You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
JavaScript was a language created for the web that is why its popularity has sky rocked because as the popularity of the web has increased so has the popularity of JavaScript. Since most developers know JavaScript we have taken it out of the web to build mobile applications (React Native), servers (Node JS) and desktop applications (electron). It is important to gain a deep understanding of it if you become a professional dev but it is very easy to get started. Let's look at its original application, making dynamic websites through DOM manipulations and making HTTP calls to get data from websites/servers.
DOM
It is a JavaScript API for accessing the website to alter the HTML and example JavaScript that would alter HTML
document.title='hello world'
This would change the text inside <title></title> tags. You can imagine how we could create dynamic websites now, for example, we set the title of the page based on the user's name. The DOM allows us to think of the HTML in a website in a programmatic sense.
Here is how JavaScript would view a webpage.
We won't spend much time learning the DOM because you don't work with it much in your day job because of libraries that have built abstractions over it so you don't directly write JavaScript that manipulates the DOM like what we did above. Example DOM manipulations
window.onload=function(){alert('hello world')}// When the webpage loads we create a popup with the text hello world// Get the first paragraph element and varparagraphs=document.getElementsByTagName("P");alert(paragraphs[0].nodeName);// loads a popup with `P`// Sets the text for an elementdocument.getElementById("someId").innerHTML="Hello World!";// Appending an element to the DOMvarnode=document.createElement("P");// Create a <li> nodevartextnode=document.createTextNode("Water");// Create a text nodenode.appendChild(textnode);// Append the text to <li>document.getElementById("container").appendChild(node);// Append to the container element // When you click a button with id btn it will write to the console the current datedocument.getElementById("btn").addEventListener("click",function(){console.log((newDate).toLocalDateString())});
Making a reactive webpage
<!DOCTYPE html><html><head><title>Parcel Sandbox</title><metacharset="UTF-8" />
</head><body><buttonid="btn">Add some text</button><h2>Some text will go here</h2><ulid="list"></ul></body><script>functionaddSomeText(){varnode=document.createElement("LI");vartextnode=document.createTextNode("some text");node.appendChild(textnode);document.getElementById("list").appendChild(node);}window.onload=function(){document.getElementById("btn").addEventListener('click',addSomeText)};</script></html>
Take away
Although you won't use this much or at all (I haven't used this sort of code for over a year) it is important to appreciate what is going on behind the scenes with the frameworks you are using. At the end of the day, all the frameworks compile down to plain JavaScript consisting of these types of functions. The most important concepts are the DOM and event listeners so you should research them more before a job interview.
JavaScript Syntax
It is a very relaxed language that has a lot of quirks noobling/david-learns#21 that remain because if they changed it would break a lot of websites that have written this kind of code. A major reason why I believe JavaScript has become so popular is the low barrier to learn it. You can just open up your browser console F12 and start writing JavaScript. The issue with that is it has allowed a lot of low-quality content to float around and to make things worse the language changes so fast, new syntax is constantly added for example es5 introduced classes, destructuring, let and const.
The important parts
I find it boring just listing out everything like a dictionary. But somethings you just have to learn before you can do the fun practical stuff.
constnums=[1,2,3,4]nums.map(x=>x+1)// => [2,3,4,5]nums.filter(x=>x<3)// => [1,2]nums.reduce((accumulator,current)=>accumulator+current))// => 10nums.forEach(x=> ...)// loops through each number in arraynums.find(x=>x<3)// => 1nums.findIndex(x=>x<3)// => 0constobj={key1: 'value',key2: 'value'}Object.keys(obj).forEach(key=>obj[key])
Strings
consthello='hello world'`string template ${hello}`hello.length// => 11hello.indexOf('hello')// => 0hello.indexOf('apple')// => -1hello.slice(1,4)// => ellhello.replace('hello','dog')// => dog worldhello.toUpperCase()// => HELLO WORLDhello.toLowerCase()// => hello world' hello world'.trim()// => hello worldhello.split()// => ['hello', 'world']['hello','world'].join()// => 'hello world'// NOTE: most string methods can take regex input instead of string input
Object destructuring
// This is an advanced concept introduced in es6constobj1={k1: 1,k2: 2}constobj2={k3: 3,k4: 4}{...obj1, ...obj2}// => {k1: 1, k2: 2, k3: 3, k4: 4}constobj3={k1: 4}{...obj1, ...obj3}// => {k1: 4, k2: 2}const{k1}=obj1// k1 = 1constarr=[1,2,3,4]const[first,second]=arr// first = 1, second = 2
Async
There are two types of communication synchronous and asynchronous the former you have to wait for the response and the latter you don't wait for the response. The main use case for this is when you want to read or save from an API/Server/Database but you don't want to reload the entire page. JavaScript can be written asynchronously the issue with this is it can make the code hard to understand.
fetch('https://api.somewhere.com').then(function(response){if(response.status!==200){console.log(`error occured ${response.status}`)return;}// Examine the text in the responseresponse.json().then(function(data){console.log(data);});}).catch(function(err){console.log('Fetch Error :-S',err);});console.log('hello world')// code here will execute immediately without waiting for the slow network request.// Using async/await instead of promisestry{constresponse=awaitfetch('https://api.somwhere.com')if(response.status!==200){console.log(`error occured ${response.status}`)}else{const{ data }=awaitresponse.json()// do something with data}}catch(e){console.error(e)}
Perhaps it is a bit easier to read with async/await since it looks synchronous.
Wrap up
That is it you can build a lot of things with just those constructs. Of course, there is much more to JavaScript, but that is what I use daily. I encourage you to go deeper into JavaScript and learn it more in-depth because like it or not it is here to say and will remain the dominant programming language for years to come.
JavaScript was a language created for the web that is why its popularity has sky rocked because as the popularity of the web has increased so has the popularity of JavaScript. Since most developers know JavaScript we have taken it out of the web to build mobile applications (React Native), servers (Node JS) and desktop applications (electron). It is important to gain a deep understanding of it if you become a professional dev but it is very easy to get started. Let's look at its original application, making dynamic websites through DOM manipulations and making HTTP calls to get data from websites/servers.
DOM
It is a JavaScript API for accessing the website to alter the HTML and example JavaScript that would alter HTML
This would change the text inside
<title></title>
tags. You can imagine how we could create dynamic websites now, for example, we set the title of the page based on the user's name. The DOM allows us to think of the HTML in a website in a programmatic sense.Here is how JavaScript would view a webpage.
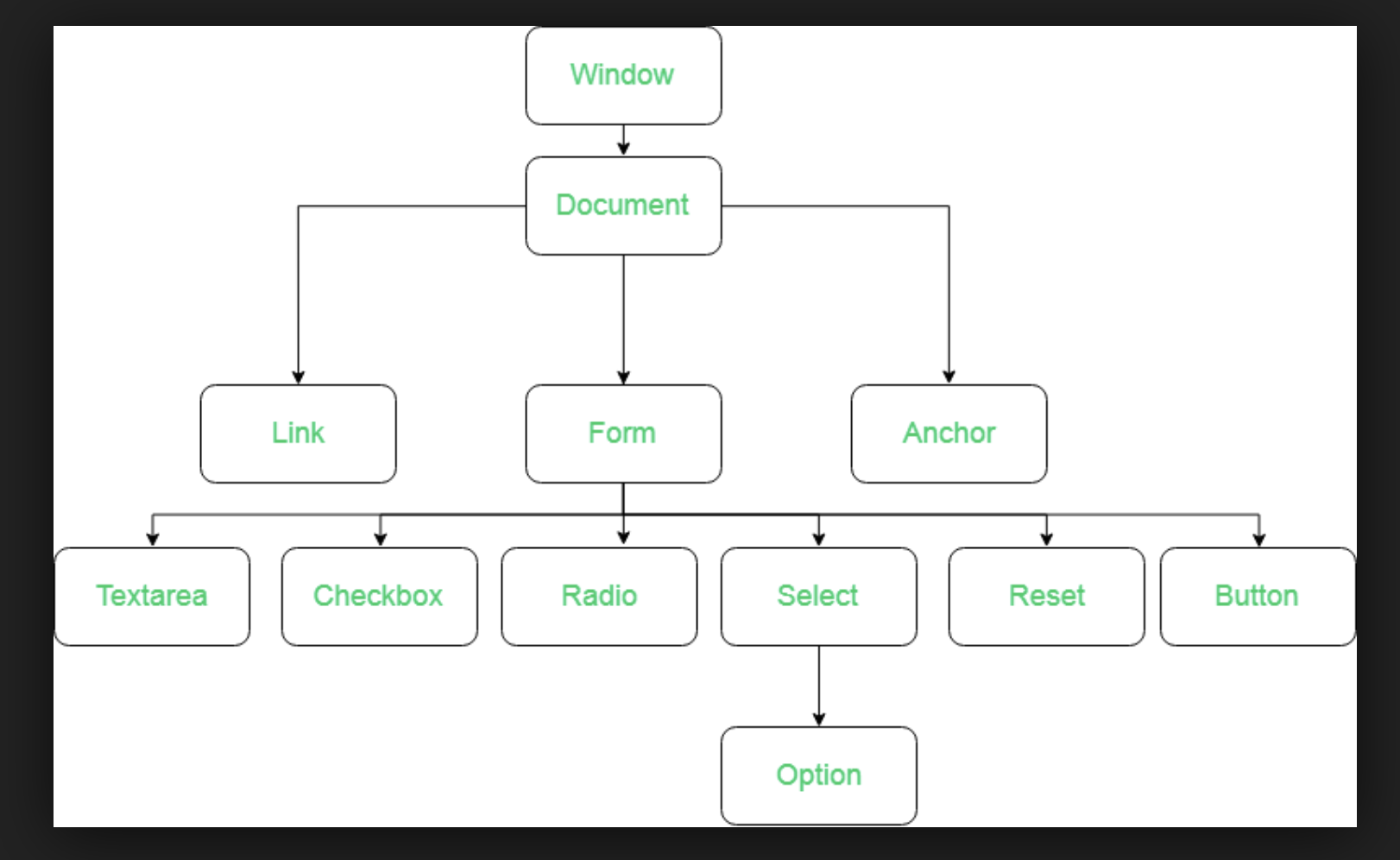
We won't spend much time learning the DOM because you don't work with it much in your day job because of libraries that have built abstractions over it so you don't directly write JavaScript that manipulates the DOM like what we did above. Example DOM manipulations
Making a reactive webpage
Take away
Although you won't use this much or at all (I haven't used this sort of code for over a year) it is important to appreciate what is going on behind the scenes with the frameworks you are using. At the end of the day, all the frameworks compile down to plain JavaScript consisting of these types of functions. The most important concepts are the DOM and event listeners so you should research them more before a job interview.
JavaScript Syntax
It is a very relaxed language that has a lot of quirks noobling/david-learns#21 that remain because if they changed it would break a lot of websites that have written this kind of code. A major reason why I believe JavaScript has become so popular is the low barrier to learn it. You can just open up your browser console
F12
and start writing JavaScript. The issue with that is it has allowed a lot of low-quality content to float around and to make things worse the language changes so fast, new syntax is constantly added for example es5 introduced classes, destructuring, let and const.The important parts
I find it boring just listing out everything like a dictionary. But somethings you just have to learn before you can do the fun practical stuff.
variable definitions
function declarations
loops
conditionals
Data structures
Iteration and manipulation of data structures
Strings
Object destructuring
Async
There are two types of communication synchronous and asynchronous the former you have to wait for the response and the latter you don't wait for the response. The main use case for this is when you want to read or save from an API/Server/Database but you don't want to reload the entire page. JavaScript can be written asynchronously the issue with this is it can make the code hard to understand.
Perhaps it is a bit easier to read with async/await since it looks synchronous.
Wrap up
That is it you can build a lot of things with just those constructs. Of course, there is much more to JavaScript, but that is what I use daily. I encourage you to go deeper into JavaScript and learn it more in-depth because like it or not it is here to say and will remain the dominant programming language for years to come.
Practical Example
Specification
now
Example solution
Go further
There are a lot of issues in our current implementation
Further readings
noobling/david-learns#2
noobling/david-learns#21
🌮 We did it! Let's look at how we can improve what we did by using React
The text was updated successfully, but these errors were encountered: