) {
+ queue.poll();
+ }
+ }
+ for (int i = k - 1; i >= 0; i--) {
+ result[i] = queue.poll().getKey();
+ }
+ return result;
+ }
+}
+```
Python:
diff --git "a/problems/0377.\347\273\204\345\220\210\346\200\273\345\222\214\342\205\243.md" "b/problems/0377.\347\273\204\345\220\210\346\200\273\345\222\214\342\205\243.md"
index 8f8cfd86b6..eca9a13b70 100644
--- "a/problems/0377.\347\273\204\345\220\210\346\200\273\345\222\214\342\205\243.md"
+++ "b/problems/0377.\347\273\204\345\220\210\346\200\273\345\222\214\342\205\243.md"
@@ -147,6 +147,7 @@ C++测试用例有超过两个树相加超过int的数据,所以需要在if里
Java:
+
```Java
class Solution {
public int combinationSum4(int[] nums, int target) {
@@ -163,10 +164,23 @@ class Solution {
}
}
-```
Python:
+```python
+class Solution:
+ def combinationSum4(self, nums, target):
+ dp = [0] * (target + 1)
+ dp[0] = 1
+
+ for i in range(1, target+1):
+ for j in nums:
+ if i >= j:
+ dp[i] += dp[i - j]
+
+ return dp[-1]
+```
+
Go:
@@ -177,4 +191,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0404.\345\267\246\345\217\266\345\255\220\344\271\213\345\222\214.md" "b/problems/0404.\345\267\246\345\217\266\345\255\220\344\271\213\345\222\214.md"
index da4ed66661..0290dccd70 100644
--- "a/problems/0404.\345\267\246\345\217\266\345\255\220\344\271\213\345\222\214.md"
+++ "b/problems/0404.\345\267\246\345\217\266\345\255\220\344\271\213\345\222\214.md"
@@ -204,7 +204,6 @@ class Solution {
-
Python:
@@ -217,5 +216,6 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
+
diff --git "a/problems/0406.\346\240\271\346\215\256\350\272\253\351\253\230\351\207\215\345\273\272\351\230\237\345\210\227.md" "b/problems/0406.\346\240\271\346\215\256\350\272\253\351\253\230\351\207\215\345\273\272\351\230\237\345\210\227.md"
index b5260a8c19..2ef0a2fdd2 100644
--- "a/problems/0406.\346\240\271\346\215\256\350\272\253\351\253\230\351\207\215\345\273\272\351\230\237\345\210\227.md"
+++ "b/problems/0406.\346\240\271\346\215\256\350\272\253\351\253\230\351\207\215\345\273\272\351\230\237\345\210\227.md"
@@ -185,7 +185,30 @@ public:
Java:
+```java
+class Solution {
+ public int[][] reconstructQueue(int[][] people) {
+ Arrays.sort(people, new Comparator() {
+ @Override
+ public int compare(int[] o1, int[] o2) {
+ if (o1[0] != o2[0]) {
+ return Integer.compare(o2[0],o1[0]);
+ } else {
+ return Integer.compare(o1[1],o2[1]);
+ }
+ }
+ });
+ LinkedList que = new LinkedList<>();
+
+ for (int[] p : people) {
+ que.add(p[1],p);
+ }
+
+ return que.toArray(new int[people.length][]);
+ }
+}
+```
Python:
@@ -199,4 +222,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0435.\346\227\240\351\207\215\345\217\240\345\214\272\351\227\264.md" "b/problems/0435.\346\227\240\351\207\215\345\217\240\345\214\272\351\227\264.md"
index 3df496f4e1..4341f3b8a9 100644
--- "a/problems/0435.\346\227\240\351\207\215\345\217\240\345\214\272\351\227\264.md"
+++ "b/problems/0435.\346\227\240\351\207\215\345\217\240\345\214\272\351\227\264.md"
@@ -182,7 +182,34 @@ public:
Java:
+```java
+class Solution {
+ public int eraseOverlapIntervals(int[][] intervals) {
+ if (intervals.length < 2) return 0;
+ Arrays.sort(intervals, new Comparator() {
+ @Override
+ public int compare(int[] o1, int[] o2) {
+ if (o1[0] != o2[0]) {
+ return Integer.compare(o1[1],o2[1]);
+ } else {
+ return Integer.compare(o2[0],o1[0]);
+ }
+ }
+ });
+ int count = 0;
+ int edge = intervals[0][1];
+ for (int i = 1; i < intervals.length; i++) {
+ if (intervals[i][0] < edge) {
+ count++;
+ } else {
+ edge = intervals[i][1];
+ }
+ }
+ return count;
+ }
+}
+```
Python:
@@ -196,4 +223,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0450.\345\210\240\351\231\244\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\350\212\202\347\202\271.md" "b/problems/0450.\345\210\240\351\231\244\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\350\212\202\347\202\271.md"
index 6e38b4feb8..604fb37602 100644
--- "a/problems/0450.\345\210\240\351\231\244\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\350\212\202\347\202\271.md"
