support for environment variables in path
#8
Labels
documentation
Improvements or additions to documentation
enhancement
New feature or request
wontfix
This will not be worked on
We provide a JupyterHub+JupyterLab solution to access our hpc systems at the Jülich Supercomputing Centre. Depending on the system and project a user requests at login, environment variables like $HOME, $PROJECT and $SCRATCH are set to different paths.
@rcthomas pointed me to the fantastic idea to use `jupyterlab-favorites' to pass these environment variables through to the UI and make them accessible with one click.
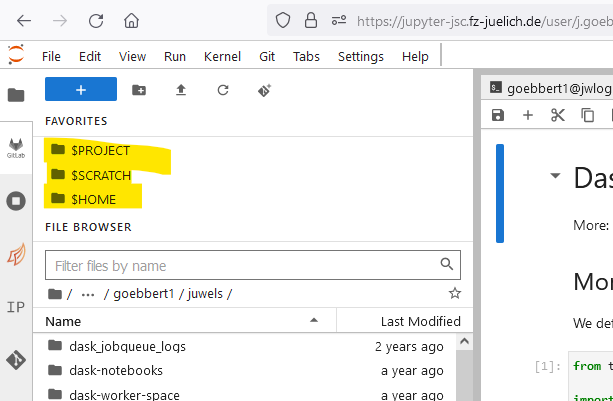
One could say, problem solved :) ... but it has one drawback:
There is no other way to add (the login-specific) $HOME, $PROJECT, $SCRATCH to the favorites than writing/updating the users owned settings file at
$HOME/.jupyter/lab/user-settings/@jlab-enhanced/favorites/favorites.jupyterlab-settings
every time a JupyterLab gets started for a user by JupyterHub.Here is our update-script which ensures, that the users favorites are kept while $HOME, $PROJECT, $SCRATCH is updated.
Update script to add $HOME, $PROJECT, $SCRATCH as favorites to `favorites.jupyterlab-settings`
Describe the solution you'd like
It would be great, if
jupyterlab-favorites
would support environment variables to be added to its settings file in the favorite propertypath
:That would allow us to do the settings once for all users at sys.prefix-level in
<jlab-install-dir>/etc/jupyter/labconfig/default_setting_overrides.json
.(but to my understanding this might need to be supported by the JupyterLab's file bowser first)
Describe alternatives you've considered
<extension_name>/<plugin_name>.jupyterlab-settings
is no option as the favorite-paths are user- and login-specific.overrides.json
in the application’s settings directory is not option as well for the same reason.overrides.json
is not taken from a list of directories, so I cannot add an additional place to checkThe text was updated successfully, but these errors were encountered: