-
-
Notifications
You must be signed in to change notification settings - Fork 5.5k
Commit
This commit does not belong to any branch on this repository, and may belong to a fork outside of the repository.
This PR implements a [Chef registry](https://chef.io/) to manage cookbooks. This package type was a bit complicated because Chef uses RSA signed requests as authentication with the registry. 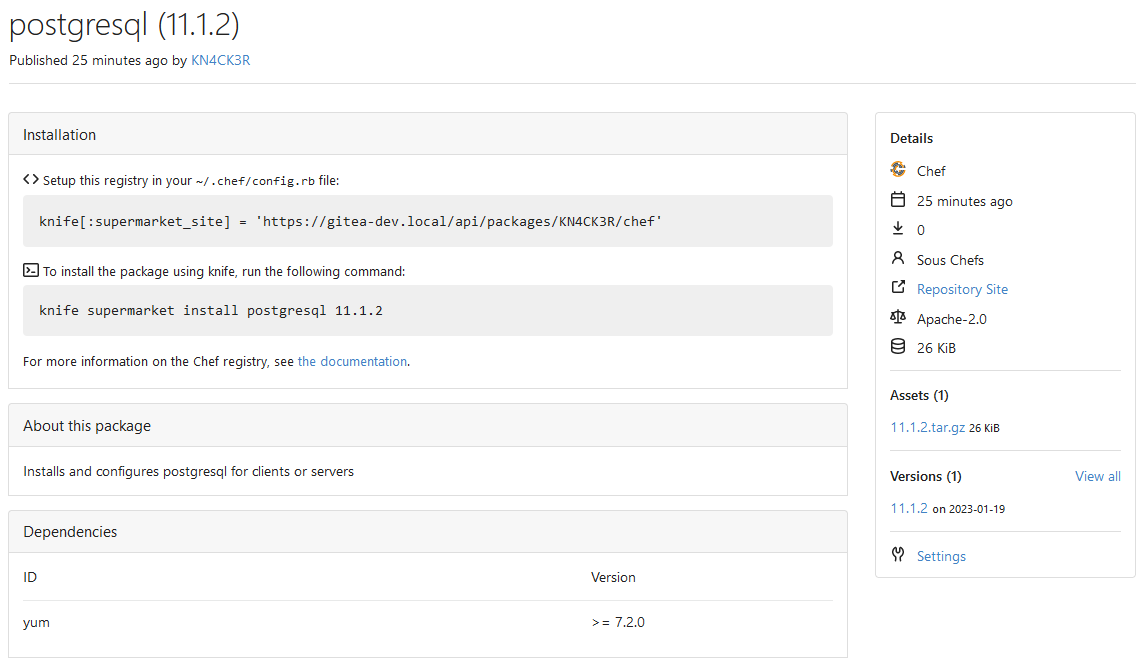 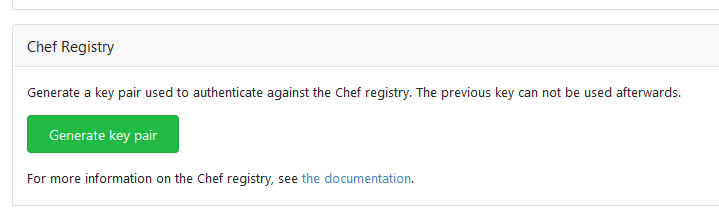 Co-authored-by: Lunny Xiao <xiaolunwen@gmail.com>
- Loading branch information
Showing
31 changed files
with
1,737 additions
and
20 deletions.
There are no files selected for viewing
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,96 @@ | ||
--- | ||
date: "2023-01-20T00:00:00+00:00" | ||
title: "Chef Packages Repository" | ||
slug: "packages/chef" | ||
draft: false | ||
toc: false | ||
menu: | ||
sidebar: | ||
parent: "packages" | ||
name: "Chef" | ||
weight: 5 | ||
identifier: "chef" | ||
--- | ||
|
||
# Chef Packages Repository | ||
|
||
Publish [Chef](https://chef.io/) cookbooks for your user or organization. | ||
|
||
**Table of Contents** | ||
|
||
{{< toc >}} | ||
|
||
## Requirements | ||
|
||
To work with the Chef package registry, you have to use [`knife`](https://docs.chef.io/workstation/knife/). | ||
|
||
## Authentication | ||
|
||
The Chef package registry does not use an username:password authentication but signed requests with a private:public key pair. | ||
Visit the package owner settings page to create the necessary key pair. | ||
Only the public key is stored inside Gitea. if you loose access to the private key you must re-generate the key pair. | ||
[Configure `knife`](https://docs.chef.io/workstation/knife_setup/) to use the downloaded private key with your Gitea username as `client_name`. | ||
|
||
## Configure the package registry | ||
|
||
To [configure `knife`](https://docs.chef.io/workstation/knife_setup/) to use the Gitea package registry add the url to the `~/.chef/config.rb` file. | ||
|
||
``` | ||
knife[:supermarket_site] = 'https://gitea.example.com/api/packages/{owner}/chef' | ||
``` | ||
|
||
| Parameter | Description | | ||
| --------- | ----------- | | ||
| `owner` | The owner of the package. | | ||
|
||
## Publish a package | ||
|
||
To publish a Chef package execute the following command: | ||
|
||
```shell | ||
knife supermarket share {package_name} | ||
``` | ||
|
||
| Parameter | Description | | ||
| -------------- | ----------- | | ||
| `package_name` | The package name. | | ||
|
||
You cannot publish a package if a package of the same name and version already exists. You must delete the existing package first. | ||
|
||
## Install a package | ||
|
||
To install a package from the package registry, execute the following command: | ||
|
||
```shell | ||
knife supermarket install {package_name} | ||
``` | ||
|
||
Optional you can specify the package version: | ||
|
||
```shell | ||
knife supermarket install {package_name} {package_version} | ||
``` | ||
|
||
| Parameter | Description | | ||
| ----------------- | ----------- | | ||
| `package_name` | The package name. | | ||
| `package_version` | The package version. | | ||
|
||
## Delete a package | ||
|
||
If you want to remove a package from the registry, execute the following command: | ||
|
||
```shell | ||
knife supermarket unshare {package_name} | ||
``` | ||
|
||
Optional you can specify the package version: | ||
|
||
```shell | ||
knife supermarket unshare {package_name}/versions/{package_version} | ||
``` | ||
|
||
| Parameter | Description | | ||
| ----------------- | ----------- | | ||
| `package_name` | The package name. | | ||
| `package_version` | The package version. | |
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
This file contains bidirectional Unicode text that may be interpreted or compiled differently than what appears below. To review, open the file in an editor that reveals hidden Unicode characters.
Learn more about bidirectional Unicode characters
Original file line number | Diff line number | Diff line change |
---|---|---|
@@ -0,0 +1,134 @@ | ||
// Copyright 2023 The Gitea Authors. All rights reserved. | ||
// SPDX-License-Identifier: MIT | ||
|
||
package chef | ||
|
||
import ( | ||
"archive/tar" | ||
"compress/gzip" | ||
"io" | ||
"regexp" | ||
"strings" | ||
|
||
"code.gitea.io/gitea/modules/json" | ||
"code.gitea.io/gitea/modules/util" | ||
"code.gitea.io/gitea/modules/validation" | ||
) | ||
|
||
const ( | ||
KeyBits = 4096 | ||
SettingPublicPem = "chef.public_pem" | ||
) | ||
|
||
var ( | ||
ErrMissingMetadataFile = util.NewInvalidArgumentErrorf("metadata.json file is missing") | ||
ErrInvalidName = util.NewInvalidArgumentErrorf("package name is invalid") | ||
ErrInvalidVersion = util.NewInvalidArgumentErrorf("package version is invalid") | ||
|
||
namePattern = regexp.MustCompile(`\A\S+\z`) | ||
versionPattern = regexp.MustCompile(`\A\d+\.\d+(?:\.\d+)?\z`) | ||
) | ||
|
||
// Package represents a Chef package | ||
type Package struct { | ||
Name string | ||
Version string | ||
Metadata *Metadata | ||
} | ||
|
||
// Metadata represents the metadata of a Chef package | ||
type Metadata struct { | ||
Description string `json:"description,omitempty"` | ||
LongDescription string `json:"long_description,omitempty"` | ||
Author string `json:"author,omitempty"` | ||
License string `json:"license,omitempty"` | ||
RepositoryURL string `json:"repository_url,omitempty"` | ||
Dependencies map[string]string `json:"dependencies,omitempty"` | ||
} | ||
|
||
type chefMetadata struct { | ||
Name string `json:"name"` | ||
Description string `json:"description"` | ||
LongDescription string `json:"long_description"` | ||
Maintainer string `json:"maintainer"` | ||
MaintainerEmail string `json:"maintainer_email"` | ||
License string `json:"license"` | ||
Platforms map[string]string `json:"platforms"` | ||
Dependencies map[string]string `json:"dependencies"` | ||
Providing map[string]string `json:"providing"` | ||
Recipes map[string]string `json:"recipes"` | ||
Version string `json:"version"` | ||
SourceURL string `json:"source_url"` | ||
IssuesURL string `json:"issues_url"` | ||
Privacy bool `json:"privacy"` | ||
ChefVersions [][]string `json:"chef_versions"` | ||
Gems [][]string `json:"gems"` | ||
EagerLoadLibraries bool `json:"eager_load_libraries"` | ||
} | ||
|
||
// ParsePackage parses the Chef package file | ||
func ParsePackage(r io.Reader) (*Package, error) { | ||
gzr, err := gzip.NewReader(r) | ||
if err != nil { | ||
return nil, err | ||
} | ||
defer gzr.Close() | ||
|
||
tr := tar.NewReader(gzr) | ||
for { | ||
hd, err := tr.Next() | ||
if err == io.EOF { | ||
break | ||
} | ||
if err != nil { | ||
return nil, err | ||
} | ||
|
||
if hd.Typeflag != tar.TypeReg { | ||
continue | ||
} | ||
|
||
if strings.Count(hd.Name, "/") != 1 { | ||
continue | ||
} | ||
|
||
if hd.FileInfo().Name() == "metadata.json" { | ||
return ParseChefMetadata(tr) | ||
} | ||
} | ||
|
||
return nil, ErrMissingMetadataFile | ||
} | ||
|
||
// ParseChefMetadata parses a metadata.json file to retrieve the metadata of a Chef package | ||
func ParseChefMetadata(r io.Reader) (*Package, error) { | ||
var cm chefMetadata | ||
if err := json.NewDecoder(r).Decode(&cm); err != nil { | ||
return nil, err | ||
} | ||
|
||
if !namePattern.MatchString(cm.Name) { | ||
return nil, ErrInvalidName | ||
} | ||
|
||
if !versionPattern.MatchString(cm.Version) { | ||
return nil, ErrInvalidVersion | ||
} | ||
|
||
if !validation.IsValidURL(cm.SourceURL) { | ||
cm.SourceURL = "" | ||
} | ||
|
||
return &Package{ | ||
Name: cm.Name, | ||
Version: cm.Version, | ||
Metadata: &Metadata{ | ||
Description: cm.Description, | ||
LongDescription: cm.LongDescription, | ||
Author: cm.Maintainer, | ||
License: cm.License, | ||
RepositoryURL: cm.SourceURL, | ||
Dependencies: cm.Dependencies, | ||
}, | ||
}, nil | ||
} |
Oops, something went wrong.