+++ "b/problems/0450.\345\210\240\351\231\244\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\350\212\202\347\202\271.md"
@@ -251,7 +251,34 @@ public:
Java:
+```java
+class Solution {
+ public TreeNode deleteNode(TreeNode root, int key) {
+ root = delete(root,key);
+ return root;
+ }
+ private TreeNode delete(TreeNode root, int key) {
+ if (root == null) return null;
+
+ if (root.val > key) {
+ root.left = delete(root.left,key);
+ } else if (root.val < key) {
+ root.right = delete(root.right,key);
+ } else {
+ if (root.left == null) return root.right;
+ if (root.right == null) return root.left;
+ TreeNode tmp = root.right;
+ while (tmp.left != null) {
+ tmp = tmp.left;
+ }
+ root.val = tmp.val;
+ root.right = delete(root.right,tmp.val);
+ }
+ return root;
+ }
+}
+```
Python:
@@ -303,4 +330,4 @@ func deleteNode1(root *TreeNode)*TreeNode{
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0452.\347\224\250\346\234\200\345\260\221\346\225\260\351\207\217\347\232\204\347\256\255\345\274\225\347\210\206\346\260\224\347\220\203.md" "b/problems/0452.\347\224\250\346\234\200\345\260\221\346\225\260\351\207\217\347\232\204\347\256\255\345\274\225\347\210\206\346\260\224\347\220\203.md"
index 7372ea929b..f62fb15306 100644
--- "a/problems/0452.\347\224\250\346\234\200\345\260\221\346\225\260\351\207\217\347\232\204\347\256\255\345\274\225\347\210\206\346\260\224\347\220\203.md"
+++ "b/problems/0452.\347\224\250\346\234\200\345\260\221\346\225\260\351\207\217\347\232\204\347\256\255\345\274\225\347\210\206\346\260\224\347\220\203.md"
@@ -139,7 +139,32 @@ public:
Java:
-
+```java
+class Solution {
+ public int findMinArrowShots(int[][] points) {
+ Arrays.sort(points, new Comparator() {
+ @Override
+ public int compare(int[] o1, int[] o2) {
+ if (o1[0] != o2[0]) {
+ return Integer.compare(o1[0],o2[0]);
+ } else {
+ return Integer.compare(o1[0],o2[0]);
+ }
+ }
+ });
+
+ int count = 1;
+ for (int i = 1; i < points.length; i++) {
+ if (points[i][0] > points[i - 1][1]) {
+ count++;
+ } else {
+ points[i][1] = Math.min(points[i][1],points[i - 1][1]);
+ }
+ }
+ return count;
+ }
+}
+```
Python:
@@ -153,4 +178,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0455.\345\210\206\345\217\221\351\245\274\345\271\262.md" "b/problems/0455.\345\210\206\345\217\221\351\245\274\345\271\262.md"
index ca3eba7467..f57a7fa64a 100644
--- "a/problems/0455.\345\210\206\345\217\221\351\245\274\345\271\262.md"
+++ "b/problems/0455.\345\210\206\345\217\221\351\245\274\345\271\262.md"
@@ -30,7 +30,7 @@
你有两个孩子和三块小饼干,2个孩子的胃口值分别是1,2。
你拥有的饼干数量和尺寸都足以让所有孩子满足。
所以你应该输出2.
-
+
提示:
* 1 <= g.length <= 3 * 10^4
@@ -115,7 +115,23 @@ public:
Java:
-
+```java
+class Solution {
+ public int findContentChildren(int[] g, int[] s) {
+ Arrays.sort(g);
+ Arrays.sort(s);
+ int start = 0;
+ int count = 0;
+ for (int i = 0; i < s.length && start < g.length; i++) {
+ if (s[i] >= g[start]) {
+ start++;
+ count++;
+ }
+ }
+ return count;
+ }
+}
+```
Python:
@@ -129,4 +145,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0491.\351\200\222\345\242\236\345\255\220\345\272\217\345\210\227.md" "b/problems/0491.\351\200\222\345\242\236\345\255\220\345\272\217\345\210\227.md"
index 5deec0eeff..5e36d6bed4 100644
--- "a/problems/0491.\351\200\222\345\242\236\345\255\220\345\272\217\345\210\227.md"
+++ "b/problems/0491.\351\200\222\345\242\236\345\255\220\345\272\217\345\210\227.md"
@@ -200,6 +200,32 @@ public:
Java:
+```java
+class Solution {
+ private List path = new ArrayList<>();
+ private List> res = new ArrayList<>();
+ public List> findSubsequences(int[] nums) {
+ backtracking(nums,0);
+ return res;
+ }
+
+ private void backtracking (int[] nums, int start) {
+ if (path.size() > 1) {
+ res.add(new ArrayList<>(path));
+ }
+
+ int[] used = new int[201];
+ for (int i = start; i < nums.length; i++) {
+ if (!path.isEmpty() && nums[i] < path.get(path.size() - 1) ||
+ (used[nums[i] + 100] == 1)) continue;
+ used[nums[i] + 100] = 1;
+ path.add(nums[i]);
+ backtracking(nums, i + 1);
+ path.remove(path.size() - 1);
+ }
+ }
+}
+```
Python:
@@ -214,4 +240,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0494.\347\233\256\346\240\207\345\222\214.md" "b/problems/0494.\347\233\256\346\240\207\345\222\214.md"
index 2fb2a5eb1c..1782c88c27 100644
--- "a/problems/0494.\347\233\256\346\240\207\345\222\214.md"
+++ "b/problems/0494.\347\233\256\346\240\207\345\222\214.md"
@@ -241,7 +241,24 @@ dp[j] += dp[j - nums[i]];
Java:
-
+```java
+class Solution {
+ public int findTargetSumWays(int[] nums, int target) {
+ int sum = 0;
+ for (int i = 0; i < nums.length; i++) sum += nums[i];
+ if ((target + sum) % 2 != 0) return 0;
+ int size = (target + sum) / 2;
+ int[] dp = new int[size + 1];
+ dp[0] = 1;
+ for (int i = 0; i < nums.length; i++) {
+ for (int j = size; j >= nums[i]; j--) {
+ dp[j] += dp[j - nums[i]];
+ }
+ }
+ return dp[size];
+ }
+}
+```
Python:
@@ -255,4 +272,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0501.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\344\274\227\346\225\260.md" "b/problems/0501.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\344\274\227\346\225\260.md"
index 0e8c0d0e8b..385ce2f1b0 100644
--- "a/problems/0501.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\344\274\227\346\225\260.md"
+++ "b/problems/0501.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\344\274\227\346\225\260.md"
@@ -345,6 +345,53 @@ public:
Java:
+```Java
+class Solution {
+ ArrayList resList;
+ int maxCount;
+ int count;
+ TreeNode pre;
+
+ public int[] findMode(TreeNode root) {
+ resList = new ArrayList<>();
+ maxCount = 0;
+ count = 0;
+ pre = null;
+ findMode1(root);
+ int[] res = new int[resList.size()];
+ for (int i = 0; i < resList.size(); i++) {
+ res[i] = resList.get(i);
+ }
+ return res;
+ }
+
+ public void findMode1(TreeNode root) {
+ if (root == null) {
+ return;
+ }
+ findMode1(root.left);
+
+ int rootValue = root.val;
+ // 计数
+ if (pre == null || rootValue != pre.val) {
+ count = 1;
+ } else {
+ count++;
+ }
+ // 更新结果以及maxCount
+ if (count > maxCount) {
+ resList.clear();
+ resList.add(rootValue);
+ maxCount = count;
+ } else if (count == maxCount) {
+ resList.add(rootValue);
+ }
+ pre = root;
+
+ findMode1(root.right);
+ }
+}
+```
Python:
@@ -358,4 +405,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0513.\346\211\276\346\240\221\345\267\246\344\270\213\350\247\222\347\232\204\345\200\274.md" "b/problems/0513.\346\211\276\346\240\221\345\267\246\344\270\213\350\247\222\347\232\204\345\200\274.md"
index 19c870c367..ac21dc6881 100644
--- "a/problems/0513.\346\211\276\346\240\221\345\267\246\344\270\213\350\247\222\347\232\204\345\200\274.md"
+++ "b/problems/0513.\346\211\276\346\240\221\345\267\246\344\270\213\350\247\222\347\232\204\345\200\274.md"
@@ -218,6 +218,60 @@ public:
Java:
+```java
+// 递归法
+class Solution {
+ private int Deep = -1;
+ private int value = 0;
+ public int findBottomLeftValue(TreeNode root) {
+ value = root.val;
+ findLeftValue(root,0);
+ return value;
+ }
+
+ private void findLeftValue (TreeNode root,int deep) {
+ if (root == null) return;
+ if (root.left == null && root.right == null) {
+ if (deep > Deep) {
+ value = root.val;
+ Deep = deep;
+ }
+ }
+ if (root.left != null) findLeftValue(root.left,deep + 1);
+ if (root.right != null) findLeftValue(root.right,deep + 1);
+ }
+}
+```
+
+```java
+//迭代法
+class Solution {
+
+ public int findBottomLeftValue(TreeNode root) {
+ Queue queue = new LinkedList<>();
+ queue.offer(root);
+ int res = 0;
+ while (!queue.isEmpty()) {
+ int size = queue.size();
+ for (int i = 0; i < size; i++) {
+ TreeNode poll = queue.poll();
+ if (i == 0) {
+ res = poll.val;
+ }
+ if (poll.left != null) {
+ queue.offer(poll.left);
+ }
+ if (poll.right != null) {
+ queue.offer(poll.right);
+ }
+ }
+ }
+ return res;
+ }
+}
+```
+
+
Python:
@@ -231,4 +285,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md" "b/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md"
index 813e75f589..d7acef9975 100644
--- "a/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md"
+++ "b/problems/0516.\346\234\200\351\225\277\345\233\236\346\226\207\345\255\220\345\272\217\345\210\227.md"
@@ -148,6 +148,25 @@ public:
Java:
+```java
+public class Solution {
+ public int longestPalindromeSubseq(String s) {
+ int len = s.length();
+ int[][] dp = new int[len + 1][len + 1];
+ for (int i = len - 1; i >= 0; i--) { // 从后往前遍历 保证情况不漏
+ dp[i][i] = 1; // 初始化
+ for (int j = i + 1; j < len; j++) {
+ if (s.charAt(i) == s.charAt(j)) {
+ dp[i][j] = dp[i + 1][j - 1] + 2;
+ } else {
+ dp[i][j] = Math.max(dp[i + 1][j], Math.max(dp[i][j], dp[i][j - 1]));
+ }
+ }
+ }
+ return dp[0][len - 1];
+ }
+}
+```
Python:
diff --git "a/problems/0530.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\345\260\217\347\273\235\345\257\271\345\267\256.md" "b/problems/0530.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\345\260\217\347\273\235\345\257\271\345\267\256.md"
index d5abc692db..903ebf78cc 100644
--- "a/problems/0530.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\345\260\217\347\273\235\345\257\271\345\267\256.md"
+++ "b/problems/0530.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\347\232\204\346\234\200\345\260\217\347\273\235\345\257\271\345\267\256.md"
@@ -152,6 +152,29 @@ public:
Java:
+```Java
+class Solution {
+ TreeNode pre;// 记录上一个遍历的结点
+ int result = Integer.MAX_VALUE;
+ public int getMinimumDifference(TreeNode root) {
+ if (root == null) {
+ return result;
+ }
+ // 左
+ int left = getMinimumDifference(root.left);
+
+ // 中
+ if (pre != null) {
+ result = Math.min(left, root.val - pre.val);
+ }
+ pre = root;
+ // 右
+ int right = getMinimumDifference(root.right);
+ result = Math.min(right, result);
+ return result;
+ }
+}
+```
Python:
@@ -165,4 +188,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0538.\346\212\212\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\350\275\254\346\215\242\344\270\272\347\264\257\345\212\240\346\240\221.md" "b/problems/0538.\346\212\212\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\350\275\254\346\215\242\344\270\272\347\264\257\345\212\240\346\240\221.md"
index a5f4c43c0d..209c989b67 100644
--- "a/problems/0538.\346\212\212\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\350\275\254\346\215\242\344\270\272\347\264\257\345\212\240\346\240\221.md"
+++ "b/problems/0538.\346\212\212\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\350\275\254\346\215\242\344\270\272\347\264\257\345\212\240\346\240\221.md"
@@ -173,7 +173,27 @@ public:
Java:
+```Java
+class Solution {
+ int sum;
+ public TreeNode convertBST(TreeNode root) {
+ sum = 0;
+ convertBST1(root);
+ return root;
+ }
+ // 按右中左顺序遍历,累加即可
+ public void convertBST1(TreeNode root) {
+ if (root == null) {
+ return;
+ }
+ convertBST1(root.right);
+ sum += root.val;
+ root.val = sum;
+ convertBST1(root.left);
+ }
+}
+```
Python:
@@ -187,4 +207,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0617.\345\220\210\345\271\266\344\272\214\345\217\211\346\240\221.md" "b/problems/0617.\345\220\210\345\271\266\344\272\214\345\217\211\346\240\221.md"
index adc0703bc3..848454de61 100644
--- "a/problems/0617.\345\220\210\345\271\266\344\272\214\345\217\211\346\240\221.md"
+++ "b/problems/0617.\345\220\210\345\271\266\344\272\214\345\217\211\346\240\221.md"
@@ -257,6 +257,59 @@ public:
Java:
+```Java
+class Solution {
+ // 递归
+ public TreeNode mergeTrees(TreeNode root1, TreeNode root2) {
+ if (root1 == null) return root2;
+ if (root2 == null) return root1;
+
+ TreeNode newRoot = new TreeNode(root1.val + root2.val);
+ newRoot.left = mergeTrees(root1.left,root2.left);
+ newRoot.right = mergeTrees(root1.right,root2.right);
+ return newRoot;
+ }
+}
+```
+
+```Java
+class Solution {
+ // 迭代
+ public TreeNode mergeTrees(TreeNode root1, TreeNode root2) {
+ if (root1 == null) {
+ return root2;
+ }
+ if (root2 == null) {
+ return root1;
+ }
+ Stack stack = new Stack<>();
+ stack.push(root2);
+ stack.push(root1);
+ while (!stack.isEmpty()) {
+ TreeNode node1 = stack.pop();
+ TreeNode node2 = stack.pop();
+ node1.val += node2.val;
+ if (node2.right != null && node1.right != null) {
+ stack.push(node2.right);
+ stack.push(node1.right);
+ } else {
+ if (node1.right == null) {
+ node1.right = node2.right;
+ }
+ }
+ if (node2.left != null && node1.left != null) {
+ stack.push(node2.left);
+ stack.push(node1.left);
+ } else {
+ if (node1.left == null) {
+ node1.left = node2.left;
+ }
+ }
+ }
+ return root1;
+ }
+}
+```
Python:
@@ -270,4 +323,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0654.\346\234\200\345\244\247\344\272\214\345\217\211\346\240\221.md" "b/problems/0654.\346\234\200\345\244\247\344\272\214\345\217\211\346\240\221.md"
index af133e0cdd..905bcbaeb8 100644
--- "a/problems/0654.\346\234\200\345\244\247\344\272\214\345\217\211\346\240\221.md"
+++ "b/problems/0654.\346\234\200\345\244\247\344\272\214\345\217\211\346\240\221.md"
@@ -225,6 +225,35 @@ root->right = traversal(nums, maxValueIndex + 1, right);
Java:
+```Java
+class Solution {
+ public TreeNode constructMaximumBinaryTree(int[] nums) {
+ return constructMaximumBinaryTree1(nums, 0, nums.length);
+ }
+
+ public TreeNode constructMaximumBinaryTree1(int[] nums, int leftIndex, int rightIndex) {
+ if (rightIndex - leftIndex < 1) {// 没有元素了
+ return null;
+ }
+ if (rightIndex - leftIndex == 1) {// 只有一个元素
+ return new TreeNode(nums[leftIndex]);
+ }
+ int maxIndex = leftIndex;// 最大值所在位置
+ int maxVal = nums[maxIndex];// 最大值
+ for (int i = leftIndex + 1; i < rightIndex; i++) {
+ if (nums[i] > maxVal){
+ maxVal = nums[i];
+ maxIndex = i;
+ }
+ }
+ TreeNode root = new TreeNode(maxVal);
+ // 根据maxIndex划分左右子树
+ root.left = constructMaximumBinaryTree1(nums, leftIndex, maxIndex);
+ root.right = constructMaximumBinaryTree1(nums, maxIndex + 1, rightIndex);
+ return root;
+ }
+}
+```
Python:
@@ -238,4 +267,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0669.\344\277\256\345\211\252\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md" "b/problems/0669.\344\277\256\345\211\252\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
index 41f684f4a9..442ea8fb76 100644
--- "a/problems/0669.\344\277\256\345\211\252\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
+++ "b/problems/0669.\344\277\256\345\211\252\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221.md"
@@ -242,7 +242,30 @@ public:
Java:
+```java
+class Solution {
+ public TreeNode trimBST(TreeNode root, int low, int high) {
+ root = trim(root,low,high);
+ return root;
+ }
+
+ private static TreeNode trim(TreeNode root,int low, int high) {
+ if (root == null ) return null;
+ if (root.val < low) {
+ return trim(root.right,low,high);
+ }
+ if (root.val > high) {
+ return trim(root.left,low,high);
+ }
+ root.left = trim(root.left,low,high);
+ root.right = trim(root.right,low,high);
+
+ return root;
+ }
+}
+
+```
Python:
@@ -256,4 +279,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0700.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\220\234\347\264\242.md" "b/problems/0700.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\220\234\347\264\242.md"
index 5c1cdfdf54..277ef681f1 100644
--- "a/problems/0700.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\220\234\347\264\242.md"
+++ "b/problems/0700.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\220\234\347\264\242.md"
@@ -140,12 +140,99 @@ public:
## 其他语言版本
-
Java:
+```Java
+class Solution {
+ // 递归,普通二叉树
+ public TreeNode searchBST(TreeNode root, int val) {
+ if (root == null || root.val == val) {
+ return root;
+ }
+ TreeNode left = searchBST(root.left, val);
+ if (left != null) {
+ return left;
+ }
+ return searchBST(root.right, val);
+ }
+}
+
+class Solution {
+ // 递归,利用二叉搜索树特点,优化
+ public TreeNode searchBST(TreeNode root, int val) {
+ if (root == null || root.val == val) {
+ return root;
+ }
+ if (val < root.val) {
+ return searchBST(root.left, val);
+ } else {
+ return searchBST(root.right, val);
+ }
+ }
+}
+
+class Solution {
+ // 迭代,普通二叉树
+ public TreeNode searchBST(TreeNode root, int val) {
+ if (root == null || root.val == val) {
+ return root;
+ }
+ Stack stack = new Stack<>();
+ stack.push(root);
+ while (!stack.isEmpty()) {
+ TreeNode pop = stack.pop();
+ if (pop.val == val) {
+ return pop;
+ }
+ if (pop.right != null) {
+ stack.push(pop.right);
+ }
+ if (pop.left != null) {
+ stack.push(pop.left);
+ }
+ }
+ return null;
+ }
+}
+
+class Solution {
+ // 迭代,利用二叉搜索树特点,优化,可以不需要栈
+ public TreeNode searchBST(TreeNode root, int val) {
+ while (root != null)
+ if (val < root.val) root = root.left;
+ else if (val > root.val) root = root.right;
+ else return root;
+ return root;
+ }
+}
+```
Python:
+递归法:
+
+```python
+class Solution:
+ def searchBST(self, root: TreeNode, val: int) -> TreeNode:
+ if root is None:
+ return None
+ if val < root.val: return self.searchBST(root.left, val)
+ elif val > root.val: return self.searchBST(root.right, val)
+ else: return root
+```
+
+迭代法:
+
+```python
+class Solution:
+ def searchBST(self, root: TreeNode, val: int) -> TreeNode:
+ while root is not None:
+ if val < root.val: root = root.left
+ elif val > root.val: root = root.right
+ else: return root
+ return root
+```
+
Go:
@@ -156,4 +243,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0701.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\217\222\345\205\245\346\223\215\344\275\234.md" "b/problems/0701.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\217\222\345\205\245\346\223\215\344\275\234.md"
index 760509d9cc..ee690d04c5 100644
--- "a/problems/0701.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\217\222\345\205\245\346\223\215\344\275\234.md"
+++ "b/problems/0701.\344\272\214\345\217\211\346\220\234\347\264\242\346\240\221\344\270\255\347\232\204\346\217\222\345\205\245\346\223\215\344\275\234.md"
@@ -16,7 +16,7 @@
注意,可能存在多种有效的插入方式,只要树在插入后仍保持为二叉搜索树即可。 你可以返回任意有效的结果。
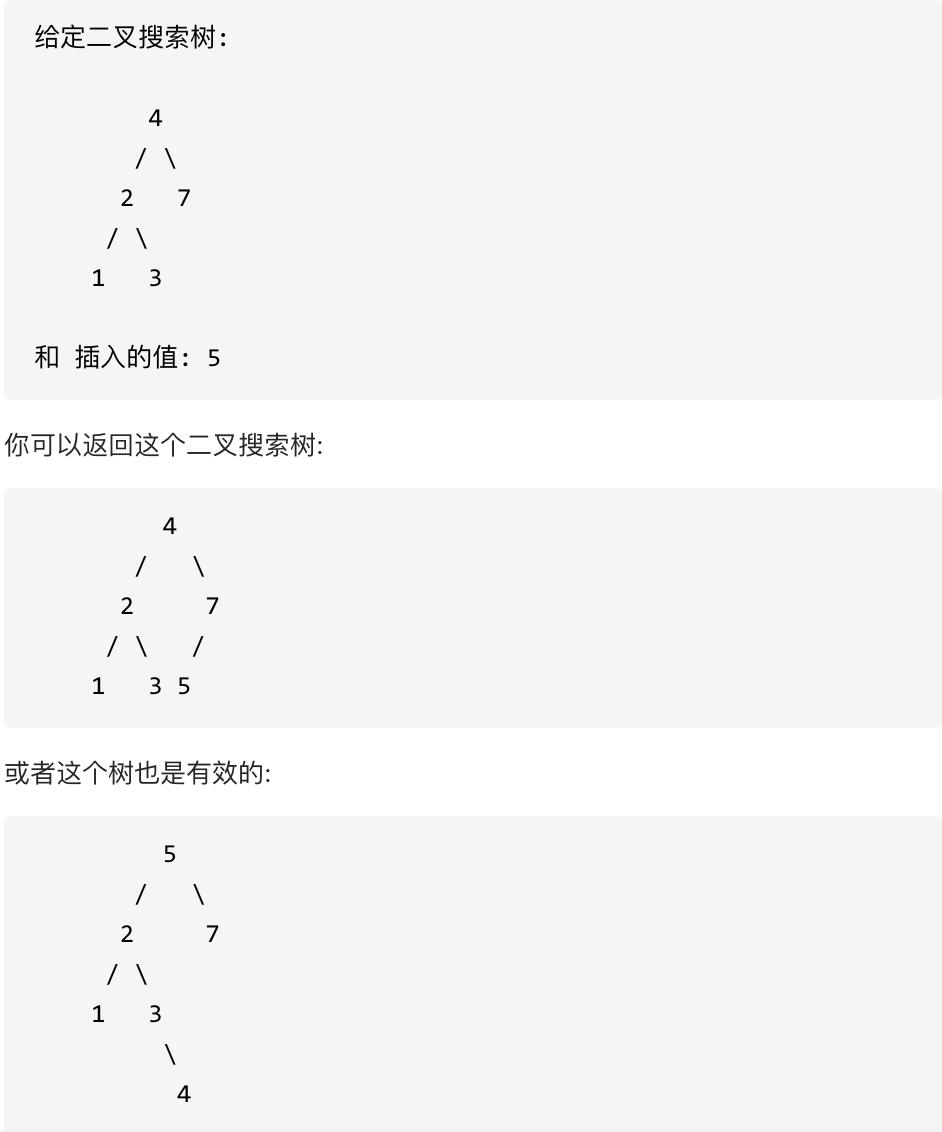
-
+
提示:
* 给定的树上的节点数介于 0 和 10^4 之间
@@ -206,12 +206,69 @@ public:
## 其他语言版本
-
Java:
+```java
+class Solution {
+ public TreeNode insertIntoBST(TreeNode root, int val) {
+ if (root == null) return new TreeNode(val);
+ TreeNode newRoot = root;
+ TreeNode pre = root;
+ while (root != null) {
+ pre = root;
+ if (root.val > val) {
+ root = root.left;
+ } else if (root.val < val) {
+ root = root.right;
+ }
+ }
+ if (pre.val > val) {
+ pre.left = new TreeNode(val);
+ } else {
+ pre.right = new TreeNode(val);
+ }
+
+ return newRoot;
+ }
+}
+```
+
+递归法
+```java
+class Solution {
+ public TreeNode insertIntoBST(TreeNode root, int val) {
+ return buildTree(root, val);
+ }
+
+ public TreeNode buildTree(TreeNode root, int val){
+ if (root == null) // 如果当前节点为空,也就意味着val找到了合适的位置,此时创建节点直接返回。
+ return new TreeNode(val);
+ if (root.val < val){
+ root.right = buildTree(root.right, val); // 递归创建右子树
+ }else if (root.val > val){
+ root.left = buildTree(root.left, val); // 递归创建左子树
+ }
+ return root;
+ }
+}
+```
Python:
+递归法
+
+```python
+class Solution:
+ def insertIntoBST(self, root: TreeNode, val: int) -> TreeNode:
+ if root is None:
+ return TreeNode(val) # 如果当前节点为空,也就意味着val找到了合适的位置,此时创建节点直接返回。
+ if root.val < val:
+ root.right = self.insertIntoBST(root.right, val) # 递归创建右子树
+ if root.val > val:
+ root.left = self.insertIntoBST(root.left, val) # 递归创建左子树
+ return root
+```
+
Go:
@@ -222,4 +279,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0704.\344\272\214\345\210\206\346\237\245\346\211\276.md" "b/problems/0704.\344\272\214\345\210\206\346\237\245\346\211\276.md"
index bb013d9538..c7207364b2 100644
--- "a/problems/0704.\344\272\214\345\210\206\346\237\245\346\211\276.md"
+++ "b/problems/0704.\344\272\214\345\210\206\346\237\245\346\211\276.md"
@@ -23,7 +23,7 @@
输入: nums = [-1,0,3,5,9,12], target = 2
输出: -1
解释: 2 不存在 nums 中因此返回 -1
-
+
提示:
* 你可以假设 nums 中的所有元素是不重复的。
@@ -146,11 +146,50 @@ public:
## 其他语言版本
-
Java:
+(版本一)左闭右闭区间
+
+```java
+class Solution {
+ public int search(int[] nums, int target) {
+ int left = 0, right = nums.length - 1;
+ while (left <= right) {
+ int mid = left + ((right - left) >> 1);
+ if (nums[mid] == target)
+ return mid;
+ else if (nums[mid] < target)
+ left = mid + 1;
+ else if (nums[mid] > target)
+ right = mid - 1;
+ }
+ return -1;
+ }
+}
+```
+
+(版本二)左闭右开区间
+
+```java
+class Solution {
+ public int search(int[] nums, int target) {
+ int left = 0, right = nums.length;
+ while (left < right) {
+ int mid = left + ((right - left) >> 1);
+ if (nums[mid] == target)
+ return mid;
+ else if (nums[mid] < target)
+ left = mid + 1;
+ else if (nums[mid] > target)
+ right = mid;
+ }
+ return -1;
+ }
+}
+```
Python:
+
```python3
class Solution:
def search(self, nums: List[int], target: int) -> int:
@@ -178,4 +217,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0714.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272\345\220\253\346\211\213\347\273\255\350\264\271.md" "b/problems/0714.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272\345\220\253\346\211\213\347\273\255\350\264\271.md"
index 92697a6413..86954c1459 100644
--- "a/problems/0714.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272\345\220\253\346\211\213\347\273\255\350\264\271.md"
+++ "b/problems/0714.\344\271\260\345\215\226\350\202\241\347\245\250\347\232\204\346\234\200\344\275\263\346\227\266\346\234\272\345\220\253\346\211\213\347\273\255\350\264\271.md"
@@ -156,7 +156,23 @@ public:
Java:
-
+```java
+class Solution {
+ public int maxProfit(int[] prices, int fee) {
+ int buy = prices[0] + fee;
+ int sum = 0;
+ for (int p : prices) {
+ if (p + fee < buy) {
+ buy = p + fee;
+ } else if (p > buy){
+ sum += p - buy;
+ buy = p;
+ }
+ }
+ return sum;
+ }
+}
+```
Python:
diff --git "a/problems/0738.\345\215\225\350\260\203\351\200\222\345\242\236\347\232\204\346\225\260\345\255\227.md" "b/problems/0738.\345\215\225\350\260\203\351\200\222\345\242\236\347\232\204\346\225\260\345\255\227.md"
index d423d4d646..dc136028a7 100644
--- "a/problems/0738.\345\215\225\350\260\203\351\200\222\345\242\236\347\232\204\346\225\260\345\255\227.md"
+++ "b/problems/0738.\345\215\225\350\260\203\351\200\222\345\242\236\347\232\204\346\225\260\345\255\227.md"
@@ -125,6 +125,24 @@ public:
Java:
+```java
+class Solution {
+ public int monotoneIncreasingDigits(int N) {
+ String[] strings = (N + "").split("");
+ int start = strings.length;
+ for (int i = strings.length - 1; i > 0; i--) {
+ if (Integer.parseInt(strings[i]) < Integer.parseInt(strings[i - 1])) {
+ strings[i - 1] = (Integer.parseInt(strings[i - 1]) - 1) + "";
+ start = i;
+ }
+ }
+ for (int i = start; i < strings.length; i++) {
+ strings[i] = "9";
+ }
+ return Integer.parseInt(String.join("",strings));
+ }
+}
+```
Python:
@@ -139,4 +157,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0763.\345\210\222\345\210\206\345\255\227\346\257\215\345\214\272\351\227\264.md" "b/problems/0763.\345\210\222\345\210\206\345\255\227\346\257\215\345\214\272\351\227\264.md"
index 1b93edf710..c147428080 100644
--- "a/problems/0763.\345\210\222\345\210\206\345\255\227\346\257\215\345\214\272\351\227\264.md"
+++ "b/problems/0763.\345\210\222\345\210\206\345\255\227\346\257\215\345\214\272\351\227\264.md"
@@ -84,7 +84,28 @@ public:
Java:
-
+```java
+class Solution {
+ public List partitionLabels(String S) {
+ List list = new LinkedList<>();
+ int[] edge = new int[123];
+ char[] chars = S.toCharArray();
+ for (int i = 0; i < chars.length; i++) {
+ edge[chars[i] - 0] = i;
+ }
+ int idx = 0;
+ int last = -1;
+ for (int i = 0; i < chars.length; i++) {
+ idx = Math.max(idx,edge[chars[i] - 0]);
+ if (i == idx) {
+ list.add(i - last);
+ last = i;
+ }
+ }
+ return list;
+ }
+}
+```
Python:
diff --git "a/problems/0860.\346\237\240\346\252\254\346\260\264\346\211\276\351\233\266.md" "b/problems/0860.\346\237\240\346\252\254\346\260\264\346\211\276\351\233\266.md"
index c718b0cd5b..bf8a377641 100644
--- "a/problems/0860.\346\237\240\346\252\254\346\260\264\346\211\276\351\233\266.md"
+++ "b/problems/0860.\346\237\240\346\252\254\346\260\264\346\211\276\351\233\266.md"
@@ -127,7 +127,33 @@ public:
Java:
-
+```java
+class Solution {
+ public boolean lemonadeChange(int[] bills) {
+ int cash_5 = 0;
+ int cash_10 = 0;
+
+ for (int i = 0; i < bills.length; i++) {
+ if (bills[i] == 5) {
+ cash_5++;
+ } else if (bills[i] == 10) {
+ cash_5--;
+ cash_10++;
+ } else if (bills[i] == 20) {
+ if (cash_10 > 0) {
+ cash_10--;
+ cash_5--;
+ } else {
+ cash_5 -= 3;
+ }
+ }
+ if (cash_5 < 0 || cash_10 < 0) return false;
+ }
+
+ return true;
+ }
+}
+```
Python:
