-
-
Notifications
You must be signed in to change notification settings - Fork 97
New issue
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement. We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Overhaul the Tween system #514
Comments
All up for parallel execution! Would love to see that implemented. |
Maybe the array of tweens should be in a singleton? I don't understand how one property of the same object could be tweened from 2 different tween objects from 2 different scenes. Having them all in one place seems like a good way to ensure you arn't accidentally overlapping tweens and keeps them nice and organized. P.S. I'm not sure you need append.advance I think doing destination - start isn't so difficult that we need to make another function to simulate it. |
It's useful for relative movement, i.e. when you don't necessarily know the start and destination points. This way you can tween jumping movements which don't depend on starting height etc. |
but if the default if the default starting point is the current one then you've already found the start. Maybe you could have a start() method that returns it then the destination would be start() + advance |
Here's an idea: do the same thing for animations. You would have play() only require 1 argument (the name of the animation) then once you've created it you can specify custom blend, play speed (I don't see the need for having a from end boolean, can't we just assume that is the case for any negative value?), delay, and perhaps even easetype such that you could interpolate through the animation as you would a tween. |
So, would the following still work then, if you create a temporary Tween every time? I have a counter that flashes whenever it's being increased (tween that interpolates both scale from 1.5 to 1, and modulates color). |
Theoretically, when you interpolate the same thing with 2 Tweens, the one added later takes precedence (AnimationPlayers work this way for example). Or you can just store reference to the Tween and remove/restart it manually. |
This would be an improvement. +1 support! |
This looks very good. I was just thinking if this could benefit from a sort of "chainable" API, which I think could make it very readable. Unfortunately, for this to be really nice we would need to have godotengine/godot#35415 fixed, otherwise you need to put backslashes at the end of every line and would not be able to use comments, but the idea would be something like this: var tween = (TweenBuilder.new()
.begin() # begin() gives you an object with the API to add a tween
.interpolate(object, property, target_value, duration)
# Tween methods give you an object with the API to set properties
.with_ease(ease)
.with_trans(trans)
.then() # .then() is sequential concatenation
.advance(object, property, advance, duration)
.with_delay(delay)
.and_() # and_() is parallel execution wrt latest .then() or .begin()
.interpolate_method(object, method, val1, val2, duration)
# You can make "sub-tweeners" (or whatever you want to call them)
# to have a sequence of tweens to execute in parallel
.and_()
.begin() # Begin "sub-tweener"
.interpolate(...)
.with_this_property(...)
.then()
.follow_method(...)
.with_that_property(...)
.done() # End of "sub-tweener"
# Could have a parameter in .then() or and_() to add delay
# (in theory even negative delays could be made to work I think?)
.then(2.0)
.targeting_property(...)
.done() # Finish chain
)
# Set general tween properties as usual
tween.set_loops(...)
tween.set_speed(...)
tween.set_autostart(...) |
One more suggestion could be supporting custom easing functions (which must return a float from 0 to 1), but yet I can't think of any actual use; maybe when you don't like both of the default functions. |
Describe the project you are working on:
A very big game™, although it's loosely relevant.
Describe the problem or limitation you are having in your project:
Godot's tweens have problems. You need to add them to scene tree, then interpolate desired properties using extremely long method calls and then you need to remember to start them. This is maybe acceptable, but real fun starts when you want to chain multiple tweens. You end up with a yield mess like this
Absolutely horrible. Lots of lines, long lines. All to get this effect
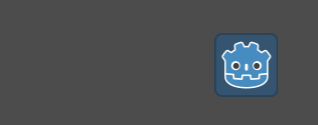
Godot's tweens weren't designed with chaining in mind and from my experience you want to chain them more than not.
Describe the feature / enhancement and how it helps to overcome the problem or limitation:
My inspiration for this proposal was DOTween. It's a very productive implementation of tweens that make you want create animations from code instead of e.g. AnimationPlayer (if it was in Godot).
The aim is to reduce code amount required to make an animation. The most basic thing would be making chaining easier. The Tween commands could just run sequentially instead of simultaneously like now and the parallel execution could be done explicitly. Also requiring to set transition and ease type each time is counter-productive, we should have defaults (probably linear + in/out). Also Tweens could start automatically, e.g. by connecting to SceneTree's idle frame signal.
Another thing inspired by DOTeen are Tweeners. Imagine if
interpolate_property
returned an object that could be used to tweak the command it's created for, so instead ofwe could do
We omit the
initial
argument and by default it uses current value. Also we omit ease/trans and set them only when non-defaults are needed. I'll give more examples later, but the last thing that could be changed is making Tweens Reference instead of Node. They don't have associated editor and don't have interesting inspector properties, so there's no need to keep them in tree. They could just be created on the fly and removed automatically when not needed, similar to SceneTreeTimers.I actually implemented a system like this in GDScript and the above code changes to
It's much easier to create both simple and complex animations and you write only what you need.
Describe how your proposal will work, with code, pseudocode, mockups, and/or diagrams:
Literally this: godot-extended-libraries/godot-next#50
but in C++. The Tweens could get a total overhaul that functionally make them work like my custom class (which is basically GDScript DOTween).
So here's a breakdown of my code.
First, creating Tweens would be dynamic:
var tween = Tween.new()
When you have a Tween, you can adjust it's properties. In my case you can do
Then, to add operations to Tween, you can do
These operations should return Tweener objects (e.g. PropertyTweener etc.) that can be used to tweak the calls further. Calling anything on the Tweener returns the Tweener again, for easy chaining of multiple settings.
For property it would be
Example chained usage:
For callback, you can set the delay and for method you can set ease/trans/delay.
Finally, to allow parallel execution, you can do:
^ these two properties would be tweened simultanously.
Alternatively,
tween.join()
could be a version ofparallel()
that throws an error when the tween doesn't have preceding commands.That sums up the functionality I think (at least from my class). I see that current Tween have more methods, but they could be adopted to the new system too. Also we could have some way to get a list of all running tweens, tweak more properties like per-tween or global default transition/ease type etc. My proposal is more like base for the new Tweens.
If this enhancement will not be used often, can it be worked around with a few lines of script?:
IMO it would be used often. More often that current Tweens, because the new system would be easier.
Is there a reason why this should be core and not an add-on in the asset library?:
The current Tweens are meh, so they should be replaced by this.
The text was updated successfully, but these errors were encountered: