|
| 1 | +# TextBee - Android SMS Gateway |
| 2 | + |
| 3 | +A simple SMS gateway that allows users to send SMS messages from a web interface or |
| 4 | +from their application via a REST API. It utilizes android phones as SMS gateways. |
| 5 | + |
| 6 | +- **Technology stack**: React, Next.js, Node.js, NestJs, MongoDB, Android, Java |
| 7 | +- **Status**: MVP in development, not ready for production use yet |
| 8 | +- **Link**: [https://textbee.vernu.dev](https://textbee.vernu.dev/) |
| 9 | + |
| 10 | +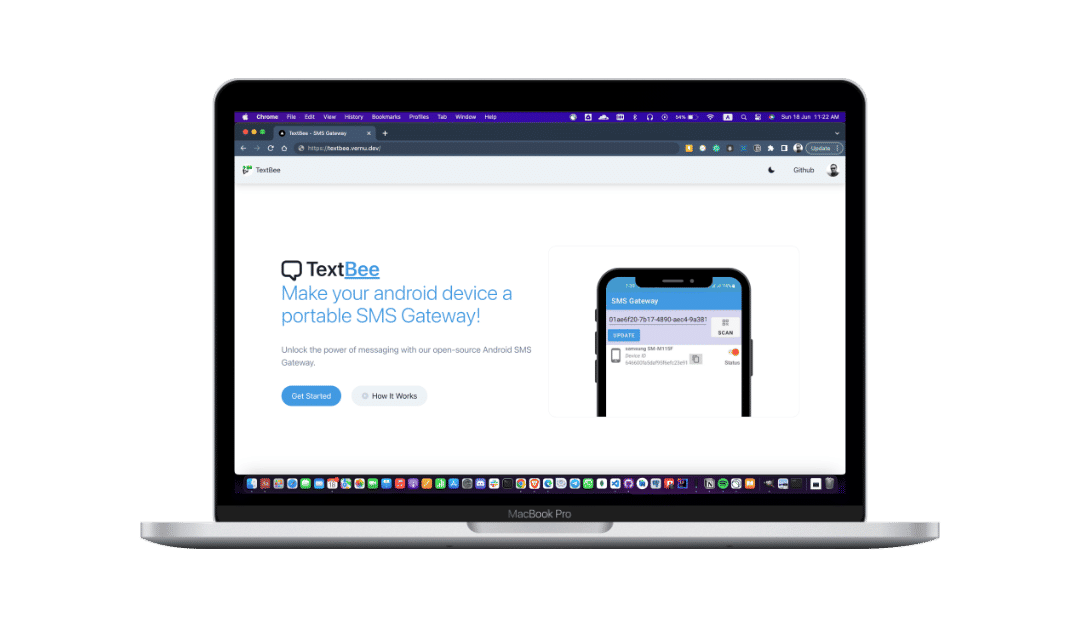 |
| 11 | + |
| 12 | +## Usage |
| 13 | + |
| 14 | +1. Go to [textbee.vernu.dev](https://textbee.vernu.dev) and register or login with your account |
| 15 | +2. Install the app on your android phone from [textbee.vernu.dev/android](https://textbee.vernu.dev/android) |
| 16 | +3. Open the app and grant the permissions for SMS |
| 17 | +4. Go to [textbee.vernu.dev/dashboard](https://textbee.vernu.dev/dashboard) and click register device/ generate API Key |
| 18 | +5. Scan the QR code with the app or enter the API key manually |
| 19 | +6. You are ready to send SMS messages from the dashboard or from your application via the REST API |
| 20 | + |
| 21 | +**Code Snippet**: Few lines of code showing how to send an SMS message via the REST API |
| 22 | + |
| 23 | +```javascript |
| 24 | +const API_KEY = 'YOUR_API_KEY'; |
| 25 | +const DEVICE_ID = 'YOUR_DEVICE_ID'; |
| 26 | + |
| 27 | +await axios.post(`https://api.textbee.vernu.dev/api/v1/devices/${DEVICE_ID}/sendSMS?apiKey=${API_KEY}`, { |
| 28 | + receivers: [ '+251912345678' ], |
| 29 | + smsBody: 'Hello World!', |
| 30 | +}) |
| 31 | + |
| 32 | +``` |
| 33 | + |
| 34 | +## Contributing |
| 35 | + |
| 36 | +Contributions are welcome! |
| 37 | + |
| 38 | +1. Fork the project. |
| 39 | +2. Create a feature or bugfix branch from `main` branch. |
| 40 | +3. Make sure your commit messages and PR comment summaries are descriptive. |
| 41 | +4. Create a pull request to the `main` branch. |
0 commit comments