You signed in with another tab or window. Reload to refresh your session.You signed out in another tab or window. Reload to refresh your session.You switched accounts on another tab or window. Reload to refresh your session.Dismiss alert
{{ message }}
This repository was archived by the owner on Sep 1, 2022. It is now read-only.
Go ahead and uncomment the conditional statement underneath where it says to
115
-
116
-
`Uncomment below for step 2`
117
-
118
-
119
-
### Run your code
120
-
121
-
To run your code, move inside your repo folder in your terminal and run `npm start`
122
-
123
-
### What your code should look like
124
-
125
-
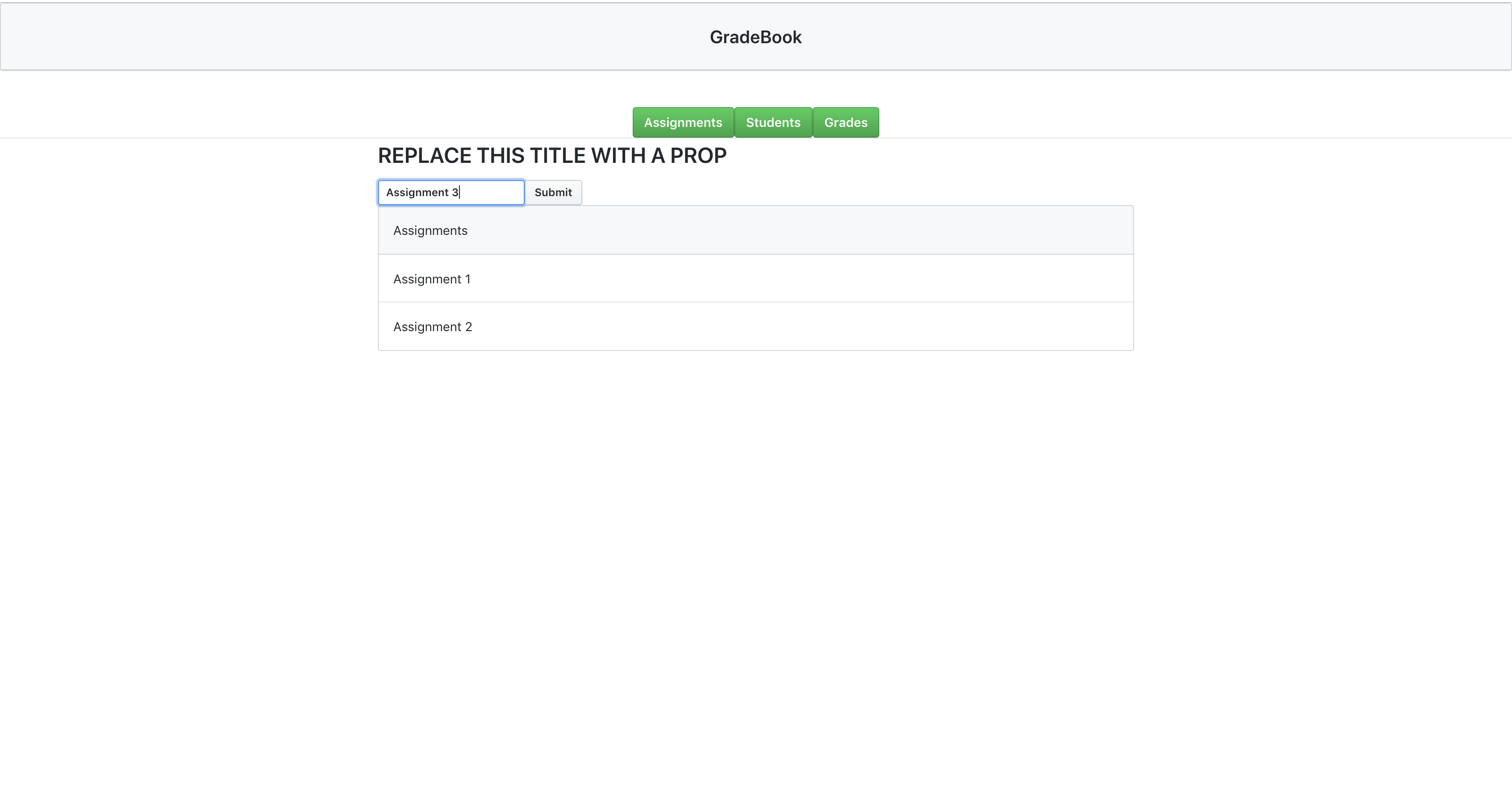
126
-
127
-
### Code Explanation
128
-
129
-
Let's take a look at what this code means. Basically, what it is saying is that when we click the `Assignments` button, the list for assignments will be assigned to the variable `tabChoice`. In our `render` method, we see that `tabChoice` is rendered.
130
-
131
-
But how do we specialize the `List` for our assignments? This where our props come into play. We create properties such as `placeholder`, and `currList`. We then assign values to them.
But what does the `List` component do with this information? Let's take a look.
146
-
147
-
Go to `List.jsx`. In the `render` method, in our input tag, you see that we set our `placeholder` to `this.props.placeholder`, and then at the bottom of our `render` method, we loop through out `this.props.currList`, to show each item in the list that we pass. Adding `this.props` tells the component to look for that property that was passed to it.
148
-
149
-
Let's go ahead and replace that ugly title with a prop. In our `render` method in `List.jsx`, where it says to replace the title with a prop, replace that with `{this.props.title}`.
150
-
151
-
### Run your code
152
-
153
-
To run your code, move inside your repo folder in your terminal and run `npm start`
154
-
155
-
### Push your code
156
-
Awesome! We are using props! You already learned so much!
157
-
158
-
To push your code to Github, run the following lines inside the repo folder in your terminal.
159
-
160
-
```
161
-
git add src/App.jsx
162
-
git add src/List.jsx
163
-
git commit -m "using props for List component"
164
-
git push origin changes
165
-
```
59
+
pullRequest: '%actions.metaPR2.data.number%'
60
+
- type: createPullRequestComment
61
+
body: replace-title-activity.md
62
+
file: src/List.jsx
166
63
position: 5
64
+
pullRequest: '%actions.metaPR2.data.number%'
167
65
description: Learn how to pass data to child components
In React, we store dynamic data in **state variables**. Let's take a look at how we store state variables. In `App.jsx`, take a look at the constructor. You'll see that we declare our state variables using `this.state = {.....}`.
204
-
205
-
206
-
Currently, in `App`, we have three state variables:
207
-
- `buttonClicked` - This stores which button was clicked. It is a state variable because the button that is clicked has the ability to change.
208
-
- `assignments` - This stores the list of assignments. It is a state variable because the list changes every time a new assignment is added.
209
-
- `grades` - This should store the grade for each student. However, we have no way to store students, so let's fix that!
210
-
211
-
Add a state variable to `App` and name it `students`. Set it equal to an empty list. Make sure to add the comma!
Ok, so now we added a state variable, how do we actually change the data?
225
-
226
-
Unfortunatley, we can't just do `this.state.studentsList = .....` to change data. I wish it was that simple!
227
-
228
-
To set the state of a state variable we have to use the method, `this.setState`.
229
-
230
-
Take a look at the above code in `App.jsx`
231
-
232
-
When this method is called, it setting the state variable `buttonClicked` to the argument, `buttonName` using `this.setState`.
233
-
234
-
In our `render` method we see that `handleButtonClicked` is called when each button is clicked with it's respective arguments. For instance, when the `Assignments` button is clicked, we call `handleButtonClicked("assignments")`.
Also, since we created a method that changes the a state variable, we have to bind it to our class, so that when we call it, we know what method to reference.
267
-
268
-
Go ahead and uncomment this line.
269
-
270
-
### Run your code
271
-
Run your code with `npm start` to make sure there are no errors.
272
-
273
-
274
-
### Push your code
275
-
276
-
```
277
-
git add src/App.jsx
278
-
git commit -m "using students state variable and setting state"
In React, we store dynamic data in **state variables**. Let's take a look at how we store state variables. In `App.jsx`, take a look at the constructor. You'll see that we declare our state variables using `this.state = {.....}`.
4
+
5
+
6
+
Currently, in `App`, we have three state variables:
7
+
-`buttonClicked` - This stores which button was clicked. It is a state variable because the button that is clicked has the ability to change.
8
+
-`assignments` - This stores the list of assignments. It is a state variable because the list changes every time a new assignment is added.
9
+
-`grades` - This should store the grade for each student. However, we have no way to store students, so let's fix that!
10
+
11
+
Add a state variable to `App` and name it `students`. Set it equal to an empty list. Make sure to add the comma!
Also, since we created a method that changes the a state variable, we have to bind it to our class, so that when we call it, we know what method to reference.
4
+
5
+
Go ahead and uncomment this line.
6
+
7
+
### Run your code
8
+
Run your code with `npm start` to make sure there are no errors.
9
+
10
+
11
+
### Push your code
12
+
13
+
```
14
+
git add src/App.jsx
15
+
git commit -m "using students state variable and setting state"
0 commit comments