@@ -141,4 +167,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/0968.\347\233\221\346\216\247\344\272\214\345\217\211\346\240\221.md" "b/problems/0968.\347\233\221\346\216\247\344\272\214\345\217\211\346\240\221.md"
index ab2bd2e55e..e541ba139f 100644
--- "a/problems/0968.\347\233\221\346\216\247\344\272\214\345\217\211\346\240\221.md"
+++ "b/problems/0968.\347\233\221\346\216\247\344\272\214\345\217\211\346\240\221.md"
@@ -316,6 +316,33 @@ public:
Java:
+```java
+class Solution {
+ private int count = 0;
+ public int minCameraCover(TreeNode root) {
+ if (trval(root) == 0) count++;
+ return count;
+ }
+
+ private int trval(TreeNode root) {
+ if (root == null) return -1;
+
+ int left = trval(root.left);
+ int right = trval(root.right);
+
+ if (left == 0 || right == 0) {
+ count++;
+ return 2;
+ }
+
+ if (left == 2 || right == 2) {
+ return 1;
+ }
+
+ return 0;
+ }
+}
+```
Python:
@@ -330,4 +357,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/1005.K\346\254\241\345\217\226\345\217\215\345\220\216\346\234\200\345\244\247\345\214\226\347\232\204\346\225\260\347\273\204\345\222\214.md" "b/problems/1005.K\346\254\241\345\217\226\345\217\215\345\220\216\346\234\200\345\244\247\345\214\226\347\232\204\346\225\260\347\273\204\345\222\214.md"
index b2073e1434..1653201e8f 100644
--- "a/problems/1005.K\346\254\241\345\217\226\345\217\215\345\220\216\346\234\200\345\244\247\345\214\226\347\232\204\346\225\260\347\273\204\345\222\214.md"
+++ "b/problems/1005.K\346\254\241\345\217\226\345\217\215\345\220\216\346\234\200\345\244\247\345\214\226\347\232\204\346\225\260\347\273\204\345\222\214.md"
@@ -29,7 +29,7 @@
输入:A = [2,-3,-1,5,-4], K = 2
输出:13
解释:选择索引 (1, 4) ,然后 A 变为 [2,3,-1,5,4]。
-
+
提示:
* 1 <= A.length <= 10000
@@ -99,7 +99,29 @@ public:
Java:
+```java
+class Solution {
+ public int largestSumAfterKNegations(int[] A, int K) {
+ if (A.length == 1) return A[0];
+ Arrays.sort(A);
+ int sum = 0;
+ int idx = 0;
+ for (int i = 0; i < K; i++) {
+ if (i < A.length - 1 && A[idx] < 0) {
+ A[idx] = -A[idx];
+ if (A[idx] >= Math.abs(A[idx + 1])) idx++;
+ continue;
+ }
+ A[idx] = -A[idx];
+ }
+ for (int i = 0; i < A.length; i++) {
+ sum += A[i];
+ }
+ return sum;
+ }
+}
+```
Python:
@@ -113,4 +135,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file
diff --git "a/problems/1143.\346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227.md" "b/problems/1143.\346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227.md"
index 0664b0d970..19f4cc728d 100644
--- "a/problems/1143.\346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227.md"
+++ "b/problems/1143.\346\234\200\351\225\277\345\205\254\345\205\261\345\255\220\345\272\217\345\210\227.md"
@@ -31,7 +31,7 @@
输入:text1 = "abc", text2 = "def"
输出:0
解释:两个字符串没有公共子序列,返回 0。
-
+
提示:
* 1 <= text1.length <= 1000
* 1 <= text2.length <= 1000
@@ -126,12 +126,44 @@ public:
## 其他语言版本
-
Java:
+```java
+class Solution {
+ public int longestCommonSubsequence(String text1, String text2) {
+ int[][] dp = new int[text1.length() + 1][text2.length() + 1]; // 先对dp数组做初始化操作
+ for (int i = 1 ; i <= text1.length() ; i++) {
+ char char1 = text1.charAt(i - 1);
+ for (int j = 1; j <= text2.length(); j++) {
+ char char2 = text2.charAt(j - 1);
+ if (char1 == char2) { // 开始列出状态转移方程
+ dp[i][j] = dp[i - 1][j - 1] + 1;
+ } else {
+ dp[i][j] = Math.max(dp[i - 1][j], dp[i][j - 1]);
+ }
+ }
+ }
+ return dp[text1.length()][text2.length()];
+ }
+}
+```
Python:
+```python
+class Solution:
+ def longestCommonSubsequence(self, text1: str, text2: str) -> int:
+ len1, len2 = len(text1)+1, len(text2)+1
+ dp = [[0 for _ in range(len1)] for _ in range(len2)] # 先对dp数组做初始化操作
+ for i in range(1, len2):
+ for j in range(1, len1): # 开始列出状态转移方程
+ if text1[j-1] == text2[i-1]:
+ dp[i][j] = dp[i-1][j-1]+1
+ else:
+ dp[i][j] = max(dp[i-1][j], dp[i][j-1])
+ return dp[-1][-1]
+```
+
Go:
@@ -142,4 +174,4 @@ Go:
* 作者微信:[程序员Carl](https://mp.weixin.qq.com/s/b66DFkOp8OOxdZC_xLZxfw)
* B站视频:[代码随想录](https://space.bilibili.com/525438321)
* 知识星球:[代码随想录](https://mp.weixin.qq.com/s/QVF6upVMSbgvZy8lHZS3CQ)
-
+
\ No newline at end of file